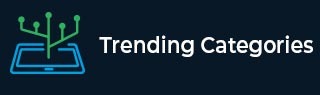
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

247 Views
To get the set view of keys from a HashMap in Java, we can use the in-built method named ‘keySet()’. Here, the HashMap is a class that is used to implement Map Interface. It stores its element in key-value pairs. The Key is an object that is used to fetch and receive value associated with it. It has access to all the methods of Map Interface, it does not have any additional methods of its own. Duplicate values are not allowed, although we can store null values and keys. Java Program to Get Set View of Keys from HashMap keySet() ... Read More

2K+ Views
To get the synchronized Map from a Hash Map in Java, we can use an in-built method of Collection interface named ‘synchronized Map()’. Here, the Hash Map is a class that is used to implement Map Interface. It stores its element in key-value pairs. The Key is an object that is used to fetch and receive value associated with it. It has access to all the methods of Map Interface, it does not have any additional methods of its own. Duplicate values are not allowed, although we can store null values and keys. In this article, we will explore the ... Read More

238 Views
To get the synchronized Map from a TreeMap in Java, we can use an in-built method of Collection interface called ‘synchronizedMap()’. Here, TreeMap is a class that is used to implement NavigableMap Interface. It stores the elements of the map in a tree structure. It provides an efficient alternative to store the key-value pairs in sorted order. By default, a TreeMap is not synchronized. In this article, we will explain the need for synchronization and its practical implementation through example programs. Synchronized Map from a Tree Map A Tree Map is not thread-safe which means when we implement it in ... Read More

97 Views
ArrayList is a class of Java Collection Framework that implements List Interface. It is a linear structure that stores and accesses each object in a sequential manner. It allows the storage of duplicate values. Always remember, each class of the collection framework can hold instances of wrapper classes or custom objects. They do not work with primitives. This article aims to explain how those objects hold by an ArrayList in Java. How ArrayList holds Objects ArrayList internally uses an array to store its elements. However, the size of the array is not fixed, it can increase and decrease as per ... Read More

157 Views
In Java, concurrent programming is a technique that allows multiple tasks or processes to run simultaneously on a single processor or multiple processors. It can improve the performance and responsiveness of applications. However, it also introduces new challenges and complexities to the Java developers, such as synchronization and deadlock. In this article, we will explore some of the different approaches to concurrent programming such as multithreading and executors. Concurrent Programming in Java The following three components of Java are used for Concurrent Programming java.lang.Thread clas java.lang.Runnable java.util.concurrent Multithreading It is a feature of the Java programming language that ... Read More

538 Views
The most frequent query asked by beginner programmers, especially those who have already worked with C and C++ in the past, is whether Java supports pass-by-referenceor pass-by-value. Generally, programming languages use pass-by-value and pass-byreferencefor passing parameters to a method. However, Java does not support both approaches rather it uses pass-by-value to pass both primitive and reference type values. But, it provides a few ways to achieve pass-by-reference, we will explore those through this article. Ways to Achieve Paas By Reference Let’s start this discussion by understanding the storage mechanism of Java. The reference variables, names of methods and classes are ... Read More

205 Views
The Java filter() method allows us to strain elements of the stream based on the specified condition. It is a part of higher-order function that is used to apply a certain behavior on stream items. This method takes a predicate as an argument and returns a list of elements that match the predicate. But the question arises here is that how this filter() method works in the background. This article aims to explain this question through some example programs. Working of filter() method in Background Before going deep into the filter() method, let’s familiarize ourselves with I/O Streams. Itis an ... Read More

1K+ Views
The Hashtable class is a part of the Java Collection Framework that stores its element in key-value pairs in a hash table. The Key is an object that can be used to fetch and receive value associated with it. There exist a few similarities between a Hashtable and HashMapclass but Hash table is synchronized. Also, its keys must be associated with values, they could not be null. This article aims to explain how Hash table works internally in Java. Working of Hashtable in Java We can consider a Hashtable as an array of buckets, where each bucket contains a list ... Read More

394 Views
The backspace terminal control character is a special character represented by the ‘\b’notation. It is used to move the cursor one character back. It comes under Java escape characters, these characters are used with backslash (\) and hold a special meaning to the compiler. In this article, we will understand and see the practical implementation of‘\b’ notation through Java example programs. Working of Backspace Terminal Control Character Two types of situations may arise while working with this escape character. First, when we hard code the backspace character into a String and the second, when we take input using a keyboard. ... Read More

618 Views
Java provides different ways to copy files including the ‘File’, ‘FileInputStream’ and‘FileOutputStream’ classes. There are times when we need to take a backup, compress a file or share it with others. In these situations, copying that file becomes necessary. Weare going to explore the methods and classes that will help us to copy the content of one file to another file through Java programs. Before jumping to the example program directly, let’s discuss some classes and built-in methods that we will be using. This will build a foundation for understanding the code. Note that these classes and methods are associated ... Read More