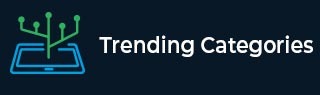
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

299 Views
We are required to write a JavaScript function that takes in a number, say n. The function should count the letters in the number names from 1 to n.For example − If n = 5;Then the numbers are one, two, three, four, five.And the total number of letters are 19, so the output should be 19.Exampleconst sumUpto = (num = 1) => { let sum = 0; const lenHundred = 7; const lenThousand = 8; const lenPlaceOnes = [0,3,3,5,4,4,3,5,5,4]; const lenPlaceTens = [0,3,6,6,5,5,5,7,6,6]; for (let i = 1; i 100 && i % 100 != 0) { sum += 3; } } return sum; } console.log(sumUpto(12)); console.log(sumUpto(5)); console.log(sumUpto(122));OutputThis will produce the following output −51 19 1280

763 Views
Suppose, we have a reference array of phrases like this −const reference = ["your", "majesty", "they", "are", "ready"];And we are required to join some of the elements of above array based on another array so if another array is this −const another = ["your", "they are"];The result would be like −result = ["your", "majesty", "they are", "ready"];Here, we compared the elements in both the arrays, we joined the elements of the first array if they existed together in the second array.We are required to write a JavaScript function that takes in two such arrays and returns a new joined array.Exampleconst ... Read More

1K+ Views
We are required to write a JavaScript String function that takes in a search string can loosely checks for the search string in the string it is used with.The function should take this criterion into consideration: It should loop through the search query letters and check if they occur in the same order in the string.For example −('a haystack with a needle').fuzzySearch('hay sucks'); // false ('a haystack with a needle').fuzzySearch('sack hand'); // trueExampleconst fuzzySearch = function (query) { const str = this.toLowerCase(); let i = 0, n = -1, l; query = query.toLowerCase(); for (; l ... Read More

153 Views
We are required to write a JavaScript function that takes in an array of Integers. The function should return the length of the longest decreasing subsequence from the array.For example −If the input array is −const arr = [5, 2, 5, 4, 3, 2, 4, 6, 7];Then the output should be −const output = 4;because the longest decreasing subsequence (of consecutive words) is [5, 4, 3, 2];Exampleconst arr = [5, 2, 5, 4, 3, 2, 4, 6, 7]; const decreasingSequence = (arr = []) => { let longest = []; let curr = []; const setDefault = ... Read More

272 Views
We are required to write a JavaScript function that in any number of arguments (all of Number type).The function should calculate all possible sums of addition and subtraction.For example − If the arguments are 1, 2, 3Then all possible combinations are −1 + 2 + 3 1 - 2 - 3 1 + 2 - 3 1 - 2 + 3Finally, the function should the sum that is closest to 0. In this case, that answer would just be 0.Exampleconst findSmallestPositive = (...arr) => { let set = new Set([Math.abs(arr[0])]); for (let i = 1; i < ... Read More

222 Views
We are required to write a JavaScript function that takes in a read only array of n + 1 integers between 1 and n.The function should find one number that repeats in linear time and using at most O(n) space.For example If the input array is −const arr = [3 4 1 4 1];Then the output should be −const output = 1;If there are multiple possible answers ( like above ), we should output any one. If there is no duplicate, we should output -1.Exampleconst arr = [3, 4, 1, 4, 1]; const findRepeatedNumber = (arr = []) => { ... Read More

697 Views
We are required to write a JavaScript function that takes in an array of Numbers. The function should apply the algorithm of quicksort to sort the array either in increasing or decreasing order.QuickSort AlgorithmQuicksort follows the below steps −Step 1 − Make any element as the pivot (preferably first or last, but any element can be the pivot)Step 2 − Partition the array on the basis of pivotStep 3 − Apply a quick sort on the left partition recursivelyStep 4 − Apply a quick sort on the right partition recursivelyThe average and best case time complexity of QuickSort are O(nlogn) ... Read More

134 Views
Suppose, we have an array that contains some demo credit card numbers like this −const arr = ['4916-2600-1804-0530', '4779-252888-3972', '4252-278893-7978', '4556-4242-9283-2260'];We have been tasked with creating a function that takes in this array. The function must return the credit card number with the greatest sum of digits.If two credit card numbers have the same sum, then the last credit card number should be returned by the function.ExampleThe code for this will be −const arr = ['4916-2600-1804-0530', '4779-252888-3972', '4252-278893-7978', '4556-4242-9283-2260']; const findGreatestNumber = (arr) => { let n, i = 0, sums; sums = []; while (i < ... Read More

1K+ Views
Suppose, we have the following array of objects −const arr = [ {"2015":11259750.05}, {"2016":14129456.9} ];We are required to write a JavaScript function that takes in one such array. The function should prepare an array of arrays based on the input array.Therefore, the output for the above array should look like −const output = [ [2015,11259750.05], [2016,14129456.9] ];ExampleThe code for this will be −const arr = [ {"2015":11259750.05}, {"2016":14129456.9} ]; const mapToArray = (arr = []) => { const res = []; arr.forEach(function(obj,index){ const key= Object.keys(obj)[0]; const value = parseInt(key, 10); res.push([value, obj[key]]); }); return res; }; console.log(mapToArray(arr));OutputAnd the output in the console will be −[ [ 2015, 11259750.05 ], [ 2016, 14129456.9 ] ]

488 Views
We are required to write a JavaScript program that provides users an input to fill in a number.And upon filling when the user clicks the button, we should display the sum of all the digits of the number.ExampleThe code for this will be −JavaScript Code −function myFunc() { var num = document.getElementById('digits').value; var tot = 0; num.split('').forEach( function (x) { tot = tot + parseInt(x,10); }); document.getElementById('output').innerHTML = tot; }HTML Code − Submit OutputAnd the output will be &miuns;After clicking “Submit” −