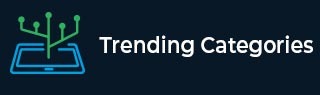
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
In our day to day life, there is a huge data being generated in this real world. Among the information generated, Dates are generally used for various things such as scheduling an appointment, planning for day-to-day activities. While working with dates in programming, extracting the year from a specific date can be a common task. We can extract the Year from a particular Date using different in-built functions in Java programming language. In this article we will be discussing different approaches for extracting year from a particular date. Example Input: "13-07-2000" Output: 2000 Example Input: "1997/07/13" Output: 1997 ... Read More

442 Views
A group of files combined stored at a common location is known as “Directory”. A directory not only contains a group of files but also it can contain a group of other directories as well. A directory is also known as a “Folder”. Directories are a fundamental component of file systems and are used by different operating systems to provide a logical organization of files and to helps us to perform file management tasks such as searching, sorting, and moving files. In this section, we are going to write a java program to get the size of a directory. What ... Read More

5K+ Views
Introduction In Java, a list is an ordered collection of elements that allows duplicates. Sometimes, we may need to combine two lists into one list. Concatenating two lists means joining the elements of the two lists to form a new list containing all the elements of both lists. There are various ways to concatenate two lists in Java, including using loops, streams, and built-in methods. In this context, we will explore different approaches to concatenate two lists in Java. One approach is to use the addAll() method provided by the List interface. This method adds all the elements of one ... Read More

458 Views
Introduction The Java program to compute the sum of numbers in a list using a while-loop is a simple program that takes a list of integers and computes their sum using a while-loop construct. In this program, an ArrayList of integers is created, and a few numbers are added to the list. The program then uses a while-loop to iterate over each element in the list, adding each element to a variable "sum", which keeps track of the running sum of the numbers. Once the loop has finished, the final value of "sum" is printed to the console, which is ... Read More

2K+ Views
Introduction Java is a popular programming language that is used for developing a wide range of applications, including those that involve working with lists of numbers. One common task is to compute the sum of numbers in a list, which can be accomplished using a for-loop. In this approach, we first create a list of numbers, then initialize a variable to hold the sum. We then use a for-loop to iterate over each element in the list, adding it to the sum variable. After the loop has completed, the sum variable contains the total sum of all the numbers in ... Read More

588 Views
Introduction The Java program to compute the running total of a list is a simple program that demonstrates the use of loops and lists in Java. The program takes a list of integers as input and computes the running total of the numbers in the list, which is the cumulative sum of all the numbers up to a particular point. The program initializes a variable called runningTotal to 0 and then loops through the list of numbers using a for loop. For each number in the list, the program adds it to the running total variable and prints out the ... Read More

164 Views
Introduction The Java program to compute the area of a triangle using determinants is a concise and efficient program that calculates the area of a triangle given the coordinates of its three vertices. This program is useful for anyone studying or working with geometry, as it demonstrates how to use basic arithmetic and algebraic calculations in Java, and how to read in user input using the Scanner class. The program prompts the user to enter the coordinates of three points of the triangle, which are then read in and used to calculate the determinant of the matrix of coordinates. The ... Read More

342 Views
Introduction In Java, objects can be compared using the equals() method, which checks whether two objects are equal based on their properties. When comparing objects in Java, it is important to override the equals() method in the class to ensure that the comparison is done based on the desired properties. This Java program compares two objects of type Person by overriding the equals() method to compare the objects based on their name and age properties. The equals() method is first used to check if the objects are of the same class and then compares the name and age properties. If ... Read More

893 Views
Introduction The Java program to combine two lists by alternatively taking elements is a simple code snippet that takes in two lists of any type of object that can be stored in a list and returns a new list that contains elements from both input lists alternately. The program defines a method called alternateMerge that takes two lists as input and returns a new list that contains elements from both lists alternately. It determines the size of both lists and loops through the larger of the two lists. For each index in the loop, it adds the element from the ... Read More

222 Views
Introduction In Java, we can define variables and methods as static. A static variable or method belongs to the class itself rather than to the individual objects of the class. Therefore, we can access a static variable or method using the class name, without creating an object of the class. In this program, we will explore how to check the accessibility of a static variable by a static method. We will define a class with a static variable and a static method that accesses the variable. We will then call the static method to check if it can access the ... Read More