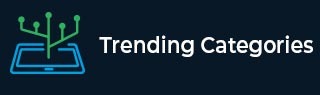
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

269 Views
In the given problem statement we are asked to find the best way to search for an element in a sorted list with the help of javascript functionalities. As we talk about sorting any list or array the binary search algorithm is the best way in data structures. What is binary search in a sorted list in JavaScript ? Let's understand the working of a list in JavaScript. A list is an object that stores multiple elements. In programming, a list is a collection of similar data elements under one roof. Since a list is also an object, it has ... Read More

213 Views
This problem says to determine if the given numbers in the array are in the form of additive sequence or not. We will check, If any two adjacent numbers in a sequence add up to the next number in the sequence, we can confirm this using a straightforward procedure. Understanding the problem To understand the above mentioned problem we need to first understand the problem in depth. If the question is broken down into subproblems, it will be easy to solve it. Consider any important procedures or stages that must be followed in order to solve the issue. So first ... Read More

428 Views
This problem says to merge the subarrays with the help of javascript concepts. We will use email data in this issue. Additionally, we will combine emails from people with the same name. Understanding the problem For solving any given statement we need to first understand the problem in depth. This problem will be broken down into subproblems. So it will be easy to solve this problem. Consider any important procedures or stages that must be followed in order to solve the issue. To solve this problem, we must organize the email addresses according to their matching names. This can ... Read More

267 Views
The Given problem is trying to find three strictly increasing numbers with consecutive or non−consecutive numbers in an array with the help of javascript. Understanding the Problem Before starting to write algorithms and programmes for the given problem first we will understand the logic behind the problem. Consider a set of array with some random numbers in it. So JavaScript allows us to loop through an array and maintain track of the smallest and second−smallest values in order to find three strictly rising numbers. In this algorithm, We will discover three strictly growing integers if a third value ... Read More

173 Views
The Given problem is stating to manipulate objects to group based on the output array object list with the help of javascript. Understanding the problem Before starting to write algorithms and programmes for the given problem first we will understand the logic behind the problem. Consider a set of objects that each represents a person and has details about that individual, such as person, age, and profession. We need to make a group of persons with the same profession. For instance, we wish to build an object whose properties each indicate a job and whose values are an array of ... Read More

2K+ Views
This problem statement is saying to sort an array of objects based on the length of the nested arrays in it. For example if the first nested array has 3 elements and the second array has 2 elements then put the second array in first place and the first array at the second index. Understanding the problem Sorting an array of objects based on the size of a nested array is the task we have given. For example, let's say we have an array of objects in which each object has a name property and a hobbies property, ... Read More

473 Views
In the given problem, we have to merge and group object properties with the help of javascript. In this article you will learn how to merge and group the same type of properties in javascript. Understanding the Problem We are required to create a javascript method that will take in one array of objects. The created function should group all the properties of all the objects that have a value for name property. For example: Input [ {name: 'Adi', age: 22, color:'Black'}, {name: 'Adi', weight: 40, height:6} , {name: 'Mack', ... Read More

3K+ Views
As the problem stated, create a program for a unique sort in javascript. Basically we have to remove duplicate elements from an array. Understand the problem In this problem statement we need to eliminate the same or duplicated item from an array. For solving this kind of problem we can use some predefined methods of javascript. In this article you will be able to learn usage of forEach(), spread operator, set() method, filter() method and indexOf() methods. Lets understand this problem with an example. Array before sorting and with duplicates [535, 646, ... Read More

987 Views
The problem statement says to merge duplicate elements and increase the count for every object present in the array and implement this program in javascript. Understanding the Problem To start coding we need to understand the basic functionality of the javascript functions. Predefined functions in JavaScript will complete half of our task. Merging and eliminating duplicates are the fundamental array optimization operations that can be performed. In this issue, we will define a count variable that will count how many entries there are in the array. We will go through each item in the array using the ... Read More

2K+ Views
Given problem says to sort the given array of objects by two properties and create a program in javascript. Understanding the problem Let's have a deep understanding of the problem statement. You will be given an array of objects with key and value. As per the problem statement we need a sorted form of that array. To implement this problem we will first sort the first column of array and then second column of array. And then again arrange it in sorted form. As we understand arrays are basic data structures in computer science. Array can also ... Read More