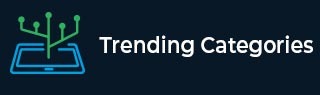
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

176 Views
Suppose, we have an array of objects like this −const arr = [ { region: "Africa", fruit: "Orange", user: "Gary" }, { region: "Africa", fruit: "Apple", user: "Steve" }, { region: "Europe", fruit: "Orange", user: "John" }, { region: "Europe", fruit: "Apple", user: "bob" }, { region: "Asia", fruit: "Orange", user: "Ian" }, { region: "Asia", fruit: "Apple", user: "Angelo" }, { region: "Africa", fruit: "Orange", user: "Gary" } ];We are required to write a JavaScript function that takes in one such array. The function should prepare a new array of objects that ... Read More

576 Views
Suppose, we have a JSON array with key/value pair objects like this −const arr = [{ "key": "name", "value": "john" }, { "key": "number", "value": "1234" }, { "key": "price", "value": [{ "item": [{ "item": [{ "key": "quantity", "value": "20" }, { "key": "price", "value": "200" }] }] ... Read More

738 Views
We have data with a one to many relationships in the same array. The organization is established by level. An element's parent is always one level higher than itself and is referenced by parentId.We are required to get a multi-level array from this array. The elements with the highest level would be the main array, with their children as subarray.If the input array is given by −const arr = [ { _id: 100, level: 3, parentId: null, }, { _id: 101, level: ... Read More

3K+ Views
We have a complex json file that we have to handle with JavaScript to make it hierarchical, in order to later build a tree.Every entry of the JSON array has −id − a unique id, parentId − the id of the parent node (which is 0 if the node is a root of the tree)level − the level of depth in the treeThe JSON data is already "ordered", means that an entry will have above itself a parent node or brother node, and under itself a child node or a brother node.The input array is −const arr = [ ... Read More

639 Views
Suppose, we have the following array of arrays −const arr = [ [ ['dog', 'Harry'], ['age', 2] ], [ ['dog', 'Roger'], ['age', 5] ] ];We are required to write a JavaScript function that takes in one such nested array. The function should then prepare an object based on the array.The object for the above array should look like −const output = [ {dog: 'Harry', age: 2}, {dog: 'Roger', age: 5} ];ExampleThe code for this will be −const arr = [ [ ['dog', 'Harry'], ['age', ... Read More

330 Views
We are required to write a JavaScript function that takes in an array of literal values. The function should then count the frequency of each element of the input array and prepare a new array on that basis.For example − If the input array is −const arr = [5, 5, 5, 2, 2, 2, 2, 2, 9, 4];Then the output should be −const output = [ [5, 3], [2, 5], [9, 1], [4, 1] ];ExampleThe code for this will be −const arr = [5, 5, 5, 2, 2, 2, 2, 2, 9, 4]; const frequencyArray = (arr = []) => { const res = []; arr.forEach(el => { if (!this[el]) { this[el] = [el, 0]; res.push(this[el]) }; this[el][1] ++ }, {}); return res; }; console.log(frequencyArray(arr));OutputAnd the output in the console will be −[ [ 5, 3 ], [ 2, 5 ], [ 9, 1 ], [ 4, 1 ] ]

763 Views
Suppose, we have a reference to an object −let test = {};This object will potentially (but not immediately) have nested objects, something like −test = {level1: {level2: {level3: "level3"}}};We are required to write a JavaScript function that takes in one such object as the first argument and then any number of key strings as the arguments after.The function should determine whether or not the nested combination depicted by key strings exist in the object.ExampleThe code for this will be −const checkNested = function(obj = {}){ const args = Array.prototype.slice.call(arguments, 1); for (let i = 0; i < args.length; ... Read More

542 Views
We are required to write a JavaScript function that takes in a string. The function should count the number of vowels present in the string.The function should prepare an object mapping the count of each vowel against them.ExampleThe code for this will be −const str = 'this is an example string'; const vowelCount = (str = '') => { const splitString=str.split(''); const obj={}; const vowels="aeiou"; splitString.forEach((letter)=>{ if(vowels.indexOf(letter.toLowerCase()) !== -1){ if(letter in obj){ obj[letter]++; }else{ ... Read More

340 Views
We are required to write a JavaScript function that takes two arrays, say arr1 and arr2. Our function should return a sorted array in lexicographical order of the strings of arr1 which are substrings of strings of arr2.ExampleThe code for this will be −const lexicographicalSort = (arr1 = [], arr2 = []) => { let i, j; const res = []; outer: for (j = 0; j < arr1.length; j++) { for (i = 0; i < arr2.length; i++) { if (arr2[i].includes(arr1[j])) { res.push(arr1[j]); ... Read More

263 Views
We are required to write a JavaScript function that takes in an array of strings. The function should find all the substring and superstring combinations that exist in the array and return an array of those elements.For example − If the array is −const arr = ["abc", "abcd", "abcde", "xyz"];Then the output should be −const output = ["abc", "abcd", "abcde"];because the first two are the substring of last.ExampleThe code for this will be −const arr = ["abc", "abcd", "abcde", "xyz"]; const findStringCombinations = (arr = []) => { let i, j, res = {}; for (i = 0; ... Read More