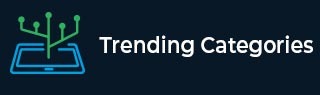
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

109 Views
Suppose, we have a long string that represents a number like this −const str = '11222233344444445666';We are required to write a JavaScript function that takes in one such string. Our function is supposed to return an object that should assign a unique "id" property to each unique number in the string and one other property "count" that stores the count of the number of times the number appears in the string.Therefore, for the above string, the output should look like −const output = { '1': { id: '1', displayed: 2 }, '2': { id: '2', displayed: 4 }, ... Read More

105 Views
We are required to write a JavaScript function that takes in an array of sorted integers and a target average as first and second arguments.The function should determine whether there is a pair of values in the array where the average of the pair equals to the target average.There's a solution with O(1) additional space complexity and O(n) time complexity. Since an array is sorted, it makes sense to have two indices: one going from begin to end (say y), another from end to begin of an array (say x).ExampleThe code for this will be −const arr = [1, 2, ... Read More

572 Views
Suppose, we have an array of arrays like this −const arr = [[12345, "product", "10"], [12345, "product", "15"], [1234567, "other", "10"]];We are supposed to write a function that takes in one such array. Notice that all the subarrays have precisely three elements in them.Our function should filter out that subarray which has a repeating value as its first element. Moreover, for the subarray, we remove we should add their third element to its existing nonrepeating counterpart.So, for the above array, the output should look like −const output = [[12345, "product", "25"], [1234567, "other", "10"]];ExampleThe code for this will be −const ... Read More

694 Views
We are required to write a JavaScript function that takes in an array of strings. The function should then generate and return all possible combinations of the strings of the array.ExampleThe code for this will be −const arr = ['a', 'b', 'c', 'd']; const permutations = (len, val, existing) => { if(len==0){ res.push(val); return; } for(let i=0; i { for(let i=0; i < arr.length; i++){ permutations(arr.length−i, "", []); } }; buildPermuations(arr); console.log(res);ExampleAnd the output in the console will be −[ 'abcd', 'abdc', 'acbd', 'acdb', 'adbc', ... Read More

131 Views
Consider the following backtracing problem: On a 2−dimensional grid, there are 4 types of squares −1 represents the starting square. There is exactly one starting square.2 represents the ending square. There is exactly one ending square.0 represents empty squares we can walk over.−1 represents obstacles that we cannot walk over.We are required to write a function that returns the number of 4−directional walks from the starting square to the ending square, that walk over every non−obstacle square exactly once.Exampleconst arr = [ [1, 0, 0, 0], [0, 0, 0, 0], [0, 0, 2, -1] ]; const uniquePaths ... Read More

229 Views
We are required to write a function that does the following −takes an array of integers as an argument (e.g. [1, 2, 3, 4])creates an array of all the possible permutations of [1, 2, 3, 4], with each permutation having a length of 4 (i.e., the length of original array)ExampleThe code for this will be −const arr = [1, 2, 3, 4]; const permute = (arr = [], res = [], used = []) => { let i, ch; for (i = 0; i < arr.length; i++) { ch = arr.splice(i, 1)[0]; ... Read More

543 Views
Suppose, we have an array of objects containing data about likes of some users like this −const arr = [ {"user":"dan", "liked":"yes", "age":"22"}, {"user":"sarah", "liked":"no", "age":"21"}, {"user":"john", "liked":"yes", "age":"23"}, ];We are required to write a JavaScript function that takes in one such array. The function should construct another array based on this array like this −const output = [ {"dan":"yes"}, {"sarah":"no"}, {"john":"yes"}, ];Exampleconst arr = [ {"user":"dan", "liked":"yes", "age":"22"}, {"user":"sarah", "liked":"no", "age":"21"}, {"user":"john", "liked":"yes", "age":"23"}, ]; const mapToPair = (arr = []) => { const result = arr.map(obj => ... Read More

131 Views
We are required to write a JavaScript function that takes in an array of Numbers as the first argument and a target sum Number as the second argument.The function should return an array of all those subarrays from the original array whose elements sum to make the target sum. We can use a single number twice to achieve the sum.For example −If the input array and number are −const arr = [1, 2, 4]; const sum = 4;then the output should be −const output = [ [1, 1, 1, 1], [1, 1, 2], [2, 2], [4] ... Read More

340 Views
Suppose, we have an array of objects like this −const arr = [ {"id":7, "name":"Kuwait", "parentId":2}, {"id":4, "name":"Iraq", "parentId":2}, {"id":10, "name":"Qatar", "parentId":2}, {"id":2, "name":"Middle East", "parentId":1}, {"id":3, "name":"Bahrain", "parentId":2}, {"id":6, "name":"Jordan", "parentId":2}, {"id":8, "name":"Lebanon", "parentId":2}, {"id":1, "name":"Africa/Middle East", "parentId":null}, {"id":5, "name":"Israel", "parentId":2}, {"id":9, "name":"Oman", "parentId":2} ];We are required to write a JavaScript function that takes in one such array. The function should then prepare a new array that contains the objects grouped according to their parents.Therefore, the output should look like this −const output = [ ... Read More

206 Views
Suppose, we have two arrays, let’s say arr1 and arr2. The elements of arr2 are distinct, and all elements in arr2 are also in arr1.We are required to write a JavaScript function that takes in two such arrays and sorts the elements of arr1 such that the relative ordering of items in arr1 are the same as in arr2. Elements that don't appear in arr2 should be placed at the end of arr1 in ascending order.For example− If the two input arrays are −const arr1 = [2, 3, 1, 3, 2, 4, 6, 7, 9, 2, 19]; const arr2 = ... Read More