- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript

4K+ Views
In JavaScript, the functions wrapped with parenthesis are called “Immediately Invoked Function Expressions" or "Self Executing Functions.The purpose of wrapping is to namespace and control the visibility of member functions. It wraps code inside a function scope and decrease clashing with other libraries. This is what we call Immediately Invoked Function Expression (IIFE) or Self Executing Anonymous Function.Here’s the syntax −(function() { // code })();As you can see above, the following pair of parentheses converts the code inside the parentheses into an expression −function(){...}In addition, the next pair, i.e. the second pair of parentheses continues the operation. It calls ... Read More

796 Views
JavaScript ClosuresIn JavaScript, all functions work like closures. A closure is a function, which uses the scope in which it was declared when invoked. It is not the scope in which it was invoked.Here’s an exampleLive Demo JavaScriptClosures varp = 20; functiona(){ var p = 40; b(function(){ alert(p); ... Read More

121K+ Views
To change the font size in HTML, use the style attribute. The style attribute specifies an inline style for an element. The attribute is used with the HTML tag, with the CSS property font-size. HTML5 do not support the tag, so the CSS style is used to add font size.Just keep in mind, the usage of style attribute overrides any style set globally. It will override any style set in the HTML tag or external style sheet.ExampleYou can try to run the following code to change the font size in an HTML page, Live Demo ... Read More
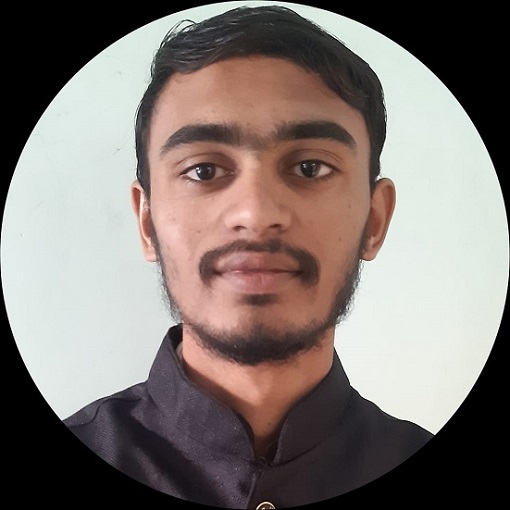
507 Views
This article will teach you how to create a JavaScript recursive function-a function that calls itself-using the recursion method. The process of recursion involves calling itself. Recursive functions are those that call themselves repeatedly. A condition to cease calling itself is always included in recursive functions. Otherwise, it will continue to call itself. Recursive functions are typically used to divide a large issue into smaller ones. Recursive functions are frequently found in data structures like binary trees, graphs, and algorithms like binary search and quick-sort.A recursive function is not immediately clear or simple to comprehend. You will read and comprehend ... Read More

19K+ Views
To create a link to send email, use tag, with href attribute. The mail to link is added inside the tag. To add a subject, you need to add ? and then include the subject. All this comes inside the tag.Just keep in mind to add the email address where you want to receive the email in the mail to link. Also, the spaces between words for the subject shouldn’t be space, instead include %20. This is to ensure the browser displays the text properly.ExampleYou can try to run the following code to create a link to ... Read More
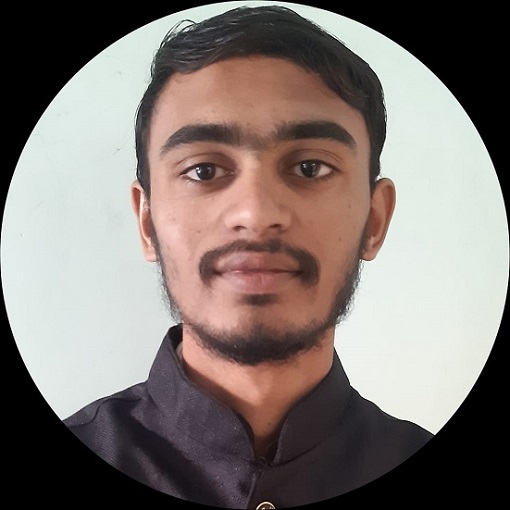
187 Views
In this tutorial, let us discuss the function scope and context in JavaScript. Functions are the building blocks of Javascript. Therefore, JavaScript is a functional programming language. Scope The 'scope' is the code space where we can define and use a variable in a function. Scopes are of four types. Global scope Global 'scope' is the default scope. A variable with global 'scope' is accessible throughout the program. Browser closing removes a global variable. Window objects can access var variables wherein they can't access the let variables. Functional or local 'scope' A function variable is accessible only with the function. ... Read More

151 Views
A function is a group of reusable code which can be called anywhere in your program. This eliminates the need of writing the same code repeatedly. It helps programmers in writing modular codes.The most common way to define a function in JavaScript is by using the function keyword, followed by a unique function name, a list of parameters (that might be empty), and a statement block surrounded by curly braces.Here’s an example − To call a function somewhere later in the script, you would simply need to write the name of that function as shown in the following ... Read More

88 Views
defaultThis came to handle function parameters with ease. Easily set Default parameters to allow initializing formal parameters with default values. This is possible only if no value or undefined is passed. Let’s see an exampleExampleLive Demo // default is set to 1 function inc(val1, inc = 1) { return val1 + inc; } document.write(inc(10, 10)); document.write(""); document.write(inc(10)); restES6 ... Read More
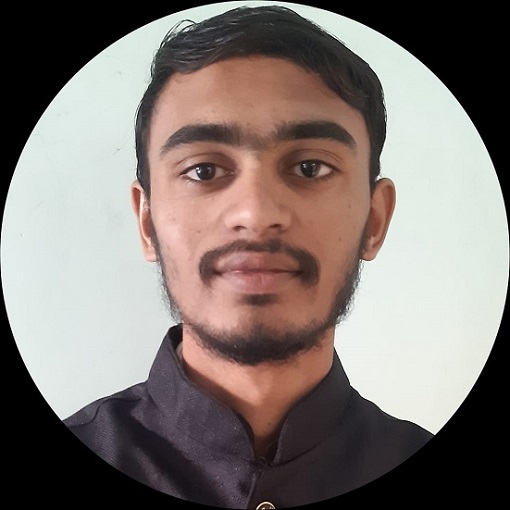
17K+ Views
In this tutorial, we will learn to concatenate the multiple string variables in JavaScript. It’s many times needed that we need to concatenate two strings in a single variable and use it. The simple meaning of concatenation is to merge two or multiple strings. Also, string concatenation is useful to insert some substring in the parent string. For example, while developing the application, as a programmer, you need to update the string by adding some other extra string to it. Users can split the string first and insert the substring using the concatenation operation. There are various methods to merge ... Read More

3K+ Views
No, you cannot create a dialog box with “yes” or “no”. A confirmation dialog box in JavaScript has “Ok” and “Cancel” button.To create a dialog with “yes” or “nor”, use a custom dialog box.ExampleLive Demo function functionConfirm(msg, myYes, myNo) { var confirmBox = $("#confirm"); confirmBox.find(".message").text(msg); confirmBox.find(".yes, .no").unbind().click(function() { confirmBox.hide(); }); ... Read More