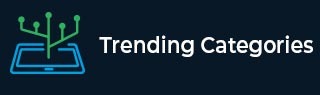
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

82 Views
We have an array that contains various objects. A few objects on this array have a date field (which basically is returned as a string from the server, not a date object), while for others this field is null.The requirement is that we have to display objects without date at top, and those with date needs to be displayed after them sorted by date field.Also, for objects without date sorting needs to be done alphabetically.Exampleconst sorter = ((a, b) => { if (typeof a.date == 'undefined' && typeof b.date != 'undefined') { return -1; } ... Read More

228 Views
Suppose, we have an array of objects like this −const arr = [ {"goods":"Wheat ", "from":"GHANA", "to":"AUSTRALIA"}, {"goods":"Wheat", "from":"USA", "to":"INDIA"}, {"goods":"Wheat", "from":"SINGAPORE", "to":"MALAYSIA"}, {"goods":"Wheat", "from":"USA", "to":"INDIA"}, ];We are required to write a JavaScript function that takes in one such array. The goal of function is to return an array of all such objects from the original array that have value "USA" for the "from" property of objects and value "INDIA" for the "to" property of objects.Exampleconst arr = [ {"goods":"Wheat ", "from":"GHANA", "to":"AUSTRALIA"}, {"goods":"Wheat", "from":"USA", "to":"INDIA"}, {"goods":"Wheat", "from":"SINGAPORE", "to":"MALAYSIA"}, {"goods":"Wheat", "from":"USA", "to":"INDIA"}, ... Read More

552 Views
Suppose, we have a string like this −const str = 'dress/cotton/black, dress/leather/red, dress/fabric, houses/restaurant/small, houses/school/big, person/james';We are required to write a JavaScript function that takes in one such string. The function should then prepare an object of arrays like this −const output = { dress = ["cotton", "leather", "black", "red", "fabric"]; houses = ["restaurant", "school", "small", "big"]; person = ["james"]; };Exampleconst str = 'dress/cotton/black, dress/leather/red, dress/fabric, houses/restaurant/small, houses/school/big, person/james'; const buildObject = (str = '') => { const result = {}; const strArr = str.split(', '); strArr.forEach(el => { const values ... Read More

157 Views
Suppose, we have a nested array of numbers like this −const arr = [ [ 0, 0, 0, −8.5, 28, 8.5 ], [ 1, 1, −3, 0, 3, 12 ], [ 2, 2, −0.5, 0, 0.5, 5.3 ] ];We are required to write a JavaScript function that takes in one such nested array of numbers. The function should combine all the numbers in the nested array to form a single string.In the resulting string, the adjacent numbers should be separated by a whitespaces and elements of two adjacent arrays should be separated by a comma.ExampleThe code for ... Read More

549 Views
Suppose, we have an array of objects like this −const arr = [ { id: '1', name: 'name 1', parentId: null }, { id: '2', name: 'name 2', parentId: null }, { id: '2_1', name: 'name 2_1', parentId: '2' }, { id: '2_2', name: 'name 2_2', parentId: '2' }, { id: '3', name: 'name 3', parentId: null }, { id: '4', name: 'name 4', parentId: null }, { id: '5', name: 'name 5', parentId: null }, { id: '6', name: 'name 6', parentId: null }, { id: '7', name: 'name 7', ... Read More

137 Views
We are required to write a JavaScript function that calculates the nth root of a number and returns it.ExampleThe code for this will be −const findNthRoot = (m, n) => { try { let negate = n % 2 == 1 && m < 0; if(negate) m = −m; let possible = Math.pow(m, 1 / n); n = Math.pow(possible, n); if(Math.abs(m − n) < 1 && (m > 0 == n > 0)) return negate ? −possible : possible; } catch(e){ return null; } }; console.log(findNthRoot(45, 6));OutputAnd the output in the console will be −1.8859727740585395

3K+ Views
The problem statement says to perform sorting of an array of objects taking in one specific condition to sort null value key pairs present in the array of objects to be pushed at the end of the array where the array of objects is given by the user as an input source. What is an Array in JavaScript ? If you are familiar with any other programming language like C, C++, or Java, you must have heard the term 'array.' In programming, an array is a collection of similar data elements under one roof. Now, an important question ... Read More

342 Views
The problem statement says to perform a complete conversion of array of objects into an object of arrays in JavaScript where array of objects are given as an input source by the user and given input can be totally converted into the resultant object of arrays successfully. What is an array in JavaScript ? If you are familiar with any other programming language like C, C++, or Java, you must have heard the term 'array.' In programming, an array is a collection of similar data elements under one roof. Now, an important question arises: if arrays are generally the ... Read More

78 Views
We are required to write a JavaScript function that takes in a number. The function should divide the number into chunks according to the following rules −The number of chunks should be a power−of−two, Each chunk should also have a power-of-two number of items (where size goes up to a max power of two, so 1, 2, 4, 8, 16, 32, 32 being the max)Therefore, for example, 8 could be divided into 1 bucket −[8]9 could be −[8, 1]That works because both numbers are powers of two, and the size of the array is 2 (also a power of two).Let's ... Read More

1K+ Views
Suppose, we have an array of objects containing some data about some users like this −const arr = [ { "name":"aaa", "id":"2100", "designation":"developer" }, { "name":"bbb", "id":"8888", "designation":"team lead" }, { "name":"ccc", "id":"6745", "designation":"manager" }, { "name":"aaa", "id":"9899", "designation":"sw" } ];We are required to write a JavaScript function that takes in one such array. Then our ... Read More