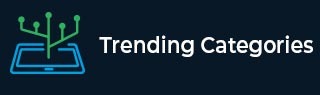
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

204 Views
We are required to write a JavaScript function that takes in a single alphabet as the only input. The function should compute the position of the that alphabet from starting and return an alphabet that’s at the same position but from the back.ExampleThe code for this will be −const alpha = 'g'; const findCounterPart = (alpha = '') => { let alphabet = 'abcdefghijklmnopqrstuvwxyz'; let firstpart = alphabet.substring(0, 13).split(''); let secondpart = alphabet.substring(13).split('').reverse(); let solution = ''; if (firstpart.indexOf(alpha) !== −1) { solution = secondpart[firstpart.indexOf(alpha)]; } else { ... Read More

163 Views
Suppose we are given the mapping a = 1, b = 2, ... z = 26, and an encoded message. We are required to write a JavaScript function that takes in the message.The function should count the number of ways it can be decoded.For example, the message '111' would give 3, since it could be decoded as 'aaa, 'ka', and 'ak'.ExampleThe code for this will be −const waysToProcess = ( message, ways = 0 ) => { if ( message.length ) { ways = waysToProcess( message.slice( 1 ,message.length), ways ); const numCurr = ... Read More

145 Views
Sometimes people repeat letters to represent extra feeling, such as "hello" −> "heeellooo", "hi" −> "hiiii". In these strings like "heeellooo", we have groups of adjacent letters that are all the same: "h", "eee", "ll", "ooo".For some given string S, a query word is stretchy if it can be made to be equal to S by any number of applications of the following extension operation: choose a group consisting of characters c, and add some number of characters c to the group so that the size of the group is 3 or more.For example, starting with "hello", we could do ... Read More

1K+ Views
We are required to write a JavaScript function that takes in a number between the range [0, 25], both inclusive.Return valueThe function should return the corresponding alphabets for that number.ExampleThe code for this will be −const num = 15; const indexToAlpha = (num = 1) => { // ASCII value of first character const A = 'A'.charCodeAt(0); let numberToCharacter = number => { return String.fromCharCode(A + number); }; return numberToCharacter(num); }; console.log(indexToAlpha(num));OutputAnd the output in the console will be −P

217 Views
We are required to write a JavaScript function that takes in an array of Integers. Making use of recursion and the push and pop methods of the array, the function should sort the array inplace.ExampleThe code for this will be −const stack = [−3, 14, 18, −5, 30]; const sortStack = (stack = []) => { if (stack.length > 0) { let t = stack.pop(); sortStack(stack); sortedInsert(stack, t); }; } const sortedInsert = (stack, e) => { if (stack.length == 0 || e > stack[stack.length − 1]) { stack.push(e); } else { let x = stack.pop(); sortedInsert(stack, e); stack.push(x); } } sortStack(stack); console.log(stack);OutputAnd the output in the console will be −[ −5, −3, 14, 18, 30 ]

1K+ Views
We are required to make a calculator with the RPN (reverse polish notation) input method using Stacks in JavaScript.Consider the following input array −const arr = [1, 5, '+', 6, 3, '-', '/', 7, '*'];Process −1 is an operand, push to Stack.5 is an operand, push to Stack.'+' is an operator, pop 1 and 5, calculate them and push result to Stack.6 is an operand, push to Stack.3 is an operand, push to Stack.'−' is an operator, pop 6 and 3, subtract them and push result to Stack.'/' is an operator, pop 6 and 3, divided them and push result ... Read More

156 Views
Heap Sort is basically a comparison-based sorting algorithm. It can be thought of as an improved selection sort − like that algorithm, it divides its input into a sorted and an unsorted region, and it interactively shrinks the unsorted region by extracting the target (largest or smallest) element and moving that to the sorted region.ExampleThe code for this will be −const constructHeap = (arr, ind) => { let left = 2 * ind + 1; let right = 2 * ind + 2; let max = ind; if (left < len && arr[left] > arr[max]) { ... Read More

295 Views
We are required to write a JavaScript function that takes in a string that represents a binary code. The function should return the alphabetical representation of the string.For example −If the binary input string is −const str = '1001000 1100101 1101100 1101100 1101111 100000 1010111 1101111 1110010 1101100 1100100';Then the output should be −const output = 'Hello World';ExampleThe code for this will be −const str = '1001000 1100101 1101100 1101100 1101111 100000 1010111 1101111 1110010 1101100 1100100'; const binaryToString = (binary = '') => { let strArr = binary.split(' '); const str = strArr.map(part => { return String.fromCharCode(parseInt(part, 2)); }).join(''); return str; }; console.log(binaryToString(str));OutputAnd the output in the console will be −Hello World

6K+ Views
We are required to write a JavaScript function that takes in a string as the only input. The function should construct and return the binary representation of the input string.For example −If the input string is −const str = 'Hello World';Then the output should be −const output = '1001000 1100101 1101100 1101100 1101111 100000 1010111 1101111 1110010 1101100 1100100';ExampleThe code for this will be −const str = 'Hello World'; const textToBinary = (str = '') => { let res = ''; res = str.split('').map(char => { return char.charCodeAt(0).toString(2); }).join(' '); return res; }; console.log(textToBinary('Hello World'));OutputAnd the output in the console will be −1001000 1100101 1101100 1101100 1101111 100000 1010111 1101111 1110010 1101100 1100100

471 Views
We are required to write a JavaScript function that takes in an array of Numbers as the first argument and a number, say n, as the second argument.Our function should then pick the n greatest numbers from the array and return a new array consisting of those numbers.ExampleThe code for this will be −const arr = [3, 4, 12, 1, 0, 5, 22, 20, 18, 30, 52]; const pickGreatest = (arr = [], num = 1) => { if(num > arr.length){ return []; }; const sorter = (a, b) => b - a; ... Read More