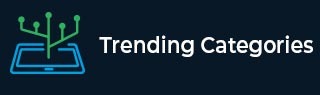
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

57 Views
The DoubleStream.generate() method returns an infinite sequential unordered stream where each element is generated by the provided DoubleSupplier.The syntax is as follows −static DoubleStream generate(DoubleSupplier s)Here, s is the DoubleSupplier for generated elements. The DoubleSupplier represents a supplier of double-valued results.To use the DoubleStream class in Java, import the following package −import java.util.stream.DoubleStream;The following is an example to generate Infinite stream of Double in Java with DoubleStream.generate() method −Exampleimport java.util.stream.*; import java.util.*; public class Demo { public static void main(String[] args) { Random r = new Random(); DoubleStream.generate(r::nextDouble).forEach(System.out::println); } }Here is ... Read More

9K+ Views
The range() method in the IntStream class in Java is used to return a sequential ordered IntStream from startInclusive to endExclusive by an incremental step of 1. This includes the startInclusive as well.The syntax is as follows −static IntStream range(int startInclusive, int endExclusive)Here, the parameter startInclusive includes the starting value, whereas endExclusive excludes the last valueTo work with the IntStream class in Java, import the following package −import java.util.stream.IntStream;Create an IntStream and add stream elements in a range using range() method. This returns a sequential ordered IntStream by an incremental step of 1 within the range −intStream.forEach(System.out::println);The following is an ... Read More

151 Views
The sum() method of the IntStream class is used in Java to return the sum of elements in this stream.The syntax is as follows −int sum()To work with the IntStream class in Java, import the following package −import java.util.stream.IntStream;Create IntStream and add some elements −IntStream intStream = IntStream.of(50, 100, 150, 200, 250, 300);Now, return the sum of elements in the IntStream added above −int sumVal = intStream.sum();The following is an example to implement IntStream sum() method in Java −Example Live Demoimport java.util.stream.IntStream; public class Demo { public static void main(String[] args) { IntStream intStream = IntStream.of(50, ... Read More

45 Views
An immutable copy of a Period where all the Period elements are negated can be obtained using the method negated() in the Period class in Java. This method requires no parameters and it returns the Period elements after negating them.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; import java.time.LocalDate; public class Demo { public static void main(String[] args) { String period = "P5Y7M15D"; Period p = Period.parse(period); System.out.println("The Period is: " + p); System.out.println("The Period with elements negated is: " + p.negated()); } ... Read More

3K+ Views
The Period between two dates can be obtained using the between() method in the Period class in Java. This method requires two parameters i.e. the start date and the end date and it returns the Period between these two dates.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; import java.time.LocalDate; public class Demo { public static void main(String[] args) { LocalDate startDate = LocalDate.parse("2015-03-15"); LocalDate endDate = LocalDate.parse("2019-05-20"); System.out.println("The start date is: " + startDate); System.out.println("The end date is: " + endDate); ... Read More

47 Views
An immutable copy of a Period where all the Period elements are multiplied by a value can be obtained using the method multipliedBy() in the Period class in Java. This method requires a single parameter i.e. the value which is to be multiplied and it returns the immutable copy of the Period which is multiplied by a value.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { String period = "P5Y9M4D"; Period p = Period.parse(period); System.out.println("The Period is: " ... Read More

63 Views
An instance of a Period object can be obtained from a Temporal object using the from() method in the Period class in Java. This method requires a single parameter i.e. the TemporalAmount and it returns the Period object that is obtained.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { int days = 20; int months = 11; int years = 3; Period p = Period.from(Period.of(years, months, days)); System.out.println("The Period is: " + ... Read More

484 Views
The Period instance can be obtained from a string value using the parse() method in the Period class in Java. This method requires a single parameter i.e. the string which is to be parsed. This string cannot be null. Also, it returns the Period instance obtained from the string value that was passed as a parameter.A program that demonstrates this is given as follows:Example Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { String period = "P5Y7M15D"; Period p = Period.parse(period); System.out.println("The Period is: " + p); ... Read More

65 Views
The Period can be obtained with the given number of days, months and years using the of() method in the Period class in Java. This method requires a 3 parameters i.e. the number of days, the number of months and the number of years. Also, it returns the Period object with the given number of days, months and years.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { int days = 20; int months = 11; int years = ... Read More