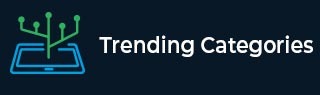
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

237 Views
Suppose you have a sorted array with no duplicate values and from a certain index this array is rotated. You have to search for a particular element in it. If it is available in the array then return the index otherwise return -1. In this article, we will solve the given problem using two approaches Linear search and Binary search. Approach 1: Using Linear Search This approach will search for the given element in sequential manner. Algorithm Step 1 − First, declare and initialize an array named ‘araylist’ and an integer variable named ‘searchElem’ that we are going ... Read More

417 Views
There are numerous ways of joining strings in java. The StringJoiner class and String.join() method are two of them. Both are used to join two or any number of strings but the difference lies in their implementation. In this article, we will try to find the differences that exist in the usage of StringJoiner class and String.join() method. StringJoiner Class It is a class available in java.util package and it is added from JDK 8. We can join multiple strings at a time. Before using, it is necessary to import it into our program using the following command ... Read More

2K+ Views
Scanner is a class available in ‘java.util’ package used to take input from numerous sources like a file, or console. The most common source of taking input is from standard input i.e. keyboard. The scanner class has few methods to take input of primitives and strings from the keyboard. We will understand scanner class and its various methods through this article. Scanner and nextChar() The following methods of Scanner class is used to take input − nextInt() − To take integer as an input. nextLong() − To take long as an input. nextDouble() − To take double as an ... Read More

705 Views
String and Arrays are two distinct things. Array is a linear data structure that is used to store group of elements with similar datatypes but a string is a class in Java that stores a series of characters. Those characters are actually String-type objects. Since strings are objects we can say that string arrays are group of string-type objects. In this article, we will understand string arrays in java and perform some operations on them. String Arrays The string array is a combination of array and string both. Therefore, it has the properties of both array and string such ... Read More

154 Views
A string is a class in Java that stores a series of characters. Those characters are actually String-type objects. The value of string is enclosed within double quotes. The string class is available in java.lang package. In this article, we will look into the storage mechanism of strings in java. String and Storage of String To create strings − Syntax String nameOfobject = “ values ”; Instance 1 String st1 = “Tutorix and Tutorialspoint”; Here, ‘st1’ is the reference variable and its values are in double quotes. We can also use new keyword to create ... Read More

2K+ Views
A linked list is a linear data structure that consists of a sequence of elements called nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. The importance of linked lists lies in their flexibility, as nodes can be inserted or removed at any position in the list, unlike arrays which require elements to be shifted over to make space. Linked lists are also dynamic, meaning they can grow or shrink as needed, unlike fixed-size arrays. Finally, linked lists can be used to implement more complex data structures such ... Read More

267 Views
A circle is a closed shape formed by tracing a point that moves in a plane such that its distance from a given point is constant. A line is a straight one-dimensional figure that does not have a thickness, and it extends endlessly in both directions. In this article we will check if a given line touches a circle or intersects it using java. We will be given with the centre coordinates and radius of a circle along with equation of the line. We need to check if the given line collides with the circle or not and hence three ... Read More

113 Views
A polygon is a 2-dimensional closed shape that has at least 3 sides. Depending on the number of sides, relationships of the sides and angles, and other characteristics, polygons can be classified under different names like triangles, squares, and quadrilaterals. The convex polygon definition explains that one is a polygon whose angles are all less than 180 degrees each. That also means that the vertices, the point where two sides meet, all point outward from the centre of the shape. In this article, we will find if two convex regular polygons have same centre or not. We will take two ... Read More

576 Views
A square is a two-dimensional shape which has four sides of equal length. The opposite sides of a square are parallel to each other and all four interior angles are right angles with diagonal of equal length. In this article we will check how to confirm if given four points form a square. We will be given a square with four points i.e. A, B, C, D as shown in the figure − We need to check from these points that if they form a square or not. To check this it should satisfy the following condition − ... Read More

317 Views
In Java, Array is an object. It is a non-primitive data type which stores values of similar data type. The matrix in java is nothing but a multi-dimensional array which represents multiple rows and columns. Here we have given a matrix which contains set of elements including both positive and negative numbers and as per the problem statement we have to replace negative numbers with 0 and positive numbers with 1. Let’s deep dive into this article, to know how it can be done by using Java programming language. To show you some instances Instance-1 Given matrix =-21 22 -23 24 -25 26 ... Read More