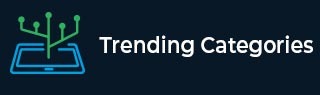
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

198 Views
We are required to write a JavaScript function that takes in an array of numbers as the first and the only argument.The function should then return the length of the longest continuous subarray from the array that only contains elements in a strictly increasing order.A strictly increasing sequence is the one in which any succeeding element is greater than all its preceding elements.Exampleconst arr = [5, 7, 8, 12, 4, 56, 6, 54, 89]; const findLongest = (arr) => { if(arr.length == 0) { return 0; }; let max = 0; let count ... Read More

477 Views
The degree of an array of literals is defined as the maximum frequency of any one of its elements.const arr = [1, 2, 3, 3, 5, 6, 4, 3, 8, 3];The degree of this array is 4, because 3 is repeated 4 times in this array.We are required to write a JavaScript function that takes in an array of literals. The task of our function is to find the length of the smallest continious subarray from the array whose degree is same as of the whole array.Exampleconst arr = [1, 2, 3, 3, 5, 6, 4, 3, 8, 3]; const ... Read More

1K+ Views
Suppose, we have the following JSON object −const obj = { "test1": [{ "1": { "rssi": -25, } }, { "2": { "rssi": -25, } }], "test2": [{ "15": { "rssi": -10, } }, { "19": { "rssi": -21, } }] };We are required to write a JavaScript function that takes in an object like this −The ... Read More

131 Views
Suppose we have an array of objects like this −const arr = [ {userId: "3t5bsFB4PJmA3oTnm", from: 1, to: 6}, {userId: "3t5bsFB4PJmA3oTnm", from: 7, to: 15}, {userId: "3t5bsFB4PJmA3oTnm", from: 172, to: 181}, {userId: "3t5bsFB4PJmA3oTnm", from: 182, to: 190} ];We are required to write a JavaScript function that takes in one such array. The function should group overlapping objects based on their "from" and "to" property into a single object like this −const output = [ {userId: "3t5bsFB4PJmA3oTnm", from: 1, to: 15}, {userId: "3t5bsFB4PJmA3oTnm", from: 172, to: 190} ];Exampleconst arr = [ {userId: "3t5bsFB4PJmA3oTnm", from: ... Read More

291 Views
We are required to write a JavaScript function that in a positive integer, say n. The function should find and return the longest distance between any two adjacent 1's in the binary representation of n.If there are no two adjacent 1's, then we have to return 0.Two 1's are adjacent if there are only 0's separating them (possibly no 0's). The distance between two 1's is the absolute difference between their bit positions. For example, the two 1's in "1001" have a distance of 3.For example −If the input is 22, then the output should be 2, because, The binary ... Read More

1K+ Views
We are required to write a JavaScript function that takes in an array of literals as the first argument and a search string as the second argument.The function should the array for the that search string. If that string exists in the array, we should return its next element from the array, otherwise we should return false.Exampleconst arr = ["", "comp", "myval", "view", "1"] const getNext = (value, arr) => { const a = [undefined].concat(arr) const p = a.indexOf(value) + 1; return a[p] || false; } console.log(getNext('comp', arr)); console.log(getNext('foo', arr));OutputAnd the output in the console will be ... Read More

1K+ Views
We are required to write a program (function) that takes in an object reference and returns an array of all the methods (member functions) that lives on that object.We are only required to return the methods in the array and not any other property that might have value of type other than a function.We will use the Object.getOwnPropertyNames functionThe Object.getOwnPropertyNames() method returns an array of all properties (enumerable or not) found directly upon a given object. And then we will filter the array to contain property of data type 'function' only.Exampleconst returnMethods = (obj = {}) => { const ... Read More

443 Views
Suppose, we have an object like this −const obj = { "firstName": "John", "lastName": "Green", "car.make": "Honda", "car.model": "Civic", "car.revisions.0.miles": 10150, "car.revisions.0.code": "REV01", "car.revisions.0.changes": "", "car.revisions.1.miles": 20021, "car.revisions.1.code": "REV02", "car.revisions.1.changes.0.type": "asthetic", "car.revisions.1.changes.0.desc": "Left tire cap", "car.revisions.1.changes.1.type": "mechanic", "car.revisions.1.changes.1.desc": "Engine pressure regulator", "visits.0.date": "2015-01-01", "visits.0.dealer": "DEAL-001", "visits.1.date": "2015-03-01", "visits.1.dealer": "DEAL-002" };We are required to write a JavaScript function that takes in one such object and unflattens it into nested objects and arrays.Therefore, ... Read More

303 Views
We are required to write a JavaScript function that takes in an array of literals which may contain some duplicate values.The function should return an array of all those elements that are repeated for the least number of times.For example− If the input array is −const arr = [1, 1, 2, 2, 3, 3, 3];Then the output should be −const output = [1, 2];because 1 and 2 are repeated for the least number of times (2)Exampleconst arr = [1, 1, 2, 2, 3, 3, 3]; const getLeastDuplicateItems = (arr = []) => { const hash = Object.create(null); let ... Read More

106 Views
Suppose we have an array of strings like this −const arr = [ 'type=A', 'day=45' ];We are required to write a JavaScript function that takes in one such array. The function should construct an object based on this array. The object should contain a key/value pair for each string in the array.For any string, the part before '=' becomes the key and the part after it becomes the value.Exampleconst arr = [ 'type=A', 'day=45' ]; const arrayToObject = (arr = []) => { const obj = {}; for (let i = 0; i < arr.length; i++) { ... Read More