- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6685 Articles for Javascript

144 Views
Rotation of the array elements means moving the elements of the given array to either the left or right side by some number of specific positions. We will assume the array in the cyclic form and rotate the elements of the edges to the other end. Block swap algorithm for array rotation means to rotate the elements of the array by a given number but not using the rotation but the swapping technique. We will implement both recursive as well as iterative approaches. Input The given array is [ 1, 2, 3, 4, 5, 6, 7]. The number of rotations ... Read More

210 Views
A singly linked list is a linear data structure that is stored in a non-contiguous way in the memory and each block is connected by holding the address of the next block also known as a node. A palindrome can be explained as a set of characters, digits, etc, and it reads the same from both the front and backside. We will be given a singly linked list and have to find whether the values stored at the nodes are equal from both the front and backside. Input 1 -> 2 -> 3 -> 3 -> 2 -> 1 ... Read More

309 Views
A symmetric matrix is a special case of a matrix where both the matrix and the transpose of the matrix are the same. A matrix is a set of integers or numbers stored in a rectangular form that is equivalent to the 2D array and the transpose of the matrix is also a matrix that we can get by replacing all the rows with columns. We will be given a matrix and have to print whether it is a symmetric matrix or not. Input Mat = [[1, 2, 3], [2, 3, 8], [3, 8, ... Read More

107 Views
In mathematics, a matrix is a set of integers or numbers stored in a rectangular form which is equivalent to the 2D array in programming or JavaScript programming. A sparse matrix is a special type of matrix in which the number of zeros is strictly more than the total number of elements or numbers present in the given matrix. We will be given a matrix and we have to find whether the current matrix is a sparse matrix or not. Input Mat = [ [1, 0, 0], [0, 3, 4], [8, 0, ... Read More

95 Views
An idempotent matrix is a square matrix that has the same number of rows and columns and when we multiply the matrix by itself the result will be equal to the same matrix. We will be given a matrix and we have to find that whether it is an idempotent matrix or not. Mathematically − If the given matrix ix M, then of M to be an idempotent matrix is should follow the property − M*M = M Multiplication of Matrix Multiplication of a matrix with another matrix produces another matrix and if the given matrix is the square ... Read More
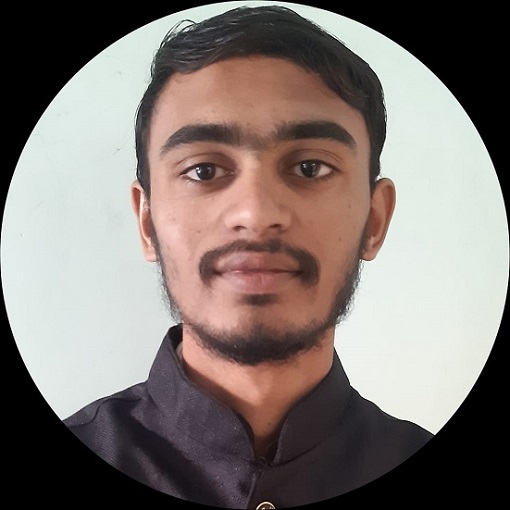
91 Views
Problem Statement − We have given a number. We need to rotate the number and need to find the total number of rotations divisible by 8. Here, we will learn two different approaches to counting rotations divisible by 8. Rotate the Number and Check if Rotation is Divisible by 8 The first approach is to rotate numbers and get every possible rotation one by one. Also, check if rotation is divisible by 8. If yes, add 1 to the count. Syntax Users can follow the syntax below to count rotations divisible by 8 by rotating the numbers. for ( ) ... Read More
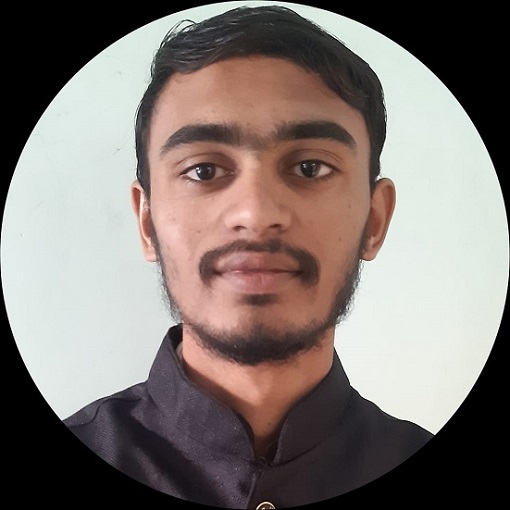
98 Views
In this tutorial, we will learn to count the total number of rotations divisible by 4 for the given number. Problem statement − We have given a number value. We need to rotate numbers in clockwise or anti-clockwise directions and count the total number of rotations divisible by 4. Here, we will learn two different approaches to counting rotations divisible by 4. Rotate the Number and Check if it is Divisible by 4 In this approach, we will convert the number to a string first. We can make n rotations for the string of length n. We will remove the ... Read More
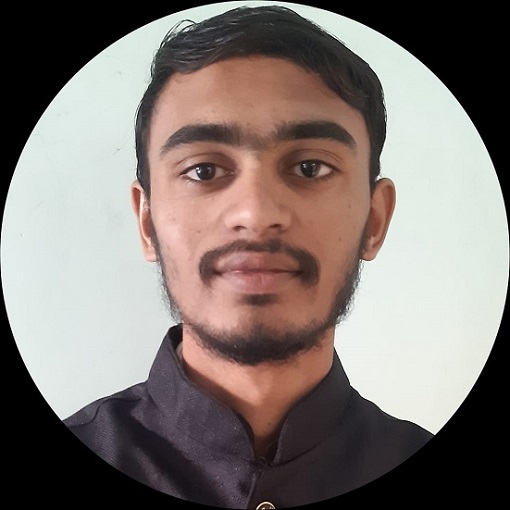
105 Views
In this tutorial, we will learn to count inversions of size three in a given array. Problem statement − We have given an array of length n containing the different numeric entries. We need to find the total number of pairs of numbers of size 3 such that arr[i] > arr[j] > arr[k], where I < j < k. Here, we will learn the brute force approach first and after that, we will optimize its time and space complexity. Using the Brute Force Approach In the brute force approach, we will use three nested for loops to find a count ... Read More
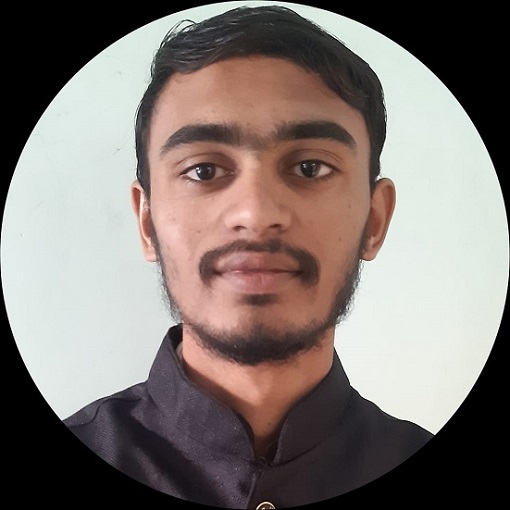
2K+ Views
The given number is a power of 2 if the number is generated using multiplying 2’s only. In this tutorial, we will learn to check whether a given number is a power of 2. Here, we will look at 5 different approaches to check whether a given number is a power of 2. Using the Math.pow() Method In JavaScript, the number can contain 64 bits most. So, we can use the for loop and Math.pow() method to find the 1 to 64 power of the 2. For loop, we can compare the ith power of 2 with the number. If ... Read More
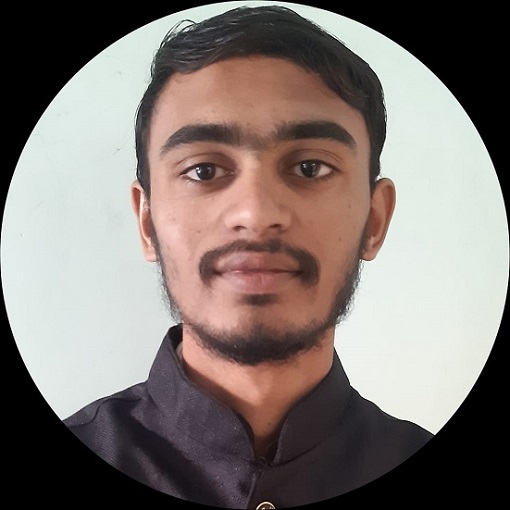
3K+ Views
The object is the most important data type of JavaScript. Even everything is an object in JavaScript. For example, Array is an object, and Number, String, and Boolean can also be an object. Sometimes, developers require to insert properties into the object based on some condition. For example, we have an object of the person, and we only require adding the driving licence property if a person is 18 years old. Here, we will learn different approaches to adding a member to an object using JavaScript conditionally. Using the Spread Operator to add a Member to an Object Conditionally The ... Read More