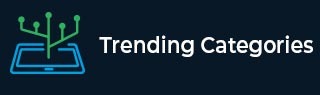
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
The HTML input value is a string. To convert the string to integer, use parseInt().ExampleFollowing is the code − Live Demo Document GetANumber function result() { var numberValue = document.getElementById("txtInput").value; if (!isNaN(numberValue)) console.log("The value=" + parseInt(numberValue)); else console.log("Please enter the integer value.."); } To run the above program, save the file name “anyName.html(index.html)”. Right click on the file and select the option “Open with ... Read More

157 Views
Let’s say the following is our object −var details = { "STUDENTNAME": "John", "STUDENTAGE": 21, "STUDENTCOUNTRYNAME": "US" }As you can see above, the keys are in capital case. We need to turn all these keys to lower case. Use toLowerCase() for this.ExampleFollowing is the code −var details = { "STUDENTNAME": "John", "STUDENTAGE": 21, "STUDENTCOUNTRYNAME": "US" } var tempKey, allKeysOfDetails = Object.keys(details); var numberOfKey = allKeysOfDetails.length; var allKeysToLowerCase = {} while (numberOfKey--) { tempKey = allKeysOfDetails[numberOfKey]; allKeysToLowerCase[tempKey.toLowerCase()] = details[tempKey]; } console.log(allKeysToLowerCase);To run the above program, you need to use the following command −node ... Read More

3K+ Views
Let’s say the following is our table − StudentName StudentCountryName JohnDoe UK DavidMiller US To get id from tr tag and display it in a new td, use document.querySelectorAll(table tr).ExampleFollowing is the code − Live Demo Document td, th, table { border: 1px solid black; margin-left: 10px; ... Read More

461 Views
Yes, you can pass default parameters in nested objects.Following is the code −ExampleFollowing is the code −function callBackFunctionDemo({ cl: { callFunctionName = "callBackFunction", values = 100 } = {} } = {}) { console.log(callFunctionName); console.log(values); } //This will print the default value. // 100 callBackFunctionDemo(); //This will print the given value. //500 callBackFunctionDemo({ cl: { values: 500 } });To run the above program, you need to use the following command −node fileName.js.Here, my file name is demo296.js.OutputThis will produce the following output on console −PS C:\Users\Amit\javascript-code> node demo296.js callBackFunction 100 callBackFunction 500

61 Views
Let’s say our original string is the following with repeated letters −var values = "DDAAVIDMMMILLERRRRR";We want to remove the repeated letters and precede letters with numbers. For this, use replace() along with regular expression.ExampleFollowing is the code −var values = "DDAAVIDMMMILLERRRRR"; var precedingNumbersInString = values.replace(/(.)\1+/g, obj => obj.length + obj[0]); console.log("The original string value=" + values); console.log("String value after preceding the numbers ="); console.log(precedingNumbersInString);To run the above program, you need to use the following command −node fileName.js. Here, my file name is demo295.js.OutputThis will produce the following output on console −PS C:\Users\Amit\javascript-code> node demo295.js The original string value=DDAAVIDMMMILLERRRRR String value ... Read More

116 Views
For this, use Object.keys() and set one key on each iteration as null using a for loop..ExampleFollowing is the code −var objectValues = { "name1": "John", "name2": "David", "address1": "US", "address2": "UK" } for (var tempKey of Object.keys(objectValues)) { var inEachIterationSetOneFieldValueWithNull = { ...objectValues, [tempKey]: null }; console.log(inEachIterationSetOneFieldValueWithNull); }To run the above program, you need to use the following command −node fileName.js.Here, my file name is demo294.js.OutputThis will produce the following output on console −PS C:\Users\Amit\javascript-code> node demo294.js { name1: null, name2: 'David', address1: 'US', address2: 'UK' ... Read More

498 Views
Use localStorage.setItem(“anyKeyName”, yourValue) to set the value in local storage and if you want to fetch value then you can use localStorage.getItem(“yourKeyName”)ExampleFollowing is the code − Live Demo Document var textBoxNameHTML = document.getElementById('txtName'); textBoxNameHTML.addEventListener('change', (e) => { localStorage.setItem("textBoxValue", e.target.value); }); To run the above program, save the file name “anyName.html(index.html)”. Right click on the file and select the option “Open with Live Server” in VS Code editor.OutputThis will produce the following output on console −Now, I ... Read More

404 Views
Let’s say we have the following array −var studentDetails = [ [89, "John"], [78, "John"], [94, "John"], [47, "John"], [33, "John"] ];And we need to sort the array on the basis of the first item i.e. 89, 78, 94, etc. For this, use sort().ExampleFollowing is the code −var studentDetails = [ [89, "John"], [78, "John"], [94, "John"], [47, "John"], [33, "John"] ]; studentDetails.sort((first, second) => second[0] - first[0]) console.log(studentDetails);To run the above program, you need to use the ... Read More

677 Views
Let’s say the following is our array −var details = [ { studentName: "John", studentMarks: [78, 98] }, { studentName: "David", studentMarks: [87, 87] }, { studentName: "Bob", studentMarks: [48, 58] }, { studentName: "Sam", studentMarks: [98, 98] }, ]We need to remove the duplicate property value i.e. 87 is repeating above. We need to remove it.For this, use the concept of map().ExampleFollowing is the code −var details ... Read More

214 Views
For this, use the addEventListener() with mousedown event.ExampleFollowing is the code − Document document.addEventListener("mousedown", function () { console.log("Mouse down event is happening"); }); To run the above program, save the file name “anyName.html(index.html)”. Right click on the file and select the option “Open with Live Server” in VS Code editor.OutputThis will produce the following output on console −When you click the mouse, the event will generate. The console output is shown below −