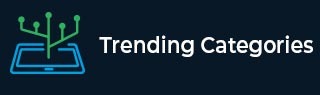
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

170 Views
The problem statement tells you to find a solution for an arbitrary number of arrays such that the user needs to find a common element present in every arbitrary array. The arbitrary array is referred to here as an object of arrays . One should not confuse finding common elements between two arrays , it is not what the problem statement is asking for. It defines and explores the variety of algorithms that can help us find the least common factors present in mutual by the given source data in JavaScript. What are Arbitrary Number of Arrays in ... Read More

1K+ Views
The problem statement says to execute Two sum problem in linear time in JavaScript. It defines and explores the variety of algorithms that can help us find the least common factors present in mutual by the given source data in JavaScript. What is the Two sum problem in JavaScript ? Two Sum Problem in JavScript equals finding a pair of indices present in the array of numbers given as an input that adds up to the target sum which is also provided as an input by the user. Given an array of integers and ... Read More

154 Views
In the given problem statement we are asked to sort an array of numbers into some sets where the array is the input source provided by the user going from brute force method to optimized solution . What is an array in JavaScript ? If you are familiar with any other programming language like C, C++, or Java, you must have heard the term 'array.' In programming, an array is a collection of similar data elements under one roof. Now, an important question arises: if arrays are generally the same in all languages, then ... Read More

3K+ Views
In the given problem statement we are asked to generate every possible permutation of the array given as input by the in JavaScript going from brute force method to optimized solution . What is an array in JavaScript ? If you are familiar with any other programming language like C, C++, or Java, you must have heard the term 'array.' In programming, an array is a collection of similar data elements under one roof. Now, an important question arises: if arrays are generally the same in all languages, then how does JavaScript make arrays more ... Read More

528 Views
We are required to write a JavaScript function that takes in an array of string literals as the first argument and a single string character as the second argument.Then our function should find and return the first array entry that starts with the character specified by the second argument.ExampleThe code for this will be −const names = ['Naman', 'Kartik', 'Anmol', 'Rajat', 'Keshav', 'Harsh', 'Suresh', 'Rahul']; const firstIndexOf = (arr = [], char = '') => { for(let i = 0; i < arr.length; i++){ const el = arr[i]; if(el.substring(0, 1) === char){ ... Read More

480 Views
We are required to write a JavaScript function that takes in a number as the only argument. The function should then return a random generated number which is always divisible by the number provided by the argument.ExampleThe code for this will be −const num = 21; // function that generates random numbers divisible by n with a default upper limit of 1000000 const specialRandom = (num = 1, limit = 1000000) => { // getting a random number const random = Math.random() * limit; // rounding it off to be divisible by num const res = ... Read More

203 Views
We are required to write a JavaScript function on the prototype object of a BinarySearchTree data type that takes in a value and finds whether or not that value is contained in the BST.ExampleThe code for this will be -// class for a single Node for BST class Node { constructor(value) { this.value = value; } } // class for BST // contains function to insert node and search for existing nodes class BinarySearchTree { constructor() { this._root = null; }; insert(value) { let node = ... Read More

192 Views
We are required to write a JavaScript function that takes in an array of exactly two numbers specifying a range.The function should then calculate the least common multiple of all the numbers within that range and return the final result.ExampleThe code for this will be −const range = [8, 3]; const gcd = (a, b) => { return !b ? a : gcd(b, a % b); } const lcm = (a, b) => { return a * (b / gcd(a,b)); }; const rangeLCM = (arr = []) => { if(arr[0] > arr[1]) (arr = [arr[1], arr[0]]); for(let x = result = arr[0]; x

1K+ Views
Let's say we have a variable “users” that contains the following string of text where each user is separated by a semicolon and each attribute of each users is separated by a comma −const users = 'Bob, 1234, Bob@example.com;Mark, 5678, Mark@example.com';We are required to write a JavaScript function that takes in one such string and splits this into a multidimensional array that looks like this −const arr = [ ['Bob', 1234, 'Bob@example.com'], ['Mark', 5678, 'Mark@example.com'] ];ExampleThe code for this will be −const users = 'Bob, 1234, Bob@example.com;Mark, 5678, Mark@example.com'; const splitByPunctuations = (str = '') => { ... Read More

252 Views
This problem takes its name by arguably the most important event in the life of the ancient historian Josephus − according to his tale, he and his 40 soldiers were trapped in a cave by the Romans during a siege.Refusing to surrender to the enemy, they instead opted for mass suicide, with a twist − they formed a circle and proceeded to kill one man every three, until one last man was left (and that it was supposed to kill himself to end the act).Josephus and another man were the last two and, as we now know every detail of ... Read More