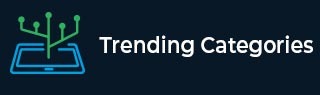
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

370 Views
Suppose, we have a nested array like this −const arr = ['zero', ['one', 'two' , 'three', ['four', ['five', 'six', ['seven']]]]];We are required to write a JavaScript function that takes in a nested array. Our function should then return a string that contains all the array elements joined by a semicolon (';')Therefore, for the above array, the output should look like −const output = 'zero;one;two;three;four;five;six;seven;';ExampleThe code for this will be −const arr = ['zero', ['one', 'two' , 'three', ['four', ['five', 'six', ['seven']]]]]; const buildString = (arr = [], res = '') => { for(let i = 0; i < arr.length; ... Read More

250 Views
Suppose, we have an array of objects like this −const arr = [ { id : "23", name : "Item 1", isActive : true}, { id : "25", name : "Item 2", isActive : false}, { id : "26", name : "Item 3", isActive : false}, { id : "30", name : "Item 4", isActive : true}, { id : "45", name : "Item 5", isActive : true} ];We are required to write a JavaScript function that takes in one such object and return an array of the value of "id" property of all those ... Read More

288 Views
We are required to write a JavaScript function that takes in an array of literal values as the first argument and a string as the second argument.Our function should sort the array alphabetically but keeping the string provided as the second argument (if it exists in the array) as the first element, irrespective of the text it contains.ExampleThe code for this will be −const arr = ["Apple", "Orange", "Grapes", "Pineapple", "None", "Dates"]; const sortKeepingConstants = (arr = [], text = '') => { const sorter = (a, b) => { return (b === text) - (a ... Read More

679 Views
Suppose, we have two child and parent JSON arrays of objects like these −const child = [{ id: 1, name: 'somename', parent: { id: 2 }, }, { id: 2, name: 'some child name', parent: { id: 4 } }]; const parent = [{ id: 1, parentName: 'The first', child: {} }, { id: 2, parentName: 'The second', child: {} }, { id: 3, parentName: 'The third', child: {} }, { id: 4, parentName: 'The ... Read More

690 Views
Suppose we have a sorted array of numbers like this where we can have consecutive numbers.const arr = [1, 2, 3, 5, 7, 8, 9, 11];We are required to write a JavaScript function that takes one such array.Our function should form a sequence for this array. The sequence should be such that for all the consecutive elements of the array, we have to just write the starting and ending numbers replacing the numbers in between with a dash (-), and keeping all other numbers unchanged.Therefore, for the above array, the output should look like −const output = '1-3, 5, 7-9, ... Read More

719 Views
Suppose, we have an array of objects like this −const arr = [ {"Date":"2014", "Amount1":90, "Amount2":800}, {"Date":"2015", "Amount1":110, "Amount2":300}, {"Date":"2016", "Amount1":3000, "Amount2":500} ];We are required to write a JavaScript function that takes in one such array and maps this array to another array that contains arrays instead of objects.Therefore, the final array should look like this −const output = [ ['2014', 90, 800], ['2015', 110, 300], ['2016', 3000, 500] ];ExampleThe code for this will be −const arr = [ {"Date":"2014", "Amount1":90, "Amount2":800}, {"Date":"2015", "Amount1":110, "Amount2":300}, {"Date":"2016", "Amount1":3000, "Amount2":500} ]; const arrify ... Read More

727 Views
Suppose, we have a comma-separated string like this −const str = "a, b, c, d , e";We are required to write a JavaScript function that takes in one such and sheds it off all the whitespaces it contains.Then our function should split the string to form an array of literals and return that array.ExampleThe code for this will be −const str = "a, b, c, d , e"; const shedAndSplit = (str = '') => { const removeSpaces = () => { let res = ''; for(let i = 0; i < str.length; ... Read More

171 Views
We are required to write a JavaScript function that takes in an array of literal values. The array might contain some repeating values inside it.Our function should remove all the repeating values keeping the first instance of repeating value in the array.ExampleThe code for this will be −const arr = [1, 5, 7, 4, 1, 4, 4, 6, 4, 5, 8, 8]; const deleteDuplicate = (arr = []) => { for(let i = 0; i < arr.length; ){ const el = arr[i]; if(i !== arr.lastIndexOf(el)){ arr.splice(i, 1); } else{ i++; }; }; }; deleteDuplicate(arr); console.log(arr);OutputAnd the output in the console will be −[ 7, 1, 6, 4, 5, 8 ]

4K+ Views
Suppose we have two arrays of objects, the first of which contains some objects with user ids and user names.The array contains objects with user ids and user addresses.The array is −const arr1 = [ {"id":"123", "name":"name 1"}, {"id":"456", "name":"name 2"} ]; const arr2 = [ {"id":"123", "address":"address 1"}, {"id":"456", "address":"address 2"} ];We are required to write a JavaScript function that takes in two such arrays and merges these two arrays to form a third array.The third array should contain the user id, name, and address object of corresponding users.ExampleThe code for this will be −const ... Read More

473 Views
We are required to write a JavaScript function that takes in an array of literal values. Our function should pick all those values from the array that appears exactly twice in the array and return a new array of those elements.ExampleThe code for this will be −const arr = [0, 1, 2, 2, 3, 3, 5]; const findAppearances = (arr, num) => { let count = 0; for(let i = 0; i < arr.length; i++){ const el = arr[i]; if(num !== el){ continue; }; ... Read More