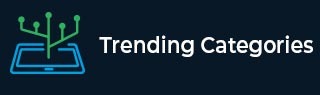
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming
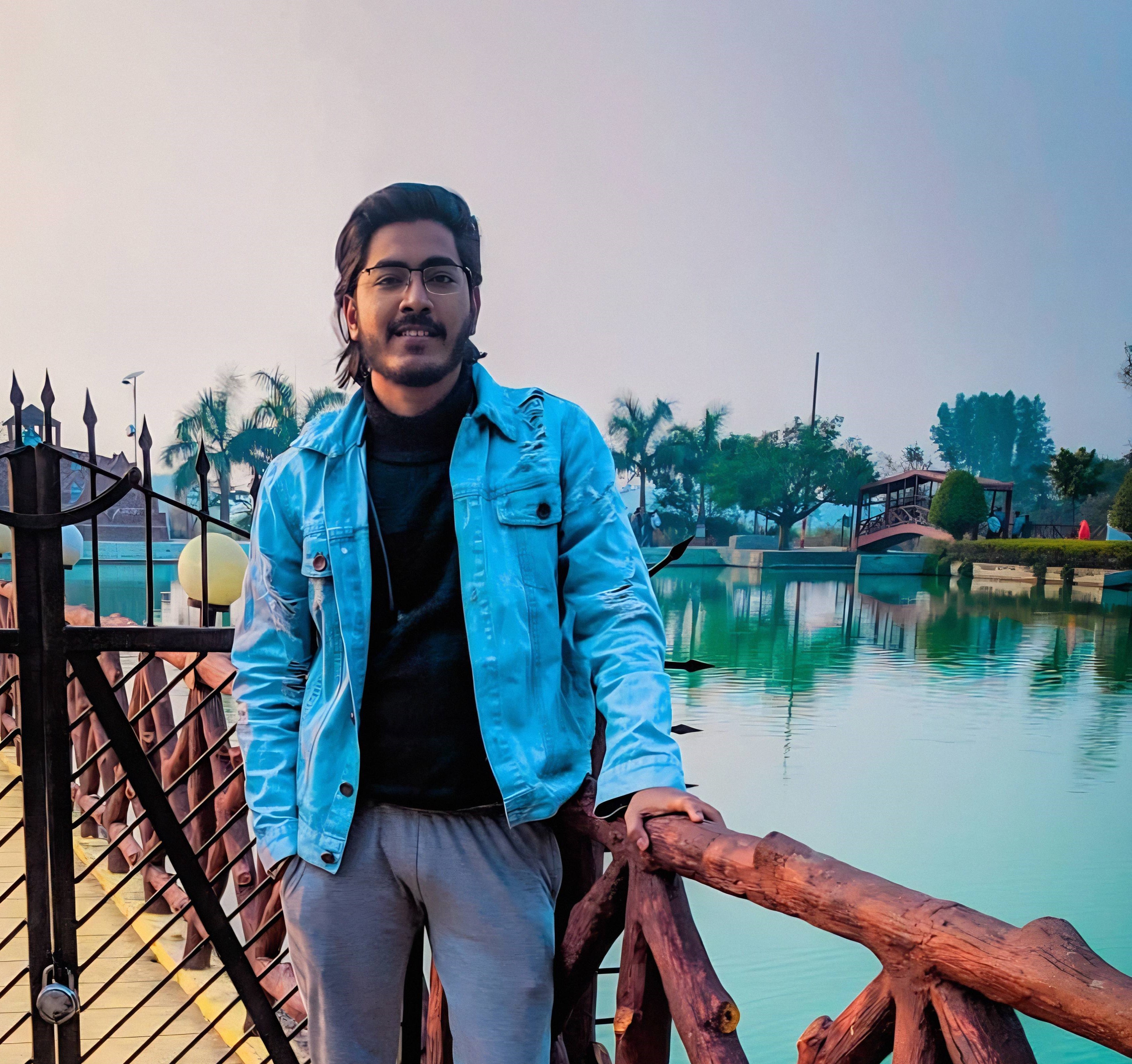
873 Views
Dataclass, NamedTuple, and Object are used to create structured datatypes in Python. Though all three are used to create structured data they differ in their properties and implementation method. In this article, we will understand the difference between DataClass, NamedTuple, and Object in Python. Feature Object NamedTuple Dataclass Creation Objects are created by defining a class and its attributes and methods manually. Named tuples are created using the named tuple function from the collections module. The field names and values are specified manually. Data classes are created using the @dataclass decorator. The class attributes ... Read More

154 Views
A feedback edge set in a graph refers to a set of edges that, when removed from the graph, eliminates all cycles or feedback loops. In other words, it is a subset of edges that, when deleted, transforms the original graph into a directed acyclic graph (DAG). A good feedback edge set is a feedback edge set that has the minimum possible number of edges. In this tutorial, we will learn to find a Good Feedback Edge Set in a Graph. Problem Statement Write a Java program that identifies and removes feedback edges in a graph to construct a Good ... Read More

9K+ Views
In Java programming, there are scenarios where we need to extract individual digits from an integer for further manipulation or analysis. This tutorial will guide you through the process of extracting digits from a given integer using Java. Syntax while (num > 0) { int digit = num % 10; System.out.println(digit); num = num / 10; } The above is the syntax to extract digits from an integer in Java. We keep extracting the last digit by taking the remainder of the number with 10. We divide the number by 10 until it ... Read More

116 Views
The Zhu-Takaoka algorithm is one of the best algorithms for pattern matching. It is developed using the combination of the Boyer-Moore and KMP string-matching algorithms. The Zhu-Takaoka algorithm utilizes the good character shift and bad character shift techniques to solve the problem. Problem statement − We have given two strings. We need to implement the Zhu-Takaoka algorithm for pattern matching. Sample Examples Input str = "PQRDPQRSSE"; patt = "PQRS"; Output 5 Explanation The ‘PQRS’ pattern exists at position 5. So, it prints 5. Input str = "PQRDPQRSSE"; patt = "PRQS"; Output -1 Explanation ... Read More

151 Views
The naïve approach to finding the prime numbers in the given range is to check whether each number is prime. Also, we need to make iterations equal to the given number to check whether the number is prime. So, the naïve approach is very time-consuming, and we need to optimize it to make it time efficient. In this tutorial, we will learn wheel factorization and Sieve of Eratosthenes algorithms given by Sieve to find the prime numbers in the given range efficiently. Problem statement − We have given left and right integer values. We need to implement the Wheel factorization ... Read More

361 Views
In this problem, we will find all palindromic substrings of the given length. There are two ways to solve the problem. The first way is to compare the characters of substring from start to last, and another way is to reverse the substring and compare it with the original substring to check whether the substring is palindrome. Problem statement − We have given a string alpha. We need to find all palindromic substrings of the given string. Sample Examples Input alpha = "abcd" Output 4 Explanation The palindromic substrings are ‘a’, ‘b’, ‘c’, and ‘d’. Input alpha ... Read More

326 Views
In this problem, we will find two array elements such that the difference between them is maximum. We can make a pair of each element and find the difference between the elements of each pair. After that, we can take a pair whose element has a maximum difference. Another approach is to sort the array and take the largest and smallest element from the array. Problem statement − We have given an array containing the integer values. We need to find two array elements to maximize the difference between them. Sample Examples Input array[] = { 50, 10, 30, ... Read More

2K+ Views
A well-liked programming paradigm called object-oriented programming (OOP) emphasizes the use of objects to represent and manipulate data. The ability to construct methods that can interact with these objects is one of the main characteristics of OOP. Class methods, static methods, and instance methods are the three different categories of methods in Python. Methods that belong to a class rather than an instance of that class include class methods and static methods. Class methods receive a reference to the class or instance as their first argument, but static methods do not. This is the primary distinction between them. ... Read More

206 Views
Understanding and effectively utilizing Java's functional interfaces is an essential skill for any modern Java developer. Among these interfaces, the ToDoubleFunction interface is a significant tool that offers tremendous utility. This article aims to provide a comprehensive exploration of the ToDoubleFunction interface in Java, enriched with practical examples to enhance your understanding. What is the ToDoubleFunction Interface? Introduced in Java 8, the ToDoubleFunction interface is a part of the java.util.function package. It represents a function that accepts an argument of one type and produces a double-valued result. Its primary use is in lambda expressions and method references where a function ... Read More

734 Views
Introduction In Java, the manipulation and handling of time is a common requirement in programming tasks. The TimeUnit class, part of java.util.concurrent package, plays a crucial role in this aspect by providing a set of methods for converting time across different units. In this article, we delve into the TimeUnit class, its applications, and practical examples to illustrate its usefulness. Understanding TimeUnit in Java The TimeUnit class in Java provides methods for time conversions and thread-sleep operations with better readability and precision than standard approaches. TimeUnit defines the following time units: DAYS, HOURS, MICROSECONDS, MILLISECONDS, MINUTES, NANOSECONDS, and SECONDS, each ... Read More