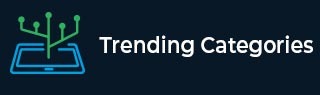
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

71 Views
We are required to write a JavaScript function that takes in an array of numbers. Our function should return true if the difference between all adjacent elements is the same positive number, false otherwise.ExampleThe code for this will be −const arr = [4, 7, 10, 13, 16, 19, 22]; const growingMarginally = arr => { if(arr.length

89 Views
const sort = ["this", "is", "my", "custom", "order"]; const myObjects = [ {"id":1, "content":"is"}, {"id":2, "content":"my"}, {"id":3, "content":"this"}, {"id":4, "content":"custom"}, {"id":5, "content":"order"} ];We are required to write a JavaScript function that takes in two such arrays and sorts the second array of objects on the basis of the first array so that the content property of objects are matched with the strings of the first array.Therefore, for the above arrays the output should look like −const output = [ {"id":3, "content":"this"}, {"id":1, "content":"is"}, {"id":2, "content":"my"}, {"id":4, "content":"custom"}, {"id":5, "content":"order"} ];ExampleThe ... Read More

296 Views
We are required to write a JavaScript function that takes in an array of Numbers. The array may contain more than one greatest element (i.e., repeating greatest element).We are required to write a JavaScript function that takes in one such array and returns all the indices of the greatest element.ExampleThe code for this will be −const arr = [10, 5, 4, 10, 5, 10, 6]; const findGreatestIndices = arr => { const val = Math.max(...arr); const greatest = arr.reduce((indexes, element, index) => { if(element === val){ return indexes.concat([index]); } else { return indexes; }; }, []); return greatest; } console.log(findGreatestIndices(arr));OutputAnd the output in the console will be −[ 0, 3, 5 ]

235 Views
We are required to write a JavaScript function that takes in an array of two numbers as the first argument, this array specifies a number range within which we can generate random numbers.The second argument will be a single number that specifies the count of random numbers we have to generate.Then at last our function should return the greatest all random numbers generated.ExampleThe code for this will be −const range = [15, 26]; const count = 10; const randomBetweenRange = ([min, max]) => { const random = Math.random() * (max - min) + min; return random; }; const ... Read More

213 Views
We are required to write a JavaScript function that takes in a number, say num.Then our function should return the sum of all the natural numbers between 1 and num, including 1 and num.For example, if num is −const num = 5;Then the output should be −const output = 15;because, 1+2+3+4+5 = 15We will use the below formula to solve this problem −Sum of all natural number upto n =((n*(n+1))/2)ExampleThe code for this will be −const num = 5; const sumUpto = num => { const res = (num * (num + 1)) / 2; return res; }; ... Read More

81 Views
We are required to write a JavaScript function that takes in an array of literals.Then the function should shuffle the order of elements in any random order inplace.ExampleThe code for this will be −const letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g']; const unorderArray = arr => { let i, pos, temp; for (i = 0; i < 100; i++) { pos = Math.random() * arr.length | 0; temp = arr[pos]; arr.splice(pos, 1); arr.push(temp); }; } unorderArray(letters); console.log(letters);OutputAnd the output in the console will ... Read More

322 Views
Suppose we have a complex JSON Object like this −const obj = { "id": "0001", "fieldName": "sample1", "fieldValue" "0001", "subList": [ { "id": 1001, "fieldName": "Sample Child 1", "fieldValue": "1001", "subList": [] }, { "id": 1002, "fieldName": "Sample Child 2", "fieldValue": "1002", "subList": [] } ] }We are required to write ... Read More

362 Views
We are required to write a JavaScript function that takes in an alphabet string and a number, say n. We should then return a new string in which all the characters are replaced by respective alphabets at position n alphabets next to them.For example, if the string and the number are −const str = 'abcd'; const n = 2;Then the output should be −const output = 'cdef';ExampleThe code for this will be −const str = 'abcd'; const n = 2; const replaceNth = (str, n) => { const alphabet = 'abcdefghijklmnopqrstuvwxyz'; let i, pos, res = ''; ... Read More

2K+ Views
Suppose we have a JSON Object that contains a nested array like this −const arr = { "DATA": [ { "BookingID": "9513", "DutyStart": "2016-02-11 12:00:00" }, { "BookingID": "91157307", "DutyStart": "2016-02-11 13:00:00" }, { "BookingID": "95117317", "DutyStart": "2016-02-11 13:30:00" }, { "BookingID": "957266", "DutyStart": "2016-02-12 19:15:00" ... Read More

2K+ Views
Suppose we have an array of objects like this −const arr = [{id:1, name:"aa"}, {id:2, name:"bb"}, {id:3, name:"cc"}];We are required to write a JavaScript function that takes in one such array and returns an object of the object where the key of each object should be the id property.Therefore, the output should look like this −const output = {1:{name:"aa"}, 2:{name:"bb"}, 3:{name:"cc"}};Notice that the id property is used to map the sub-objects is deleted from the sub-objects themselves.ExampleThe code for this will be −const arr = [{id:1, name:"aa"}, {id:2, name:"bb"}, {id:3, name:"cc"}]; const arrayToObject = arr => { const res ... Read More