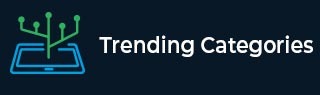
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

544 Views
Suppose we are in a situation where we need to generate random integer numbers within a specific range through Java programs. For the given scenario, there are two distinct ways available in Java. We can use either the Random class or random() method. Let’s discuss them in the next section. Generate Random Integers within a Specific Range We are going to use the following class and method − Random Class We create an object of this class to return pseudorandom numbers within a given range.We will customize this object and apply our own logic to generate any random valueswithin the ... Read More

807 Views
ArrayList is a class of Java Collection Framework that implements List Interface. It is a linear structure that stores and accesses each element sequentially. It allows the storage of duplicate elements however, there are a few approaches that may help to get unique values from an ArrayList. In this article, we are going to see the practical implementation of those approaches through Java example programs. Java Program to get Unique Values from ArrayList Before jumping to the solution program for the given problem, let’s discuss the following concepts of Collection Interface − HashSet It is a class of Java Collection ... Read More

331 Views
Generally, the Queue follows the First in First out (FIFO) approach but a PriorityQueue follows a priority based approach while accessing elements. Each element of the queue has a priority associated with it. The elements are prioritized based on natural sorting order. However, we can provide custom orders using a comparator. The elements of PriorityQueue are not actually sorted, they are only retrieved in sorted order. This feature allows us to modify an element of PriorityQueue easily. Java Program to modify an element of a ProrityQueue Before jumping into the program, let’s familiarize ourselves with a few in-built methods of ... Read More

928 Views
Getter and setter are two special methods in Java that allow accessing and modifying data members' values. They are mostly used in encapsulation and data hiding to protect our sensitive data from unauthorized access. In encapsulation, we group related data and behavior together in a class and hide the implementation details from the outside world. Data hiding means preventing direct access of the members of class from the internal state of an object. In this article, we will explain what getter and setter methods are in Java and how they are useful in data hiding. Getter and Setter methods The ... Read More

774 Views
Producer Consumer is the most common problem of Java concurrency and multi-threading. It arises during the synchronization that helps to manage multiple threads trying to access a shared resource. This article will help us to find Producer Consumer Solution using BlockingQueue in Java Thread. Producer Consumer Problem and BlockingQueue Understanding Producer Consumer Problem Producer and Consumer are two distinct entities or processes that use a shared queue. This queue is of a fixed size buffer. The producer generates pieces of information and stores them in the queue. The consumer consumes the given information and removes them from the queue. The ... Read More

135 Views
Suppose we have ‘N’ number of dice and we roll all of them at a time, then we need to show all the values that occurred on all dice. We have to emulate the same situation using Java programs. To solve this problem, we will use a class named ‘Random’ that comes under ‘java.util’ package. Java program to Emulate N Dice Roller Random Class We create an object of this class to generate pseudorandom numbers within a given range. We will customize this object and apply our own logic to select any random values from the specified dice. To retrieve ... Read More

1K+ Views
Servlets are small Java modules that are used on the server side of a web connection to enhance the functionality of web server. All the methods and classes for creating a servlet are available in ‘javax.servlet’ and ‘javax.servlet.http’ packages. Hence, it is important to import them into your program before working with the servlet They are useful when it comes to creating dynamic web pages and processing user inputs. This article aims to discuss all the necessary steps to create a servlet in detail. Steps to create a Servlet Before moving to the steps let’s discuss briefly about servlets. How ... Read More

315 Views
In Java, the IntFunction interface is a functional interface that represents a function that accepts an integer type value as an argument and returns a result of any data type. Here, the functional interface means an interface that contains only a single abstract method and exhibits single functionality. Some examples of functional interfaces are Predicate, Runnable, and Comparable interfaces. The IntFunction interface is defined in the 'java.util.function' package. In this article, we are going to explore the IntFunction Interface and its built-in methods with the help of example programs. IntFunction Interface in Java The IntFunction Interface has a ... Read More

448 Views
TreeMap is a class of Java Collection Framework that implements NavigableMap Interface. It stores the elements of the map in a tree structure and provides an efficient alternative to store the key-value pairs in sorted order. Internally, TreeMap uses a red-black tree, which is a self-balancing binary search tree. A TreeMap must implement the Comparable Interface or a custom Comparator so that it can maintain the sorting order of its elements otherwise, we will encounter a java.lang.ClassCastException. This article aims to explain how TreeMap works internally in Java. Internal Working of TreeMap in Java To understand the internal ... Read More

399 Views
In Java, both Integer.parseInt() and Integer.valueOf() methods are used for the conversion of a String into an Integer. These static methods belong to the Integer class of java.lang package and throws a NumberFormatException if the string is not a valid representation of an integer. But wait, the question arise here is that why Java provides two methods with the same functionality. Although they are used to serve similar jobs, there exist a few distinctions between them in terms of syntax and return type. In this article, we are going to explain the difference between Integer.parseInt() and Integer.valueOf() methods with ... Read More