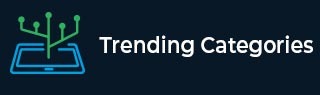
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

712 Views
In the given problem statement we have to group the given data month wise. In simple terms the data should be in sorted form after grouping them month wise. For example we have years and months given in data so it should be visible in the order from January to December. As we know that Arrays are the data structures template to store data. And we can manipulate that data as per our requirement. It can store a collection of items in a single array, and has many operations to perform like adding an element, deleting an element, ... Read More

3K+ Views
In the given problem statement we are asked to convert numbers to characters with the help of javascript functionalities. In Javascript we have some built−in functions to convert a number to its corresponding characters and also with user defined functions we can convert it. Logic for The Above Problem As we know that every programming language defines its own function to perform certain operations. So Javascript has a built−in method to convert a number into its corresponding character. These function names are 'fromCharCode' and 'charCodeAt'. With these functions we need to pass the input number and it will convert and ... Read More

13K+ Views
In the given problem statement we are asked to get value for key from nested JSON object with the help of javascript functionalities. In Javascript we can solve and access the json data object with the help of dot notation or bracket notation. Before starting coding for the given problem, let's understand what exactly is the JSON object: What is JSON and nested JSON objects? JSON’s full form is Javascript Object Notation. It is a standard text based format to show the structured data. It is basically used to transmit data in web applications, which means we can send ... Read More

568 Views
In the above statement we are asked to check if items in an array are consecutive without sorting the array with the help of javascript functionalities. We can solve this problem with some basic functionalities of javascript. Let us see how to do this! What are Consecutive Items in an Array ? Consecutive elements means every next item should be greater or smaller to the previous element in the sequence. For example we have an array [1, 2, 3, 4, 5], So here we can see that the array contains all the consecutive items as they are in increasing ... Read More

659 Views
In the given problem statement we are required to map an array to a new array with default values with the help of javascript functionalities. In Javascript we have some built−in function to map an array to a new array as per the condition and requirement. What is an array in Javascript ? An array is a collection of similar data elements under one roof. So let's understand the overall working process of arrays in JavaScript. An array is an object that contains one or more elements. Example const arr = ['A', 'B', 'C', 'D']; console.log(arr); Output [ ... Read More

435 Views
In the given problem statement we are required to convert an object to an array of objects with the help of javascript functionalities. To perform conversion of an object into an array of objects we can use Javascript’s built−in methods. What is an Array in Javascript ? An array is a collection of similar data elements under one roof. So let's understand the overall working process of arrays in JavaScript. An array is an object that contains one or more elements. So it has some properties and methods that make working with arrays easier in JavaScript. For example const ... Read More

2K+ Views
In the given problem statement we are asked to manipulate objects in an array of objects with the help of javascript functionalities. In Javascript we have some built−in functions called map(), reduce(), forEach() and filter() to manipulate the objects in an array. We can solve this problem with the help of these functions. What is the manipulating object in an array ? Let's understand the manipulation of objects in an array. Manipulation means updating an array object, deleting an array object or adding an object in an array. In Javascript, there are several methods present to do these kinds of ... Read More

3K+ Views
In the given problem statement we are asked to truncate a string with the help of javascript functionalities. We can solve this problem with the help of Javascript’s built−in functions slice() and substr(). What do you mean by truncating a string ? Let's understand the meaning of truncating a string. Truncating a string means cutting the length of a string by removing character from the beginning, end or middle part of the input string. The aim of truncating a string is to make a certain space or to make it more readable by truncating unnecessary information from the input ... Read More

820 Views
In the given problem statement we are asked to implement a function to decode a string with the help of javascript functionalities. To solve these kinds of problems we can use built−in functions of Javascript. These functions usually take encoded data as input and give output as decoded strings. What are built−in methods to decode strings in Javascript? Let's understand techniques to decode strings in javascript. In javascript, if we need to decode a string we can use different built−in methods. Some methods are: decodeURIComponent(), unescape() and atob(). We will see the working of all the methods in detail below. ... Read More

225 Views
In the given problem statement we are asked to implement backtracking for a climbing stairs practice with the help of javascript functionalities. In the data structures backtracking is popularly known to explore all the possible solutions. We can solve this problem with the help of a backtracking algorithm. What is the Backtracking Technique ? Let's understand the working of a Backtracking technique. Backtracking is a well known algorithm technique, which is basically used to solve the problem statements that include searching for all possible solutions. It is based on a depth−first strategy and it is used in combination with a ... Read More