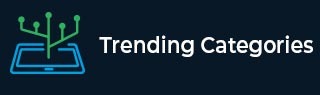
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

57 Views
An immutable copy of a duration where the required duration is multiplied by a value can be obtained using the method multipliedBy() in the Duration class in Java. This method requires a single parameter i.e. the value which is to be multiplied and it returns the immutable copy of the duration which is multiplied by a value.A program that demonstrates this is given as follows −Example Live Demoimport java.time.Duration; import java.time.temporal.*; public class Demo { public static void main(String[] args) { Duration d = Duration.ofHours(7); System.out.println("The duration is: " + d); ... Read More

54 Views
An immutable copy of a duration where the required duration is divided by a value can be obtained using the method dividedBy() in the Duration class in Java. This method requires a single parameter i.e. the value which is to be divided and it returns the immutable copy of the duration which is divided by a value.A program that demonstrates this is given as follows −Example Live Demoimport java.time.Duration; import java.time.temporal.*; public class Demo { public static void main(String[] args) { Duration d = Duration.ofHours(15); System.out.println("The duration is: " + d); ... Read More

136 Views
An immutable copy of a duration where the required duration is added to it can be obtained using the plus() method in the Duration class in Java. This method requires two parameters i.e. the duration to be added and the TemporalUnit of the duration. Also, it returns the duration with the required duration added to it.A program that demonstrates this is given as follows −Example Live Demoimport java.time.Duration; import java.time.temporal.*; public class Demo { public static void main(String[] args) { Duration d = Duration.ofHours(10); System.out.println("The duration is: " + d); System.out.println("Duration ... Read More

182 Views
An immutable copy of a duration where the required duration is subtracted from it can be obtained using the minus() method in the Duration class in Java. This method requires two parameters i.e. the duration to be subtracted and the TemporalUnit of the duration. Also, it returns the duration with the required duration subtracted from it.A program that demonstrates this is given as follows −Example Live Demoimport java.time.Duration; import java.time.temporal.*; public class Demo { public static void main(String[] args) { Duration d = Duration.ofHours(10); System.out.println("The duration is: " + d); System.out.println("Duration ... Read More

4K+ Views
The difference between two LocalTime objects can be obtained using the until() method in the LocalTime class in Java. This method requires two parameters i.e. the end time for the LocalTime object and the Temporal unit. Also, it returns the difference between two LocalTime objects in the Temporal unit specified.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.*; public class Demo { public static void main(String[] args) { LocalTime lt1 = LocalTime.parse("10:15:30"); LocalTime lt2 = LocalTime.parse("12:21:30"); System.out.println("The first LocalTime is: " + lt1); ... Read More

80 Views
The range of values for a ChronoField can be obtained using the range() method in the LocalTime class in Java. This method requires a single parameter i.e. the ChronoField for which the range of values is required and it returns the range of values.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.ChronoField; import java.time.temporal.ValueRange; public class Main { public static void main(String[] args) { LocalTime lt = LocalTime.parse("11:19:50"); System.out.println("The LocalTime is: " + lt); ValueRange range = lt.range(ChronoField.MICRO_OF_SECOND); System.out.println("The range of MICRO_OF_SECOND ... Read More

47 Views
An immutable copy of a LocalDateTime with the day of month altered as required is done using the method withDayOfMonth() in the LocalDateTime class in Java. This method requires a single parameter i.e. the day of month that is to be set in the LocalDateTime and it returns the LocalDateTime with the day of month altered as required.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Main { public static void main(String[] args) { LocalDateTime ldt1 = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt1); LocalDateTime ... Read More

83 Views
The findAny() method of the IntStream class in Java is used to return an OptionalInt describing some element of the stream, or an empty OptionalInt if the stream is empty.The syntax is as follows −OptionalInt findAny()Here, OptionalInt is a container object which may or may not contain an int value.Create an IntStream and add some elements −IntStream intStream = IntStream.of(20, 35, 50, 60, 80, 100);Now, return any of the element of the stream using findAny() in Java −OptionalInt res = intStream.findAny();The following is an example to implement IntStream findAny() method in Java −Example Live Demoimport java.util.*; import java.util.stream.IntStream; public class ... Read More

92 Views
The iterator() method of the AbstractCollection class in Java is used to return an iterator over the elements contained in this collection.The syntax is as follows −public abstract Iterator iterator()To work with AbstractCollection class in Java, import the following package −import java.util.AbstractCollection;For Iterator, import the following package −import java.util.Iterator;The following is an example to implement the AbstractCollection iterator() method in Java −Example Live Demoimport java.util.Iterator; import java.util.ArrayList; import java.util.AbstractCollection; public class Demo { public static void main(String[] args) { AbstractCollection absCollection = new ArrayList(); absCollection.add("Laptop"); absCollection.add("Tablet"); absCollection.add("Mobile"); ... Read More

236 Views
The isEmpty() method of the AbstractCollection class checks whether the collection is empty or not i.e. whether it has zero elements. It returns if the Collectionn has no elements.The syntax is as follows −public boolean isEmpty()To work with AbstractCollection class in Java, import the following package −import java.util.AbstractCollection;The following is an example to implement AbstractCollection isEmpty() method in Java −Example Live Demoimport java.util.ArrayList; import java.util.AbstractCollection; public class Demo { public static void main(String[] args) { AbstractCollection absCollection = new ArrayList(); absCollection.add("Laptop"); absCollection.add("Tablet"); absCollection.add("Mobile"); absCollection.add("E-Book ... Read More