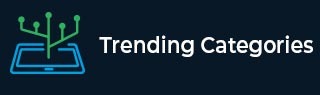
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
The Given problem is stating to count the duplicates of array elements and then aggregating objects in a new array. Understanding the Problem Problem statement is saying to identify the duplicate elements from an array and make a single array of those objects stating the counts. To solve this question we will use brute force technique with the help of for loops. What is an Aggregating Array of Objects? Aggregation in an array refers to combining down several objects in a new array as an output. We have to check if the aggregate of objects ... Read More

1K+ Views
In this problem, we have to group all the duplicate data in the array by making their subarray. After making the subarray we have to arrange them in a sorted order. This problem can be solved by searching techniques of data structures. Understanding the Problem So for solving this problem, we will use javascript’s reduce() method in two ways. The reduce method is an iterative method which uses a reducer function to iterate through all the items in the array. So by checking one by one we will keep track of all the elements and ... Read More

245 Views
The problem statement is saying that we have to find a solution for a given array and split it to form an object with a key-value pair in javascript. Understand the problem In simple terms we have to convert a given array into an object. There are several methods present in javascript to convert an array into an object. We will use and code each of them one by one. Using reduce() and split() methods In this method we will use reduce and split functions of javascript. Algorithm The algorithm here discusses ... Read More

2K+ Views
The problem stated that we have to add two properties of the JSON object and count its occurrences. For example we have name and age properties in JSON then group them in a single property and count their occurrences. What is JSON? JSON (Javascript Object Notation) is lightweight data to transfer between devices. It is human readable and writable data. JSON is in the form of key-value pairs. Keys are strings to define values. In JSON each entry is separated by a semicolon. For example - {“name” : “Peter”}, in this example name is a key and Peter ... Read More

3K+ Views
In this problem we will understand how to get a single array from multiple arrays and different ways to solve this problem. We will use three methods to solve this problem: the first method is with the help of the spread operator, second concat method and finally last method using push with the spread operator. Understanding the problem We have given two or more arrays and we have to concatenate them in a single array. There can be many arrays given and after concatenating them it should appear as a single array. Let’s understand with ... Read More

765 Views
In the given problem, we have to find out the average of every group of n elements in the array. If there are m elements in the array, then calculate the average of every group and show the result in array form. Understanding the Problem Suppose we have 6 elements in the array and make a group of 2 elements. Now calculate the average of every group individually. Now that we have their average values, we can combine them into a single array. To implement this problem we have used reduce(), push(), and slice(). These ... Read More

2K+ Views
The problem is stating that we have to add two binary strings. Binary strings are a sequence of bytes. To add them in javascript, we will first convert them to decimal and calculate the sum. After adding those decimal numbers, we will convert it again into binary strings and print the output. What are Binary Strings? Binary numbers or binary strings are sequences of bytes in base 2. It is basically a combination of 0 and 1 to represent any number. While binary addition is one of the operations of mathematics to perform on binary strings. Binary addition ... Read More

308 Views
Merging the sorted arrays together in javascript can be done using the sorting techniques available in data structures. In this problem we will be given two sorted arrays and we have to merge them. After merging them, return a single array which is also in a sorted form. For solving this problem we will use greedy approach. What are Sorted Arrays ? In javascript arrays are the collection of similar data types and we can resize the array in run time. Sorted arrays are sequential forms of items of an array. Their order should be ... Read More

96 Views
In this problem we have to validate that a given number is a power of n. If the given number is power of n then it should return the true value of it otherwise it should return false. To check this kind of validation we generally use arithmetic operators like Modulus - %, Division - /, comparison operators like less than < or greater than >. Lets understand this problem with an example. True value: N = 27 So 33 = 27 False Value: N = 28 So 33 != ... Read More

680 Views
Two strings (str1 and str2) are isomorphic if the characters in str1 can be replaced to get str2.For example −const str1 = 'abcde'; const str2 = 'eabdc';These two are an example of isomorphic stringsWe are required to write a JavaScript function that in two strings. The function should determine whether or not the two input strings are isomorphic.Exampleconst str1 = 'abcde'; const str2 = 'eabdc'; const isIsomorphic = (str1 = '', str2 = '') => { if (str1.length !== str2.length) { return false; }; for (let i = 0; i < str1.length; i++) ... Read More