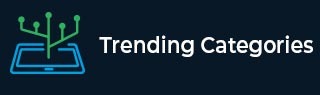
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

625 Views
We are required to write a JavaScript function that takes in a number as one and the only argument. The function should then return a randomly generated string of the length specified by the argument.The character set to be used for the string generation should only contain uppercase and lowercase alphabets (no whitespaces, punctuations or numerals).ExampleThe code for this will be −const num = 13; const randomString = (len = 1) => { const charSet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'; let randomString = ''; for (let i = 0; i < len; i++) { let randomPoz ... Read More

1K+ Views
We are required to write a JavaScript function that takes in an array of literals as the first argument and a string as the second argument. Our function should return the count of the number of times that string (provided by second argument) appears anywhere in the array.ExampleThe code for this will be −const arr = ["word", "a word", "another word"]; const query = "word"; const findAll = (arr, query) => { let count = 0; count = arr.filter(el => { return el.indexOf(query) != -1; }).length; return count; }; console.log(findAll(arr, query));OutputAnd the output in the console will be −3

131 Views
Let's say we have such an array −const arr = [A, A, B, B, C, C, D, E];We are required to create an algorithm so that it will find all the combinations that add up to the whole array, where none of the elements are repeated.Example combinations −[A, B, C, D, E] [A, B, C] [A, B, C, D] [A, B, C, E] [A, B, C] [A, B, C] [D, E]Explanation[A, B, C] [A, B, C] [D, E] and [A, B, C] [D, E] [A, B, C] are the same combinations. Also, the ordering with the subsets doesn't matter as ... Read More

2K+ Views
We are required to write a JavaScript function that takes in two arrays of equal length. The function should multiply the corresponding (by index) values in each, and sum the results.For example: If the input arrays are −const arr1 = [2, 3, 4, 5]; const arr2 = [4, 3, 3, 1];then the output should be 34, because,(4*2+3*3+4*3+5*1) = 34ExampleThe code for this will be −const arr1 = [2, 3, 4, 5]; const arr2 = [4, 3, 3, 1]; const produceAndAdd = (arr1 = [], arr2 = []) => { let sum = 0; for(let i=0; i < arr1.length; i++) { const product = (arr1[i] * arr2[i]); sum += product; }; return sum; }; console.log(produceAndAdd(arr1, arr2));OutputAnd the output in the console will be −34

1K+ Views
Suppose, we have a JSON Array that contains data about some tickets like this −const arr = [ { "quantity": "1", "description": "VIP Ticket to Event" }, { "quantity": "1", "description": "VIP Ticket to Event" }, { "quantity": "1", "description": "VIP Ticket to Event" }, { "quantity": "1", "description": "Regular Ticket to Event" }, { "quantity": "1", "description": "Regular Ticket to ... Read More

665 Views
We are required to write a JavaScript function that takes in a string of any variable length that represents a number.Our function is supposed to convert the number string to the corresponding letter string.For example − If the number string is −const str = '78956';Then the output should be −const output = 'ghief';If the number string is −const str = '12345';Then the output string should be −const output = 'lcde';Notice how we didn't convert 1 and 2 to alphabets separately because 12 also represents an alphabet. So we have to consider this case while writing our function.We, here, assume that ... Read More

2K+ Views
Suppose we have an Object of Objects like this −const obj = { "CAB": { name: 'CBSSP', position: 2 }, "NSG": { name: 'NNSSP', position: 3 }, "EQU": { name: 'SSP', position: 1 } };We are required to write a JavaScript function that takes in one such array and sorts the sub-objects on the basis of the 'position' property of sub-objects (either in increasing or decreasing order).ExampleThe code for this will be −const obj = { ... Read More

2K+ Views
Suppose, we have an array of objects that contains data about some movies like this −const arr = [ {id: "1", name: "Snatch", type: "crime"}, {id: "2", name: "Witches of Eastwick", type: "comedy"}, {id: "3", name: "X-Men", type: "action"}, {id: "4", name: "Ordinary People", type: "drama"}, {id: "5", name: "Billy Elliot", type: "drama"}, {id: "6", name: "Toy Story", type: "children"} ];We are required to write a JavaScript function that takes in one such array as the first argument and an id string as the second argument. Then our function should search for the object ... Read More

657 Views
We are required to write a JavaScript function that splits an Array of numbers into N groups, which must be ordered from larger to smaller groups.For example, in the below code, split an Array of 12 numbers into 5 Arrays, and the result should be evenly split, from large (group) to small:const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]; const output = [[1, 2, 3] [4, 5, 6] [7, 8] [9, 10] [11, 12]];The function should take in the array as the first argument and the number of partitions as the second argument.ExampleThe ... Read More

890 Views
Suppose, we have a nested object that contains data about some pets like this −const pets = { owner1: 'Frank', owner2: 'Curly', owner3: 'Maurice', dogs: { terriers: { name1: 'Fido', name2: 'Woofy', name3: { goodDog: 'Frank', badDog: 'Judas', } }, poodles: { name1: 'Curly', name2: 'Fido', }, ... Read More