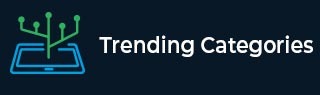
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

197 Views
Introduction Java concurrency provides several classes and tools that allow developers to create multi-threaded applications. Among these are the ThreadPoolExecutor and Semaphore classes. The former is used to manage a pool of worker threads, while the latter can limit the number of threads accessing a certain resource at a given time. This article delves into throttling the task submission rate using these two Java classes. By understanding how to effectively manage threads and control their execution, you can significantly optimize your Java applications. Understanding ThreadPoolExecutor and Semaphore Before we jump into how to throttle the task submission rate, it's essential ... Read More

408 Views
Are you embarking on a journey into the realm of multi-threaded programming in Java? Are you finding yourself tangled in the web of classes Java offers for generating random numbers, such as ThreadLocalRandom and SecureRandom? Fear not! This article will break down the differences, similarities, and suitable use-cases for these two classes, ensuring you choose the right tool for your needs. Understanding ThreadLocalRandom in Java Java's ThreadLocalRandom class was introduced in Java 7 to handle the generation of random numbers in a multi-threaded environment more efficiently. The class is part of the java.util.concurrent package and is essentially a stripped-down version ... Read More

638 Views
Introduction The ThreadFactory interface in Java is a vital component of multithreaded programming. This powerful interface creates a new thread on demand and can be customized to suit different requirements. This article aims to provide a comprehensive understanding of this interface, along with several practical examples. By the end of this piece, you'll have a firm grasp on the ThreadFactory interface and its usage in Java programming. Understanding Java ThreadFactory Interface Before delving into examples, let's start by understanding the basic concepts. What is the ThreadFactory Interface? The ThreadFactory interface is part of Java's java.util.concurrent package. It's designed to create ... Read More

792 Views
In the world of multithreaded programming, managing concurrent access to shared data is a considerable challenge. An essential aspect of this challenge is achieving thread safety. This article explores the concept of thread safety in Java and provides comprehensive guidance on how to ensure your Java code is thread-safe. Understanding Thread Safety Thread safety refers to the property of an object that guarantees safe execution by multiple threads concurrently, without causing any problems such as data inconsistencies or race conditions. When a piece of code is thread-safe, it functions correctly even when accessed by multiple threads simultaneously. A code segment ... Read More

225 Views
Java's multithreading capabilities can significantly enhance an application's performance and responsiveness. However, when multiple threads share and manipulate the same data, developers can face complex issues, notably thread interference and memory consistency errors. This article takes an in-depth look at these concepts and offers solutions to mitigate such challenges in your Java applications. Thread Interference in Java: The Race Condition Thread interference, also known as race condition, is a common issue in multithreaded environments. It occurs when two or more threads access shared data simultaneously, leading to unreliable and unexpected results. Suppose we have two threads that both increment the ... Read More

378 Views
In the world of Java web development, understanding the HttpSession interface is key to creating dynamic and responsive web applications. In this article, we will explore what the HttpSession interface is, how it works, and why it plays a crucial role in the Servlet specification. What is the HttpSession Interface? At its core, the HttpSession interface is a fundamental component of the Java Servlet API, which enables web developers to track a user's session across multiple HTTP requests. When a user first visits a web application, a unique session is created to represent their interaction. This session allows the application ... Read More

808 Views
Java, as a statically-typed language, places a heavy emphasis on compile-time checks and warnings. These alerts are crucial in catching potential problems before the program is run. However, in some scenarios, certain warnings may be deemed unnecessary or may not apply to a specific situation. This is where the @SuppressWarnings annotation comes in. This article dives into the @SuppressWarnings annotation in Java, explaining its purpose, usage, and implications for your Java code. What is the @SuppressWarnings Annotation? The @SuppressWarnings annotation belongs to the java.lang package and is used to instruct the compiler to suppress specific warnings for the annotated part ... Read More

201 Views
The Java programming language, like many others, is continually evolving. As new features are introduced and improvements are made, certain elements become less relevant or efficient, and alternatives are recommended. The @Deprecated annotation is a tool Java developers use to indicate that a class, method, or field is outdated and there is a better alternative. In this article, we'll explore the @Deprecated annotation in detail, discussing its purpose, usage, and implications for your Java code. Understanding the @Deprecated Annotation The @Deprecated annotation is a marker annotation (meaning it doesn't contain any elements) included in the java.lang package. When applied to ... Read More

290 Views
Introduction Spring Security is a highly customizable authentication and access-control framework for Java applications, particularly for Spring-based applications. Testing these security measures is crucial to ensure a secure application. In this article, we'll explore how to effectively test Spring Security with JUnit, a leading unit testing framework in Java. Understanding Spring Security and JUnit Spring Security is a powerful framework that provides authentication, authorization, and other security features for enterprise-grade applications. It's comprehensive yet flexible, making it suitable for a variety of security requirements. JUnit is a simple, open-source framework used to write repeatable tests in Java. It provides annotations ... Read More

390 Views
Test-Driven Development (TDD) is a software development approach where tests are written before the actual code. TDD has gained substantial traction due to its emphasis on code quality and maintainability. This article explores how TDD can be effectively implemented using JUnit5 and Mockito, two powerful frameworks in the Java ecosystem. What is Test-Driven Development? Test-Driven Development is an iterative development process where developers first write a test case for a new function or feature, then write the minimum amount of code to pass that test, and finally refactor the code for optimization. This approach enhances the design, reduces bugs, and ... Read More