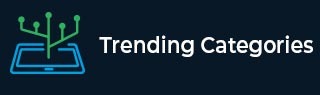
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

922 Views
In the given problem statement we are asked to find all the possible combinations from an array with the help of javascript functionalities. So for this we will be working with an empty array and add all the possible combinations into this array. Logic for The Above Problem The easiest way to find the possible combinations from an array in javascript is by using a for loop add the combination to the created new array.. To understand the logic for this implementation we will work by iterating through the input array and for each element we will create a ... Read More

267 Views
In the given problem statement we are asked to create a fibonacci like sequence with the help of javascript functionalities. In the Javascript we can solve this problem with the help of recursion or using a for loop. What is the Fibonacci Sequence ? Let's understand the working of a Fibonacci sequence in JavaScript. As we know that Fibonacci sequence is a sequence of numbers in which every number is the sum of the two forgoing numbers. The series starts from 0 and 1. The next number in the series is 1 (0 + 1), followed by 2 (1 ... Read More

248 Views
In the given problem statement we are asked to calculate the difference between the first and second element of each subarray separately and we have to return the sum of their differences with the help of javascript functionalities. In the array data structures we can define an array which can also contain subarrays. What is an Array of Subarrays in JavaScript ? Let's understand the working of a list in JavaScript. In javascript we can define array of subarray or nested array. An array of subarrays is an array which contains one or more arrays as its elements. Every array ... Read More

143 Views
In the given problem statement we are asked to calculate the average for each subarray separately and then return the sum of all the averages with the help of javascript functionalities. As we talk about average for subarrays we can use the reduce method of javascript. What is the reduce() Method in JavaScript ? Let's understand the working of a reduce function in JavaScript. In javascript the reduce method is used to reduce an array to a single value by iterating over each and every item of the array. And by applying the callback function that accumulates a value based ... Read More

125 Views
In the given problem statement we are asked to sort an array including the elements present in the subarrays with the help of javascript functionalities. As we talk about sorting any list or array the sort() and flat method is useful. What are sort(), flat() and isArray() methods in JavaScript ? Let's understand the working of sort, flat and isArray methods in JavaScript. The sort() method is basically used to sort the elements of an array and returns the sorted array. The items are sorted in dictionary order by default. But we can provide a function as an argument to ... Read More

437 Views
In the given problem statement we are asked to group the JSON object with the help of javascript functionalities. This problem can be solved with the help of a simple algorithm in javascript. What is JSON ? JSON (Javascript Object Notation) is lightweight data which can be transferred between two devices. This data can be read and written by humans. JSON objects are represented in the form of key−value pairs. Keys are strings to define values. In JSON each entry is separated by a semicolon. For example − {“Car” : “Audi”}, in this example Car is a key and Audi ... Read More

125 Views
In the given problem statement we are asked to find out maximum decreasing adjacent elements with the help of javascript functionalities. This problem can be solved with the help of a simple algorithm in javascript. Understanding the Logic Explaining the logic of the problem statement in more detail. The problem asks us to get the maximum number of decreasing adjacent items in an array or we can say we have to find the longest decreasing subarray in the input array. So for solving this problem we need to work by iterating all over the array, one item at a time ... Read More

1K+ Views
In the given problem statement we are asked to find out long factorials of a Bigint value with the help of javascript functionalities. BigInt is a data type in javascript, which is used to calculate the factorial of a large number. What is the BigInt data type in JavaScript ? Let's understand the working of a BigInt data type in JavaScript. In Javascript, there are so many data types available to define the data, one of them is BigInt. BigInt is a built−in data type in Javascript which represents integers of large size. It was introduced in ECMAScript 2020. It ... Read More

242 Views
In the given problem statement we are asked to make a group matching element in an array with the help of javascript functionalities. As we talk about grouping elements of an array we usually use the reduce method. What is the reduce() function in JavaScript ? Let's understand the working of a reduce function in JavaScript. In javascript, the built−in method reduce() is used to iterate on all the elements of an array. This method accumulates an item based on every element of the array, which basically takes two arguments. First argument is an accumulator and the second value is ... Read More

865 Views
In the given problem statement we are asked to make an array of another array’s duplicate values with the help of javascript functionalities. As we talk about duplicate values in an array means we have to find the exact elements present in the first array. What is an array in JavaScript ? Let's understand the working of an array in JavaScript. An array is an object that stores multiple elements. In programming, an array is a collection of similar data elements under one roof. Since an array is also an object, it has some properties and methods that work with ... Read More