- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 8895 Articles for Front End Technology

11K+ Views
In this tutorial, we will learn about the different methods or ways of finding the largest number contained inside a JavaScript array. An array is a collection of different values. We will define an array of numbers in which we will search for the largest number. There are three ways of finding the largest number in a JavaScript array that is listed below − Traversing the Whole Array Using the reduce() Method Using the Math.max() and apply() methods Traversing the Whole Array This is the easiest or the most naive approach to find the largest number in the ... Read More
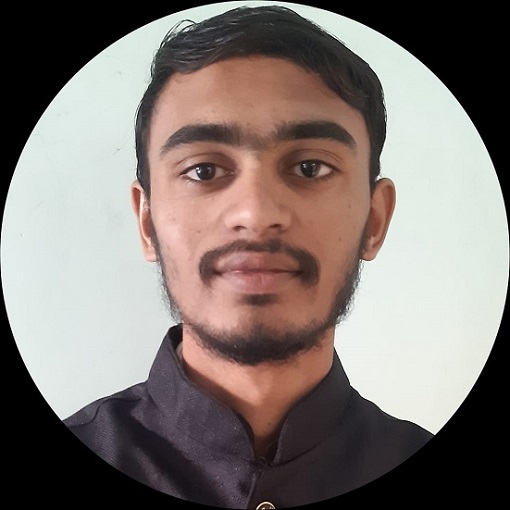
40K+ Views
In this tutorial, let’s see how to determine whether a number is odd or even using JavaScript. The first step is understanding the logic. What are even numbers? Even numbers will be exactly divisible by 2. The remainder will be zero. What are odd numbers? Odd numbers will carry a remainder when divided by 2. Using the if-else Condition and Modulo Operator "if" will check a condition, and the if block code will be executed if the condition is true. If the condition is false, else block code will be executed. The modulo operator in JavaScript returns the remainder. Syntax ... Read More

750 Views
In this tutorial, we will discuss how to round up a number in JavaScript. There is a property in JavaScript under ‘Math’ named “ceil” with the help of this property we can easily round up a number in JavaScript. Ceil() is a mathematical function that computes an integer x to greater than or equal to x. If x has the decimal value zero or is a simple integer then it returns x, and if the number is a decimal number (x.yz, here yz is a non-zero number) then it returns x+1. Syntax var x = A.B var value = Math.ceil(x); ... Read More

178 Views
NaN is a JavaScript property, which is Not-a-Number value. This shows it is not a legal number. Here’s the syntax −SyntaxNumber.NaNTo find out whether a number is NaN, use the Number.isNaN() or isNan() method. Here’s an example to check −ExampleLive Demo Check function display() { var a = ""; a = a + isNaN(434) + ": 434"; a = a + isNaN(-23.1) + ": -23.1"; a = a + isNaN('Hello') + ": 'Hello'"; a = a + isNaN(NaN) + ": NaN"; a = a + isNaN('') + ": ''"; a = a + isNaN(0) + ": 0"; a = a + isNaN(false) + ": false"; document.getElementById("test").innerHTML = a; }

14K+ Views
We use border-collapse property to create a collapsed border in HTML. The border-collapse is a CSS property used to set the table borders should collapse into a single border or be separated with its own border in HTML. Border-collapse property has four values: separate, collapse, initial, inherit. With the value collapse If you pass collapse as a value of the border-collapse Property, the borders of the table are simply collapsed into a single border. Following is the syntax to create a collapsed border in HTML. border-collapse: collapse; Example 1 In the example given below we are trying to ... Read More

1K+ Views
To get numbers of days in a month, use the getDate() method and get the days.ExampleYou can try to run the following code to get a number of days in a month −Live Demo var days = function(month,year) { return new Date(year, month, 0).getDate(); }; document.write("Days in July: "+days(7, 2012)); // July month document.write("Days in September: "+days(9, 2012)); // September Month OutputDays in July: 31 Days in September: 30

31K+ Views
To create table border in HTML, the border attribute was used. But the introduction of HTML5, deprecated the border tag. Create table border using the CSS property border. Set table border as well as border for and .ExampleYou can try to run the following code to create a border in the table in HTML. We’re using tag here: table, th, td { border: 1px solid black; } Employee Details Name Amit Sachin Age 27 34 Output
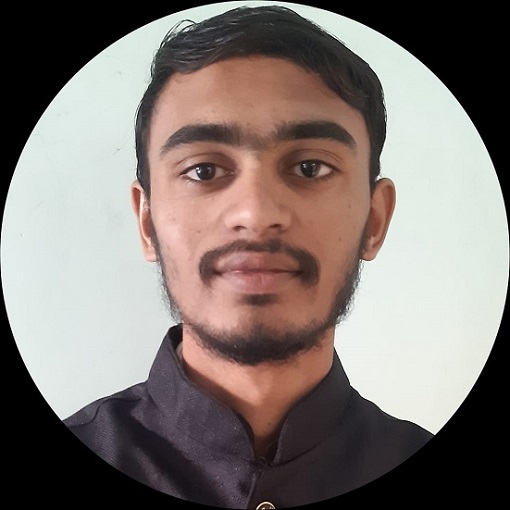
998 Views
This tutorial teaches us to round down a number in JavaScript. The meaning of the round-down number is “returning the integer which is equal to or less than the current float number”.For example, if we round down the number 9.99, we get 9 as an output, and in the same way, for 8.01 we get 8 as an output. In simple terms, if you represent your current number in the number line, you must return the nearest integer that resides on the left side of the current number.In this tutorial, we will take a look at three different approaches to ... Read More

91K+ Views
This tutorial will teach us how to format a number with two decimals using JavaScript. Formatting a number means rounding the number up to a specified decimal place. In JavaScript, we can apply many methods to perform the formatting. Some of them are listed as follows − The toFixed() Method Math.round() Method Math.floor() Method Math.ceil() Method The toFixed() Method The toFixed() method formats a number with a specific number of digits to the right of the decimal. it returns a string representation of the number that does not use exponential notation and has the exact number of digits after ... Read More
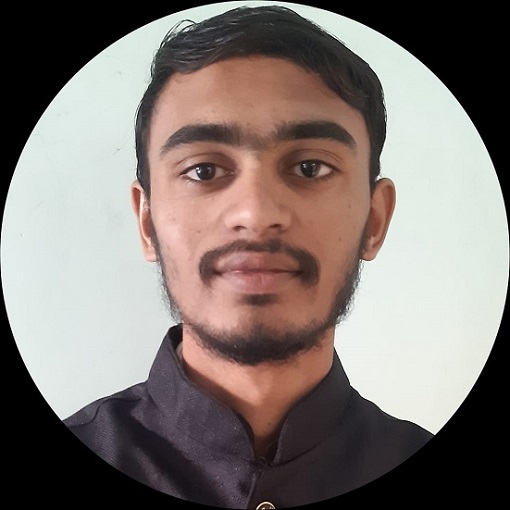
2K+ Views
In this tutorial, we will learn how to perform integer division and get the remainder in JavaScript. The division operator (/) returns the quotient of its operands, where the dividend is on the left, and the divisor is on the right. When another splits one operand, the remainder operator (%) returns the residual. It always takes the dividend sign. In the operation x% y, x is known as the dividend, and y is known as the divisor. If one of the operands is NaN, x is Infinity, or y is 0, the operation returns NaN. Otherwise, the dividend x is ... Read More