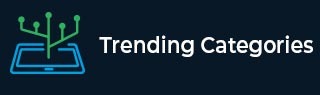
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

97 Views
We are required to write a JavaScript function that takes in an array of literal values. Our function should then return the highest occurrence of an array value, and if there's an equal occurrence, we should return the first selected value of the equal occurrences.const arr = ['25', '50', 'a', 'a', 'b', 'c']In this case, we should return 'a'const arr = ['75', '100', 'a', 'b', 'b', 'a']In this case, I should also get 'a'ExampleThe code for this will be −const arr = ['25', '50', 'a', 'a', 'b', 'c']; const arr1 = ['75', '100', 'a', 'b', 'b', 'a']; const getMostFrequentValue = ... Read More

417 Views
We are required to write a JavaScript function that takes in a string. Our function is supposed to count the number of alphabets (uppercase or lowercase) in the array.For example − If the input string is −const str = 'this is a string!';Then the output should be −13ExampleThe code for this will be −const str = 'this is a string!'; const isAlpha = char => { const legend = 'abcdefghijklmnopqrstuvwxyz'; return legend.includes(char); }; const countAlphabets = (str = '') => { let count = 0; for(let i = 0; i < str.length; i++){ ... Read More

944 Views
Suppose, we have an array of objects like this −const arr = [ { id: 1, score: 1, isCut: false, dnf: false }, { id: 2, score: 2, isCut: false, dnf: false }, { id: 3, score: 3, isCut: false, dnf: false }, { id: 4, score: 4, isCut: false, dnf: false }, { id: 5, score: 5, isCut: true, dnf: true }, { id: 6, score: 6, isCut: true, dnf: false }, { id: 7, score: 7, isCut: true, dnf: false }, { id: 8, score: 8, isCut: true, dnf: false ... Read More

842 Views
Suppose, we have an array of arrays like this −const arr = [ [ ['firstName', 'Joe'], ['lastName', 'Blow'], ['age', 42], ['role', 'clerk'], [ ['firstName', 'Mary'], ['lastName', 'Jenkins'], ['age', 36], ['role', 'manager'] ] ] ];We are required to write a JavaScript function that takes in one such array. The function should construct an array of objects based on this array of arrays.The output array should ... Read More

1K+ Views
We are required to write a JavaScript function that takes in an array of elements.Our function should check whether or not the array contains an integer value.We should return true if it does false otherwise.ExampleThe code for this will be −const arr = ["123", "", "21345", "90"]; const findInteger = (arr = []) => { const isInteger = num => { return typeof num === 'number'; }; const el = arr.find(isInteger); return !!el; }; console.log(findInteger(arr));OutputAnd the output in the console will be −false

108 Views
We are required to write a JavaScript function that takes in any number of arrays of literals. The function should compute and return an array of cartesian product of all the elements from the arrays separating them with a dash('−').ExampleThe code for this will be −const arr1= [ 'a', 'b', 'c', 'd' ]; const arr2= [ '1', '2', '3' ]; const arr3= [ 'x', 'y', ]; const dotCartesian = (...arrs) => { const res = arrs.reduce((acc, val) => { let ret = []; acc.map(obj => { val.map(obj_1 => { ... Read More

725 Views
We are required to write a JavaScript function that takes in a number as the first input and a maximum number as the second input.The function should generate four random numbers, which when summed should equal the number provided to function as the first input and neither of those four numbers should exceed the number given as the second input.For example − If the arguments to the function are −const n = 10; const max = 4;Then, const output = [3, 2, 3, 2];is a valid combination.Note that repetition of numbers is allowed.ExampleThe code for this will be −const total ... Read More

311 Views
Suppose, we n separate array of single characters. We are required to write a JavaScript function that takes in all those arrays.The function should build all such possible strings that −contains exactly one letter from each arraymust not contain any repeating character (as the arrays might contain common elements)For the purpose of this problem, we will consider these three arrays, but we will write our function such that it works well with variable number of arrays −const arr1 = [a, b ,c, d ]; const arr2 = [e, f ,g ,a]; const arr3 = [m, n, o, g, k];ExampleThe code ... Read More

113 Views
We are required to write a JavaScript function that takes in an array of strings as the first argument and a string as the second argument.The function should check whether the string specified by second argument can be formed by combining the strings of the array in any possible way.For example − If the input array is −const arr = ["for", "car", "keys", "forth"];And the string is −const str = "forthcarkeys";Then the output should be true, because the string is a combination of elements at 3, 1 and 2 indexes of the array.ExampleThe code for this will be −const arr ... Read More

264 Views
We are required to write a JavaScript function that takes in an array of integers. Our function is required find the subset of non−adjacent elements with the maximum sum.And finally, the function should calculate and return the sum of that subset.For example −If the input array is −const arr = [3, 5, 7, 8, 10];Then the output should be 20 because the non−adjacent subset of numbers will be 3, 7 and 10.ExampleThe code for this will be −const arr = [3, 5, 7, 8, 10]; const maxSubsetSum = (arr = []) => { let min = −Infinity const ... Read More