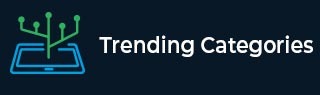
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

60 Views
Consider the following input and output arrays −const input = ["0:3", "1:3", "4:5", "5:6", "6:8"]; const output = [ [0, 1, 3], [4, 5, 6, 8] ];Considering each number as a node in a graph, and each pairing x:y as an edge between nodes x and y, we are required to find the sets of numbers that can be traveled to using the edges defined.That is, in graph theory terms, find the distinct connected components within such a graph. For instance, in the above arrays, there is no way to travel from 4 to 0 so they are ... Read More

77 Views
We are required to write a JavaScript function that takes in an array of arrays of Numbers as the first argument and an array of Numbers as the second argument. The function should pick a subarray from each array of the first array, (subarray that contains item common to both the second array and the corresponding array of first array.)For example −If the inputs are −Exampleconst arr1 = [ [1, 2, 5, 6], [5, 13, 7, 8], [9, 11, 13, 15], [13, 14, 15, 16], [1, 9, 11, 12] ]; const arr2 = [9, 11, 13, 15, 1, 2, 5, ... Read More

507 Views
We are required to write a JavaScript function that takes in an array of Numbers that may contain some repeating elements. The function should return the length of the longest repeating number sequence from the array.For example −If the input array is −const arr = [2, 1, 1, 2, 3, 3, 2, 2, 2, 1];Then the output should be 3 because the number 2 is repeated 3 times consecutively in the array (and that's the highest number).Exampleconst arr = [2, 1, 1, 2, 3, 3, 2, 2, 2, 1]; const findLongestSequence = (arr = []) => { const res ... Read More

311 Views
Suppose, we have two arrays like these −const input = ['S-1', 'S-2', 'S-3', 'S-4', 'S-5', 'S-6', 'S-7', 'S-8']; const sortingArray = ["S-1", "S-5", "S-2", "S-6", "S-3", "S-7", "S-4", "S-8"];We are required to write a JavaScript function that takes in two such arrays as first and second argument respectively.The function should sort the elements of the first array according to their position in the second array.The code for this will be −Exampleconst input = ['S-1', 'S-2', 'S-3', 'S-4', 'S-5', 'S-6', 'S-7', 'S-8']; const sortingArray = ["S-1", "S-5", "S-2", "S-6", "S-3", "S-7", "S-4", "S-8"]; const sortByReference = (arr1 = [], arr2 = ... Read More

1K+ Views
Suppose, we have the following JSON object −const obj = { "context": { "device": { "localeCountryCode": "AX", "datetime": "3047-09-29T07:09:52.498Z" }, "currentLocation": { "country": "KM", "lon": -78789486, } } };We are required to write a JavaScript recursive function that initially takes in one such array.The function should split the above object into a "label" - "children" format.Therefore, the output for the above object should look like −const output = { ... Read More

6K+ Views
Suppose we have the following nested JSON object −const obj = { id: 1, title: 'hello world', child: { id: null, title: 'foobar', child: { id: null, title: 'i should be in results array ' } }, foo: { id: null, title: 'i should be in results array too!' }, deep: [ { id: null, ... Read More

144 Views
Suppose, we have an array of objects like this −const arr = [ {"time":"18:00:00"}, {"time":"10:00:00"}, {"time":"16:30:00"} ];We are required to write a JavaScript function that takes in one such array and does the following −Extract the times from the json code: so: 18:00:00, 10:00:00, 16:30:00Convert the times to this: [18,0], [10,0], [16,30]Put it in an array.Return the final array.ExampleThe code for this will be −const arr = [ {"time":"18:00:00"}, {"time":"10:00:00"}, {"time":"16:30:00"} ]; const reduceArray = (arr = []) => { let res = []; res = arr.map(obj => { return obj['time'].split(':').slice(0, 2).map(el => { return +el; }); }); return res; }; console.log(reduceArray(arr));OutputAnd the output in the console will be −[ [ 18, 0 ], [ 10, 0 ], [ 16, 30 ] ]

702 Views
Suppose, we have an object like this −const obj = { "part1": [{"id": 1, "a": 50}, {"id": 2, "a": 55}, {"id": 4, "a": 100}], "part2":[{"id": 1, "b": 40}, {"id": 3, "b": 45}, {"id": 4, "b": 110}] };We are required to write a JavaScript function that takes in one such object. The function should merge part1 and part2 of the object to form an array of objects like this −const output = [ {"id": 1, "a": 50, "b": 40}, {"id": 2, "a": 55}, {"id": 3, "b": 45}, {"id": 4, "a": 100, "b": 110} ];ExampleThe code ... Read More

613 Views
We have an array that holds several objects named student, each object student has several properties, one of which is an array named grades −const arr = [ { name: "Student 1", grades: [ 65, 61, 67, 70 ] }, { name: "Student 2", grades: [ 50, 51, 53, 90 ] }, { name: "Student 3", grades: [ 0, 20, 40, 60 ] } ];We need to create a function that loops through the student's array ... Read More

231 Views
Suppose, we have a nested array of numbers like this −const arr = [23, 6, [2, [6, 2, 1, 2], 2], 5, 2];We are required to write a program that should print the numbers (elements) of this array to the screen.The printing order of the numbers should according to the level upto which they are nested.Therefore, the output for the above input should look like this −23 6 2 6 2 1 2 2 5 2ExampleThe code for this will be − ... Read More