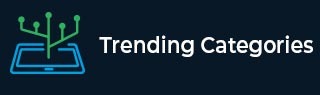
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

286 Views
Suppose, we have an array of two numbers that specify a range. We are required to write a function that finds the smallest common multiple of the provided parameters that can be evenly divided by both, as well as by all sequential numbers in the range between these parameters.The range will be an array of two numbers that will not necessarily be in numerical order.For example, if given [1, 3], then we are required to find the smallest common multiple of both 1 and 3 that is also evenly divisible by all numbers between 1 and 3. The answer here ... Read More

237 Views
Suppose, we have a JSON array of objects like this −const arr = [ { "id": "03868185", "month_10": 6, }, { "id": "03870584", "month_6": 2, }, { "id": "03870584", "month_7": 5, }, { "id": "51295", "month_1": 1, }, { "id": "51295", "month_10": 1, }, { "id": "55468", "month_11": 1, } ];Here, we ... Read More

908 Views
Suppose, we have two arrays of objects like these −const arr1 = [ {name:'test', lastname: 'test', gender:'f'}, {name:'test1', lastname: 'test1', gender:'f'}, {name:'test2', lastname: 'test2', gender:'m'} ]; const arr2 = [ {name:'test21', lastname: 'test21', gender:'f'}, {name:'test1', lastname: 'test1', gender:'f'}, {name:'test2', lastname: 'test2', gender:'m'}, {name:'test22', lastname: 'test22', gender:'m'} ];These arrays do not have any repeating objects within (repeating on the basis of 'name' property) but there exist some objects with repeating names in the first and second objects.We are required to write a JavaScript function that takes two such arrays and returns a new array.The ... Read More

145 Views
We are required to write a JavaScript function that takes in two sorted array of numbers. The function should merge the two arrays together to form a resultant sorted array and return that array.For example −If the two arrays are −const arr1 = [2, 6, 6, 8, 9]; const arr2 = [1, 4, 5, 7];Then the output array should be −const output = [1, 2, 4, 6, 6, 7, 8, 9];ExampleThe code for this will be −const arr1 = [2, 6, 6, 8, 9]; const arr2 = [1, 4, 5, 7]; const mergeSortedArrays = (arr1 = [], arr2 = []) ... Read More

498 Views
The problem statement says to perform a prepend string into all the values of array elements where the prepend string and array values will be given by the user as an input source. The problem statement can be deeply visualized as given a string text specifically to prepend or attach at the beginning of each element of the array , the output should return the new resultant array with prepended string value in combination with the value of array elements. What is an Array in JavaScript ? If you are familiar with any other programming language like C, C++, ... Read More

537 Views
The problem statement asks the user that given a binary tree , you need to find the mirror image of the elements of the binary tree such that reverse the corresponding and parallel siblings of the tree branches . In short, invert the whole binary tree given the original binary tree as an input. The output of the problem statement looks like a reverse function of the input binary tree as the summary to implement for. What is a Tree in JavaScript A tree refers to one of the optimized data structures to hold data ... Read More

317 Views
The problem statement asks the user to add all the records from one array to each record from a different array in JavaScript , the just read statement seems tough to understand and implement code upon . The simplest meaning is given two arrays of different collections of values , we need to generate a combined new array of objects such that the new generated array is a collection of every possible of values present in both arrays for say array1 and array2. The problem statement can be implemented in another way too , saying to find the Cartesian ... Read More

201 Views
The problem statement says that given a sorted array of numbers as an input source by the user such that we need to remove the duplicates or repeated elements from it making it all new array containing only unique elements inside it. What is a Sorted Array in JavaScript ? A sorted array is a kind of array in javascript that follows a certain order of line in which each element is sorted in alphabetical and numerical order all before using the sort method in javascript itself. The already sorted speed array lets you speed up the ... Read More

2K+ Views
The problem statement says to perform case sensitive sorting in javascript on the array of strings given as an input source by the user . The problem statement wants the developer to perform a case sensitive sorting where all the special characters and numerals should appear first and sort first inplace followed by the sorting of lowercase characters first before the upper case characters . Is JavaScript Case Sensitive ? Before moving forward towards the problem statement we need to understand more about the word “case-sensitive” in the context of JavaScript initially. JavaScript ... Read More

224 Views
The problem statement says to check if the string given by the user as an input can be segmented or not . What is a Segmented String ? Segmentation in string refers to breaking down words in the whole string text as an input . The words are broken down into the words of the dictionary. The problem statement is more established and dug deeper down into given some wholesome dictionaries of words and string text and we have to check if the segmented or broken down of string is compatible and equal to words of ... Read More