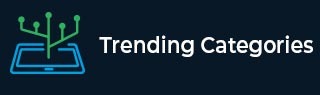
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

681 Views
We are given an array of size n, and we are required to find the majority element. The majority element is the element that appears more than [ n/2 ] times.Exampleconst arr = [2, 4, 2, 2, 2, 4, 6, 2, 5, 2]; const majorityElement = (arr = []) => { const threshold = Math.floor(arr.length / 2); const map = {}; for (let i = 0; i < arr.length; i++) { const value = arr[i]; map[value] = map[value] + 1 || 1; if (map[value] > threshold) return value }; return false; }; console.log(majorityElement(arr));OutputAnd the output in the console will be −2

271 Views
We are required to write an Array function (functions that lives on Array.prototype object). The function should take in a start index and an end index and it should sum all the elements from start index to end index in the array (including both start and end)Exampleconst arr = [1, 2, 3, 4, 5, 6, 7]; const sumRange = function(start = 0, end = 1){ const res = []; if(start > end){ return res; }; for(let i = start; i

864 Views
We are given two strings str1 and str2, we are required to write a function that checks if str1 is a subsequence of str2.A subsequence of a string is a new string which is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters.For example, "ace" is a subsequence of "abcde" while "aec" is notExampleconst str1 = 'ace'; const str2 = 'abcde'; const isSubsequence = (str1, str2) => { let i=0; let j=0; while(i

95 Views
We are required to write a JavaScript function that takes in an array of objects of dates like this −const arr = [ {date: "2016-06-08 18:10:00"}, {date: "2016-04-26 20:01:00"}, {date: "2017-02-06 14:38:00"}, {date: "2017-01-18 17:30:21"}, {date: "2017-01-18 17:24:00"} ];We are required to write a JavaScript function that takes in one such array. The function should then sort the array according to the date property of the objects.Exampleconst arr = [ {date: "2016-06-08 18:10:00"}, {date: "2016-04-26 20:01:00"}, {date: "2017-02-06 14:38:00"}, {date: "2017-01-18 17:30:21"}, {date: "2017-01-18 17:24:00"} ]; const sortByTime = (arr ... Read More

2K+ Views
Suppose, we have two objects like these −const obj1 = { a:12, b:8, c:17 }; const obj2 = { a:2, b:4, c:1 };We are required to write a JavaScript function that takes in two such object.The function should sum the values of identical properties into a single property. Therefore, the final object should look something like this −const output = { a:14, b:12, c:18 };Note − For the sake of simplicity, we have just used two objects, but we are required to write our function such that it can take in any number of objects and add ... Read More

589 Views
Suppose, we have the following array of objects −const arr = [ { "date" : "2010-01-01", "price" : 30 }, { "date" : "2010-02-01", "price" : 40 }, { "date" : "2010-03-01", "price" : 50 }, { "date" : "2010-01-01", "price2" : 45 }, { "date" : "2010-05-01", "price2" : 40 }, { "date" : "2010-10-01", ... Read More

4K+ Views
Suppose, we have an array of objects like this −const arr = [{ "value": 10, "id": "111", "name": "BlackCat", }, { "value": 10, "id": "111", "name": "BlackCat", }, { "value": 15, "id": "777", "name": "WhiteCat", }];We are required to write a JavaScript function that takes in one such array.The function should then merge all those objects together that have the common value for "id" property.Therefore, for the above array, the output should look like −const output = [{ "value": 10, "id": "111", "name": "BlackCat", "count": 2, ... Read More

108 Views
We are required to write a JavaScript function that takes in two strings, say str1 and str2. We are required to determine whether or not the second string is a rotated version of the first string.For example− If the input strings are −const str1 = 'abcde'; const str2 = 'cdeab';Then the output should be true because str2 is indeed made by shifting 'ab' to the end of string in str1.Exampleconst str1 = 'abcde'; const str2 = 'cdeab'; const isRotated = (str1, str2) => { if(str1.length !== str2.length){ return false }; if( (str1.length || str2.length) ... Read More

521 Views
We are required to write a JavaScript function that takes in a sorted array of numbers as the first argument and a search number as the second argument.If the search number exists in the array, we need to return its index in the array, otherwise we need to return -1.We have to do this making use of the binary search algorithm. The binary search algorithm is basically a divide and conquer algorithm which recursive divides the array into halves until it converses to a singleton element.The sorting of array is necessary of binary search algorithm in this case, as it ... Read More

329 Views
We are required to write a JavaScript function that takes in a string that might contain spaces. Our function should first split the string based on the spaces and then reverse and join and return the new string.For example − If the input string is −const str = 'this is a word';Then the output should be −const output = 'siht si a drow';Exampleconst str = 'this is a word'; const reverseWords = (str = '') => { const strArr = str.split(' '); for(let i = 0; i < strArr.length; i++){ let el = strArr[i]; ... Read More