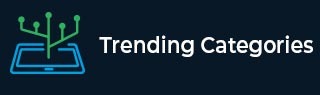
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

325 Views
We have one object like this −const obj1 = { name: " ", email: " " };and another like this −const obj2 = { name: ['x'], email: ['y']};We are required to write a JavaScript function that takes in two such objects. and want the output to be the union like this −const output = { name: {" ", [x]}, email: {" ", [y]} };ExampleThe code for this will be −const obj1 = { name: " ", email: " " }; const obj2 = { name: ['x'], email: ['y']}; const objectUnion = (obj1 = {}, obj2 = {}) => { ... Read More

79 Views
Suppose, we have two JavaScript objects defined like this −const a = { a: 1, af: function() { console.log(this.a) }, }; const b = { b: 2, bf: function() { console.log(this.b) }, };We are required to write a JavaScript function that takes in two such objects. Create another object which will get the properties of a and b, like this −const output = { a: 1, af: function() { console.log(this.a) }, b: 2, bf: function() { console.log(this.b) }, }Note that a and b need to stay the same.ExampleThe code for this will ... Read More

212 Views
Let’s say, we have an array of numbers and we added elements to it. You need to devise a simple way to remove a specific element from an array.The following is what we are looking for −array.remove(number);We have to use core JavaScript. Frameworks are not allowed.ExampleThe code for this will be −const arr = [2, 5, 9, 1, 5, 8, 5]; const removeInstances = function(el){ const { length } = this; for(let i = 0; i < this.length; ){ if(el !== this[i]){ i++; continue; ... Read More

257 Views
We are required to depict the ways in which we can access the current timestamp in JavaScript in −---seconds---millisecondsJavaScript works with the number of milliseconds since the epoch whereas most other languages work with the seconds.This will give you a Unix timestamp (in seconds) −const date = new Date(); const unix = Math.round(+date / 1000); console.log(unix);This will give you the milliseconds since the epoch (not Unix timestamp) −const date = new Date(); const milliseconds = date.getTime(); console.log(milliseconds);

75 Views
We are required to write a JavaScript function that takes in an array of Numbers as the first argument and a number, say n, as the second argument.Our function should calculate and return the greatest possible product of n numbers from the array.ExampleThe code for this will be −const getHighestProduct = (arr, num) => { let prod = 1; const sorter = (a, b) => a - b; arr.sort(sorter); if (num > arr.length || num & 2 && arr[arr.length - 1] < 0) { return; }; if (num % 2) { ... Read More

962 Views
Suppose, we have an array of nested objects like this −const arr = [{ id: 1, legs:[{ carrierName:'Pegasus' }] }, { id: 2, legs:[{ carrierName: 'SunExpress' }, { carrierName: 'SunExpress' }] }, { id: 3, legs:[{ carrierName: 'Pegasus' }, { carrierName: 'SunExpress' }] }];We are required to write a JavaScript function that takes one such array as the first argument and a search query string as the second argument.Our function should filter ... Read More

2K+ Views
Suppose, we have the following array in JavaScript −const arr = [{ "code": "2", "name": "PENDING" }, { "code": "2.2", "name": "PENDING CHILDREN" }, { "code": "2.2.01.01", "name": "PENDING CHILDREN CHILDREN" }, { "code": "2.2.01.02", "name": "PENDING CHILDREN CHILDREN02" }, { "code": "1", "name": "ACTIVE" }, { "code": "1.1", "name": "ACTIVE CHILDREN" }, { "code": "1.1.01", "name": "ACTIVE CHILDREN CHILDREN" }];We are required to write a JavaScript function that takes in one such array. The function should build a tree structure from this array based on ... Read More

707 Views
Suppose, we have an array of objects that contains data about some fruits and vegetables like this −const arr = [ {food: 'apple', type: 'fruit'}, {food: 'potato', type: 'vegetable'}, {food: 'banana', type: 'fruit'}, ];We are required to write a JavaScript function that takes in one such array.Our function should then group the array objects based on the "type" property of the objects.It means that all the "fruit" type objects are grouped together and the "vegetable' type grouped together separately.ExampleThe code for this will be −const arr = [ {food: 'apple', type: 'fruit'}, {food: 'potato', type: ... Read More

491 Views
We are required to write a JavaScript function that takes in an array of strings. Our function should iterate through the array and find and return the longest string from the array.Our function should do this without changing the content of the input array.ExampleThe code for this will be −const arr = ["aaaa", "aa", "aa", "aaaaa", "acc", "aaaaaaaa"]; const findLargest = (arr = []) => { if(!arr?.length){ return ''; }; let res = ''; res = arr.reduce((acc, val) => { return acc.length >= val.length ? acc : val; }); return res; }; console.log(findLargest(arr));OutputAnd the output in the console will be −aaaaaaaa

210 Views
We are required to write a JavaScript function that takes in an array of Numbers.The array might contain some repeating / duplicate entries within it. Our function should add all the duplicate entries and return the new array thus formed.ExampleThe code for this will be −const arr = [20, 20, 20, 10, 10, 5, 1]; const sumIdentical = (arr = []) => { let map = {}; for (let i = 0; i < arr.length; i++) { let el = arr[i]; map[el] = map[el] ? map[el] + 1 : 1; }; const res = []; for (let count in map) { res.push(map[count] * count); }; return res; }; console.log(sumIdentical(arr));OutputAnd the output in the console will be −[ 1, 5, 20, 60 ]