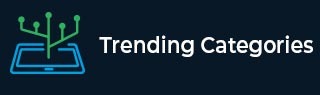
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

153 Views
Java, with its robust object-oriented programming features, offers a multitude of mechanisms for programmers to develop flexible and efficient code. One such concept, often overlooked but critically important, is variance. Understanding variance is crucial for mastering Java, especially when working with generics and collections. This article provides an in-depth exploration of variance in Java, covering its types - covariance, contravariance, and invariance - and their practical applications. Understanding Variance Variance refers to how subtyping between more complex types relates to subtyping between their components. In simpler terms, it determines how the type hierarchy of classes is preserved when these classes ... Read More

554 Views
Java is a statically-typed language known for its verbosity and strict type checking. However, with the release of Java 10, a new feature called Local-Variable Type Inference was introduced, bringing the var keyword to the language and changing the way Java developers code. This article will explore the var keyword, illustrating its use cases and discussing its implications for Java coding practices. Understanding 'var' in Java In Java, traditionally, we needed to explicitly declare the type of every variable we created. With the introduction of var in Java 10, this has changed. The var keyword allows you to declare ... Read More

62 Views
In Java, the main() method is the entry point from where the JVM begins program execution. If you've written a Java program, you're likely familiar with the traditional main() signature: public static void main(String[] args). However, did you know that there are several valid variants of the main() method in Java? This article delves into the versatility of main() in Java, showcasing its multiple valid formats and explaining their intricacies. The Canonical Main() Method Before delving into its public static void main(String[] args) In this format, public denotes that the method can be accessed from anywhere; static ... Read More

515 Views
Exception handling is a fundamental aspect of Java programming that enhances the robustness of applications and promotes a seamless user experience. Key to this is understanding how to effectively use the throw, catch, and instanceof keywords to manipulate exceptions in Java. In this article, we will delve into the usage of these three mechanisms and illustrate how they collaboratively handle exceptions in Java. Understanding Exceptions in Java In Java, an exception is an event that disrupts the normal flow of a program. It's an object which is thrown by a method and caught by another method. The Java system itself ... Read More

583 Views
In Java's object-oriented paradigm, inheritance plays a significant role, allowing developers to create classes that reuse, extend, and modify the behavior defined in other classes. To facilitate seamless interaction between a superclass and its subclass, Java provides the super keyword. This article will focus on understanding and effectively using the super keyword in Java to call a base class constructor Exploring the Super Keyword The super keyword in Java is a reference variable that is used to refer to the immediate parent class object. Whenever you create an instance of a subclass, an instance of the parent class is also ... Read More

122 Views
In the domain of concurrent programming in Java, controlling access to shared resources is crucial. This need is often fulfilled through synchronization mechanisms such as locks and monitors. However, these tools typically only protect a single instance of a resource. What if you have multiple copies of a resource and you need to control access to them? This is where Semaphores come into play. In this article, we will delve into the usage of Semaphores to protect more than one copy of a resource in Java. Understanding Semaphores Semaphore is a synchronization mechanism that controls access to one or more ... Read More

253 Views
In the dynamic world of Java programming, achieving optimal performance is often the central goal of developers. In this realm, different wait strategies, including busy spinning, can play a pivotal role. This article aims to provide a detailed understanding of busy spinning as a wait strategy in Java, why it's important, and how it can be efficiently utilized. Understanding Wait Strategies In concurrent programming, wait strategies determine how a thread should wait when there is no work available for it. Different wait strategies can drastically impact the performance of concurrent applications. A commonly used approach is blocking, where a thread ... Read More

82 Views
In the world of Java programming, Google's open-source Guava library introduces powerful utilities that bolster the Java developer's toolkit. Among these, Guava's Collectors bring a unique enhancement, enabling a seamless transition of data from streams to immutable collections. This article provides a detailed guide on leveraging Guava's Collectors for collecting streams into immutable collections in Java. The Power of Immutable Collections Immutable objects have a fixed state after their creation, which means they cannot be modified. This property brings a host of benefits, including simplicity, thread-safety, and the guarantee that they will always remain in a consistent state. Java's core ... Read More

67 Views
Geometrical computations play an essential role in various domains of computer science such as computer graphics, gaming, and computational geometry. Among the myriad of geometric operations, determining whether two lines intersect is a fundamental problem. In this article, we'll take an in-depth look at how to test if two lines intersect using the above/below primitive method in Java Understanding the Concept The above/below primitive is a fundamental concept in computational geometry. It helps determine whether a point lies above, below, or on a line. To assess whether two lines intersect in the two-dimensional plane, one needs to check if the ... Read More

255 Views
HashMap, a frequently employed data structure in the Java programming language, enables programmers to store key-value pairs. This data structure is an exceedingly efficient method for storing data and allows for swift value retrieval based on keys. However, on occasion, it may become necessary to arrange the HashMap by its keys or values. In this article, we will delve into the procedures for sorting a HashMap in Java by its keys and values, as well as examine the performance implications associated with each technique. Time complexity and HashMap The time complexity of the above technique for sorting a HashMap by ... Read More