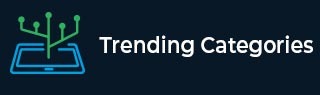
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

614 Views
We are required to write a JavaScript function that takes in an array of literal values.The array might contain some repeating values.Our function should remove all the values from the array that are repeating. We are required to remove all instances of all such elements.ExampleThe code for this will be −const arr = [1, 2, 3, 2, 4]; const removeAllInstances = (arr = []) => { filtered = arr.filter(val => { const lastIndex = arr.lastIndexOf(val); const firstIndex = arr.indexOf(val); return lastIndex === firstIndex; }); return filtered; }; console.log(removeAllInstances(arr));OutputAnd the output in the console will be −[ 1, 3, 4 ]

246 Views
Suppose, we have an array of objects that contains data about some students like this −const arr = [{ name: 'A', idNo: 1, marks: { math: 98, sci: 97, eng: 89 } }, { name: 'B', idNo: 2, marks: { math: 88, sci: 87, eng: 79 } }, { name: 'C', idNo: 3, marks: { math: 87, sci: 98, eng: 91 ... Read More

669 Views
We are required to write a JavaScript function that takes in hex color as one and only input.Our function should then find the complementary color for the color taken in as input.Here are some input and output pairs −getComplementaryColor('#142814') = '#ebd7eb'; getComplementaryColor('#ffffff') = '#000000'; getComplementaryColor('#3399ff') = '#cc6600';ExampleThe code for this will be −const str1 = '#142814'; const str2 = '#ffffff'; const str3 = '#3399ff'; const getComplementaryColor = (color = '') => { const colorPart = color.slice(1); const ind = parseInt(colorPart, 16); let iter = ((1

455 Views
Suppose, we have an array of objects like this −const arr = [ {area: 'NY', name: 'Bla', ads: true}, {area: 'DF', name: 'SFS', ads: false}, {area: 'TT', name: 'SDSD', ads: true}, {area: 'SD', name: 'Engine', ads: false}, {area: 'NSK', name: 'Toyota', ads: false}, ];We are required to write a JavaScript function that takes in one such array as the first argument and an array of string literals as the second argument.Our function should then filter the input array of objects to contain only those objects whose "area" property is included in the array of literals ... Read More

360 Views
Suppose, we have some data regarding some images in an array like this −const arr = [{ 'image': "jv2bcutaxrms4i_img.png", 'gallery_image': true }, { 'image': "abs.png", 'gallery_image': true }, { 'image': "acd.png", 'gallery_image': false }, { 'image': "jv2bcutaxrms4i_img.png", 'gallery_image': true }, { 'image': "abs.png", 'gallery_image': true }, { 'image': "acd.png", 'gallery_image': false }];We are required to write a JavaScript function that takes in one such array.Our function should remove the objects from the array that have duplicate values for the 'image' property.ExampleThe code for this will be −const arr ... Read More

638 Views
Suppose, we have an array of values like this −const arr = [ { value1:[1, 2], value2:[{type:'A'}, {type:'B'}] }, { value1:[3, 5], value2:[{type:'B'}, {type:'B'}] } ];We are required to write a JavaScript function that takes in one such array. Our function should then prepare an array where the data is grouped according to the "type" property of the objects.Therefore, for the above array, the output should look like −const output = [ {type:'A', value: [1, 2]}, {type:'B', value: [3, 5]} ];ExampleThe code ... Read More

608 Views
We are required to write a JavaScript function that takes in an array of elements. The array of elements might contain some undefined values as well.Our function should count the length of the array and the count should only contain the count of defined elements.ExampleThe code for this will be −const arr = [12, undefined, "blabla", ,true, 44]; const countDefined = (arr = []) => { let filtered; filtered = arr.filter(el => { return el !== undefined; }); const { length } = filtered; return length; }; console.log(countDefined(arr));OutputAnd the output in the console will be −4

374 Views
Suppose, we have two arrays like these −const meals = ["breakfast", "lunch", "dinner"]; const ingredients = [ ["eggs", "yogurt", "toast"], ["falafel", "mushrooms", "fries"], ["pasta", "cheese"] ];We are required to write a JavaScript function that takes in two such arrays and maps the subarrays in the second array to the corresponding strings of the first array.Therefore, the output for the above arrays should look like −const output = { "breakfast" : ["eggs", "yogurt", "toast"], "lunch": ["falafel", "mushrooms", "fries"], "dinner": ["pasta", "cheese"] };ExampleThe code for this will be −const meals = ["breakfast", "lunch", "dinner"]; const ingredients ... Read More

171 Views
We are required to write a JavaScript function that takes in two arrays of the same length.Our function should then combine corresponding elements of the arrays, to form the corresponding subarray of the output array, and then finally return the output array.If the two arrays are −const arr1 = ['a', 'b', 'c']; const arr2 = [1, 2, 3];Then the output should be −const output = [ ['a', 1], ['b', 2], ['c', 3] ];ExampleThe code for this will be −const arr1 = ['a', 'b', 'c']; const arr2 = [1, 2, 3]; const combineCorresponding = (arr1 = [], arr2 ... Read More