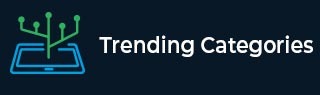
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

235 Views
The containsAll() method checks for all the elements in the specified collection. It returns TRUE if this collection has all the elements. The methods check for each element one by one to see if it's contained in this collection.The syntax is as follows −public boolean containsAll(Collection c)To work with AbstractCollection class in Java, import the following package −import java.util.AbstractCollection;The following is an example to implement AbstractCollection containsAll() method in Java −Example Live Demoimport java.util.ArrayList; import java.util.AbstractCollection; public class Demo { public static void main(String[] args) { AbstractCollection absCollection1 = new ArrayList(); absCollection1.add("These"); ... Read More

69 Views
To fetch the value from an Octet Tuple, use the getValueX() method. Here X is the index for which you want the value.For example, to fetch the 5th element i.e. 4th index, use the getValueX() method as −getValue(4);Let us first see what we need to work with JavaTuples. To work with Octet class in JavaTuples, you need to import the following package −import org.javatuples.Octet;Note − Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. ... Read More

77 Views
Create Octel Tuple from List collection as well using the fromCollection() method in Java. Let us first see what we need to work with JavaTuples. To work with Octet class in JavaTuples, you need to import the following package −import org.javatuples.Octet;Note − Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. Refer the below guide for all the steps to run JavaTuples −Steps − How to run JavaTuples program in EclipseThe following is an ... Read More

80 Views
To add elements to the AbstractCollection class, use the add() method. For example −absCollection.add("These"); absCollection.add("are"); absCollection.add("demo");To work with AbstractCollection class in Java, import the following package −import java.util.AbstractCollection;The following is an example to add elements to AbstractCollection class in Java −Example Live Demoimport java.util.ArrayList; import java.util.AbstractCollection; public class Demo { public static void main(String[] args) { AbstractCollection absCollection = new ArrayList(); absCollection.add("These"); absCollection.add("are"); absCollection.add("demo"); absCollection.add("elements"); System.out.println("Displaying elements in the AbstractCollection: " + absCollection); } }OutputDisplaying elements in the AbstractCollection: [These, ... Read More

56 Views
The flatMap() method in LongStream class returns a stream consisting of the results of replacing each element of this stream with the contents of a mapped stream produced by applying the provided mapping function to each element.The syntax is as follows −LongStream flatMap(LongFunction

60 Views
The forEachOrdered() method in Java performs an action for each element of this stream, guaranteeing that each element is processed in encounter order for streams that have a defined encounter order.The syntax is as follows −void forEachOrdered(LongConsumer action)Here, parameter wrapper is a non-interfering action to perform on the elements. LongConsumer represents an operation that accepts a single long-valued argument and returns no result.To use the LongStream class in Java, import the following package −import java.util.stream.LongStream;The following is an example to implement LongStream forEachOrdered() method in Java −Example Live Demoimport java.util.*; import java.util.stream.LongStream; public class Demo { public static void ... Read More

198 Views
The concat() method in the Java IntStream class forms a concatenated stream. The elements of this stream are all the elements of the first stream followed by all the elements of the second stream.The syntax is as follows −static IntStream concat(IntStream one, IntStream two)Here, the parameter one is the first stream, whereas two is the second stream. The method returns the concatenated result of the stream one and two.Let us create two IntStream and add some elements −IntStream intStream1 = IntStream.of(10, 20, 30, 40, 50); IntStream intStream2 = IntStream.of(60, 70, 80, 90);Now, to concate both the streams, use the concat() ... Read More

69 Views
The equals() method is inherited from the AbstractList class in Java. It is used to check the object for equality with this list. It returns TRUE if the object is equal to this list, else FALSE is returned.The syntax is as follows −public boolean equals(Object o)Here, o is the object to be compared for equality with this list.To work with the AbstractSequentialList class in Java, you need to import the following packageimport java.util.AbstractSequentialList;The following is an example to implement AbstractSequentialList equals() method in JavaExample Live Demoimport java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { public static void main(String[] args) { ... Read More

80 Views
The subList() method returns a part of this list between the specified fromIndex, inclusive, and toIndex, exclusive. Get a sublist using the method by setting the range as the two parameters.The syntax is as follows −public List subList(int fromIndex, int toIndex)Here, the parameter fromIndex is the low endpoint (inclusive) of the subList, whereas toIndex is the high endpoint (exclusive) of the subListTo work with the AbstractSequentialList class in Java, you need to import the following package −import java.util.AbstractSequentialList;The following is an example to implement AbstractSequentialList subList() method in Java −Example Live Demoimport java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { ... Read More

70 Views
The set() method of the AbtstractSequentialList class is used to replace the element at the specified position in this list with the specified element. It returns the element which is replaced. The syntax is as follows −E set(int index, E element)Here, the index is the index of the element to replace. The ele is the element to be stored at the specified position.To work with the AbstractSequentialList class in Java, you need to import the following package −import java.util.AbstractSequentialList;The following is an example to implement AbstractSequentialList set() method in Java −Example Live Demoimport java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { ... Read More