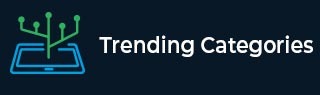
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

476 Views
We are required to write a JavaScript function that takes in an array of Numbers. The array of numbers can contain both positive as well as negative numbers.The purpose of our function is to find the sub array from the array (of any length), whose elements when summed gives the maximum sum. Then the function should return the sum of the elements of that subarray.For example −If the input array is −const arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4];Then the output should be −const output = 6because, [4, -1, 2, 1] has the largest sum of ... Read More

142 Views
We are required to write a JavaScript function that takes in two numbers say m and n. Then function should calculate and return m^n.For example − For m = 4, n = 3, thenpower(4, 3) = 4^3 = 4 * 4 * 4 = 64 power(6, 3) = 216The code for this will be the following using the power() function in JavaScript −Exampleconst power = (m, n) => { if(n < 0 && m !== 0){ return power(1/m, n*-1); }; if(n === 0){ return 1; } if(n === 1){ return m; }; if (n % 2 === 0){ const res = power(m, n / 2); return res * res; }else{ return power(m, n - 1) * m; }; }; console.log(power(4, 3)); console.log(power(6, 3));OutputAnd the output in the console will be −64 216

367 Views
We are required to write a JavaScript function that takes in an array of literals. The function should find whether or not all the values in the array are same. If they are same, the function should return true, false otherwise.Exampleconst arr1 = [1, 2, 3]; const arr2 = [1, 1, 1]; const checkIfSame = (arr = []) => { // picking array's length const { length: l } = arr; // returning true for single element and empty array if(l

60 Views
Suppose, we have an array of objects that contains information about some data storage devices like this −const drives = [ {size:"900GB", count:3}, {size:"900GB", count:100}, {size:"1200GB", count:5}, {size:"900GB", count:1} ];Notice like how the same size comes multiple times.We are required to write a function that takes in one such array and consolidate all repeated sizes into just one single array index and obviously adding up their counts.Exampleconst drives = [ {size:"900GB", count:3}, {size:"900GB", count:100}, {size:"1200GB", count:5}, {size:"900GB", count:1} ]; const groupDrives = (arr = []) => { const map = ... Read More

80 Views
Suppose, we have two arrays of literals like this −const arr1 = ['uno', 'dos', 'tres', 'cuatro']; const arr2 = ['dos', 'cuatro'];We are required to write a JavaScript function that takes in two such arrays and delete all those elements from the first array that are also included in the second array.Therefore, for these arrays, the output should look like this −const output = ['uno', 'tres'];Exampleconst arr1 = ['uno', 'dos', 'tres', 'cuatro']; const arr2 = ['dos', 'cuatro']; const findSubtraction = (arr1 = [], arr2 = []) => { let filtered = []; filtered = arr1.filter(el => { ... Read More

685 Views
Suppose, we have an object of arrays like this −const obj = { arr_a: [9, 3, 2], arr_b: [1, 5, 0], arr_c: [7, 18] };We are required to write a JavaScript function that takes in one such object of arrays. The function should construct an flattened and merged array based on this object.Therefore, the final output array should look like this −const output = [9, 3, 2, 1, 5, 0, 7, 18];Exampleconst obj = { arr_a: [9, 3, 2], arr_b: [1, 5, 0], arr_c: [7, 18] }; const objectToArray = (obj = {}) => { const res = []; for(key in obj){ const el = obj[key]; res.push(...el); }; return res; }; console.log(objectToArray(obj));OutputAnd the output in the console will be −[ 9, 3, 2, 1, 5, 0, 7, 18 ]

256 Views
We are required to write a JavaScript function that takes in two comma separated strings. The first string is the key string and the second string is the value string, the number of elements (commas) in both the strings will always be the same.Our function should construct an object based on the key and value strings and map the corresponding values to the keys.Exampleconst str1= '[atty_hourly_rate], [paralegal_hourly_rate], [advanced_deposit]'; const str2 = '250, 150, 500'; const mapStrings = (str1 = '', str2 = '') => { const keys = str1.split(', ').map( (a) => { return a.slice(1, -1); ... Read More

125 Views
Suppose, we have an array of arrays like this −const arr = [ ["Ashley", "2017-01-10", 80], ["Ashley", "2017-02-10", 75], ["Ashley", "2017-03-10", 85], ["Clara", "2017-01-10", 90], ["Clara", "2017-02-10", 82] ];We are required to write a JavaScript function that takes in one such array as the first and the only input.The function should then construct a new array of objects based on the input array. The array should contain an object for each unique subarray of the input array. (by unique, in this context, we mean the subarray that have their first element unique).Each object must ... Read More

583 Views
We are required to write a JavaScript function that takes in an array of numbers as the first argument and an upper limit and lower limit number as second and third argument respectively. Our function should filter the array and return a new array that contains elements between the range specified by the upper and lower limit (including the limits)Exampleconst array = [18, 23, 20, 17, 21, 18, 22, 19, 18, 20]; const lower = 18; const upper = 20; const filterByLimits = (arr = [], upper, lower) => { let res = []; res = arr.filter(el => { return el >= lower && el

1K+ Views
Suppose, we have an array of objects like this −const arr = [ {a: 1, b: 2}, {a: 3, b: 4}, {a: 5, b: 6} ];We are required to write a JavaScript function that takes in one such array of objects. The function should then map this array to an array of Number literals like this −const output = [1, 2, 3, 4, 5, 6];Exampleconst arr = [ {a: 1, b: 2}, {a: 3, b: 4}, {a: 5, b: 6} ]; const pushToArray = (arr = []) => { const result = arr.reduce((acc, obj) => { acc.push(obj.a); acc.push(obj.b); return acc; }, []); return result; }; console.log(pushToArray(arr));OutputAnd the output in the console will be −[ 1, 2, 3, 4, 5, 6 ]