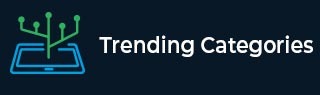
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

413 Views
The Internet Protocol suite contains all sort of protocols that enables communication between devices over the Internet. UDP is one of the protocols of this suite and its full form is User Datagram Protocol. Unlike TCP, it is not reliable and also it is a connectionless protocol. It does not establish any kind of connection to other devices before sending the data. In this article, we will develop a simple client-server side calculator using UDP in Java. The client will request the operation and the server will send the result to client device after calculating it. Java Networking ... Read More

610 Views
The Internet Protocol suite contains all sort of protocols that enables communication between devices over the Internet. TCP is the most common protocol of this suite. It is a connection-oriented protocol, which means it maintains the established connection between two devices till the end of a communication. This is the reason it is used while web surfing, sending emails and transferring files. In this article, we will develop a simple client-server side calculator using TCP in Java. The client will request the operation and the server will send the result to client device after calculating it. Java Networking Let’s ... Read More

619 Views
There are two types of collections in Java. One is ordered and the other one is unordered collection. The ordered collections store their elements in the order in which they are inserted i.e. it maintains the insertion order of elements. Whereas, the unordered collections such as Map and Set do not maintain any order. In this article, we will create an unordered collection and try to shuffle its elements using the inbuilt method ‘Collections.shuffle()’. Program to shuffle elements of Unordered Collection Set SortedSet Interface This interface has the term ‘Sorted’ in its name which signifies that it contains all ... Read More

535 Views
In Java, downcasting is the process of converting an object of parent class to object of child class. We need to perform the conversion explicitly. It is quite similar to what we do in primitive typecasting. In this article, we will learn about downcasting and what are the rules that we must follow to downcast an object in Java. Object Downcasting in Java Earlier we discussed that downcasting is somewhat similar to typecasting. However, there exist a few differences too. The first is that we can type cast only primitive datatypes and the second is that it is irreversible action ... Read More

103 Views
Vectors implement the List interface and are used to create dynamic arrays. The array whose size is not fixed and can grow as per our needs is called as a dynamic array. The vectors are very similar to ArrayList in terms of use and features. In this article, we will learn how we can create a vector and search for a particular element by its index in Java. Let’s discuss Vector first. Vector Although vector is similar to ArrayList in many ways, there exist some differences too. The Vector class is synchronized and several legacy methods are contained by it. ... Read More

158 Views
Array is a linear data structure that is used to store group of elements with similar datatypes. It stores data in a sequential manner. Once we create an array we can’t change its size i.e. it is of fixed length. In this article, we will create an array of triplet and try to sort them using the Comparable and Comparator interfaces. The term array of triplet means an array having three elements. Program to sort Array of Triplet using Comparator Comparator As the name suggests it is used to compare something. In Java, the Comparator is an interface that is ... Read More

305 Views
Vectors implement the List interface and are used to create dynamic arrays. The array whose size is not fixed and can grow as per our needs is called as a dynamic array. The Comparator is an interface available in ‘java.util’ package. Sorting means rearranging the elements of a given list or array in ascending or descending order. In this article, we will create a vector and then try to sort its elements in descending order using a comparator. Program to Sort Java Vector in Descending Order Comparator As the name suggests it is used to compare something. In ... Read More

524 Views
Runtime Type Identification in short RTTI is a feature that enables retrieval of the type of an object during run time. It is very crucial for polymorphism as in this oops functionality we have to determine which method will get executed. We can also implement it for primitive datatypes like Integers, Doubles and other datatypes. In this article, we will explain the use case of Runtime Type Identification in Java with the help of examples. Program for Runtime Type Identification Let’s discuss a few methods that can help us to identify type of object: instanceOf It is a ... Read More

257 Views
LinkedHashMap is a generic class that is used to implement Map Interface. Also, it is a sub class of the HashMap class therefore, it can use all the methods and also perform similar operations that a HashMap class is capable of. Java provides various ways to sort LinkedHashMap, we will learn how to create and sort it by its values using Comparable Interface through this article. Program to sort LinkedHashMap by Values Before jumping to the sorting program directly, let’s take a look at few concepts first − LinkedHashMap As we have discussed earlier the LinkedHashMap class extends ... Read More

695 Views
LinkedHashMap is a generic class that is used to implement Map Interface. Also, it is a sub class of the HashMap class therefore, it can use all the methods and also perform similar operations that a HashMap class is capable of. Java provides various ways to sort LinkedHashMap, we will learn how to create and sort it by using its keys through this article. Program to sort LinkedHashMap by Keys Before jumping to the sorting program directly, let’s take a look at few concepts first − LinkedHashMap As we have discussed earlier the LinkedHashMap class extends HashMap class ... Read More