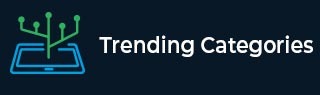
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

100 Views
Suppose, we have two arrays of literals that contain the same number of elements. We are supposed to write a function that checks whether or not the both arrays contain the same elements appearing for the same number of times.If the arrays fulfil this condition, we return true, false otherwise.We will create a copy of the second array, and start iterating over the first array. As we iterate, we will keep deleting the elements from the second array that are present in first array. If during iteration we encounter any element that isn't present in second array, we return false. ... Read More

133 Views
We are required to write a JavaScript function that takes in two arrays of literals. Then our function should return true if all the elements of first array are included in the second array, irrespective of their count, false otherwise.We have to use Array.prototype.every() method to make these comparisons.ExampleThe code for this will be −const arr1 = [0, 2, 2, 2, 1]; const arr2 = [0, 2, 2, 2, 3]; const compareArrays = (arr1, arr2) => { const areEqual = arr1.every(el => { return arr2.includes(el); }); return areEqual; }; console.log(compareArrays(arr1, arr2));OutputAnd the output in the console will be −false

285 Views
Suppose, we have an array of mixed data types like this −const arr = [1, 2, 3, 4, 5, "4", "12", "2", 6, 7, "4", 3, "2"];We are required to write a JavaScript function that takes in one such array and returns the average of all such elements that are a number or can be partially or fully converted to a number.The string "3454fdf", isn't included in the problem array, but if it wasn’t there, we would have used the number 3454 as its contribution to average.ExampleThe code for this will be −const arr = [1, 2, 3, 4, 5, ... Read More

210 Views
Suppose, we have an array of objects like this −const arr = [ {'TR-01':1}, {'TR-02':3}, {'TR-01':3}, {'TR-02':5}];We are required to write a JavaScript function that takes in one such array and sums the value of all identical keys together.Therefore, the summed array should look like −const output = [ {'TR-01':4}, {'TR-02':8}];ExampleThe code for this will be −const arr = [ {'TR-01':1}, {'TR-02':3}, {'TR-01':3}, {'TR-02':5}]; const sumDuplicate = arr => { const map = {}; for(let i = 0; i < arr.length; ){ const key = Object.keys(arr[i])[0]; if(!map.hasOwnProperty(key)){ map[key] ... Read More

4K+ Views
Suppose, we have an array of objects like this −const arr = [{id: 1, date: 'Mar 12 2012 10:00:00 AM'}, {id: 2, date: 'Mar 8 2012 08:00:00 AM'}];We are required to write a JavaScript function that takes in one such array and sorts the array according to the date property of each object.(Either newest first or oldest first).The approach should be to convert these into JS Date Object and compare their timestamps to sort the array.ExampleThe code for this will be −const arr = [{id: 1, date: 'Mar 12 2012 10:00:00 AM'}, {id: 2, date: 'Mar 8 2012 08:00:00 AM'}]; ... Read More

2K+ Views
Suppose we have any number and are required to write a JavaScript function that takes in a number and returns its Indian currency equivalent.toCurrency(1000) --> ₹4,000.00 toCurrency(129943) --> ₹1,49,419.00 toCurrency(76768798) --> ₹9,23,41,894.00ExampleThe code for this will be −const num1 = 1000; const num2 = 129943; const num3 = 76768798; const toIndianCurrency = (num) => { const curr = num.toLocaleString('en-IN', { style: 'currency', currency: 'INR' }); return curr; }; console.log(toIndianCurrency(num1)); console.log(toIndianCurrency(num2)); console.log(toIndianCurrency(num3));OutputAnd the output in the console will be −₹1,000.00 ₹1,29,943.00 ₹7,67,68,798.00

832 Views
We are required to write a JavaScript function that takes in an array of numbers of any length and returns their LCM.We will approach this problem in parts −Part 1 − We will create a helper function to calculate the Greatest Common Divisor (GCD) of two numbersPart 2 − Then using Part 1 helper function we will create another helper function to calculate the Least Common Multiple (LCM) of two numbers.Part 3 − Finally, using Part 2 helper function we will create a function that loops over the array and calculates the array LCM.ExampleThe code for this will be −const ... Read More

344 Views
We are required to write a JavaScript function that takes in three arguments −height --> no. of rows of the array width --> no. of columns of the array val --> initial value of each element of the arrayThen the function should return the new array formed based on these criteria.ExampleThe code for this will be −const rows = 4, cols = 5, val = 'Example'; const fillArray = (width, height, value) => { const arr = Array.apply(null, { length: height }).map(el => { return Array.apply(null, { length: width }).map(element => { ... Read More

455 Views
Suppose, we have this JSON object where index keys are mapped to some literals −const obj = { "0": "Rakesh", "1": "Dinesh", "2": "Mohit", "3": "Rajan", "4": "Ashish" };We are required to write a JavaScript function that takes in one such object and uses the object values to construct an array of literals.ExampleThe code for this will be −const obj = { "0": "Rakesh", "1": "Dinesh", "2": "Mohit", "3": "Rajan", "4": "Ashish" }; const objectToArray = (obj) => { const res = []; const keys = Object.keys(obj); keys.forEach(el => { res[+el] = obj[el]; }); return res; }; console.log(objectToArray(obj));OutputAnd the output in the console will be −[ 'Rakesh', 'Dinesh', 'Mohit', 'Rajan', 'Ashish' ]

620 Views
We are required to write a JavaScript function that takes in a JSON object as one and only argument.The JSON object has string keys mapped to some numbers. Our function should traverse through the object, find and return the smallest value from the object.ExampleThe code for this will be −const obj = { "a": 4, "b": 2, "c": 5, "d": 1, "e": 3 }; const findSmallestValue = obj => { const smallest = Object.keys(obj).reduce((acc, val) => { return Math.min(acc, obj[val]); }, Infinity); return smallest; } console.log(findSmallestValue(obj));OutputAnd the output in the console will be −1