- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 1862 Articles for Data Structure

119 Views
Introduction In graph theory, it is a very important task to figure out if a graph built from an array and meeting certain conditions has a cycle or not. A graph is an imaginary way to show how things are linked together. It is used in a lot of places, like computer networks and social networks. This article talks about the conditions for graph construction, the BFS and DFS algorithm, and a step by step guide how loops in a undirected graph is identified. Array Representation of a Graph An array-based method in graph theory stores vertices and edges in ... Read More
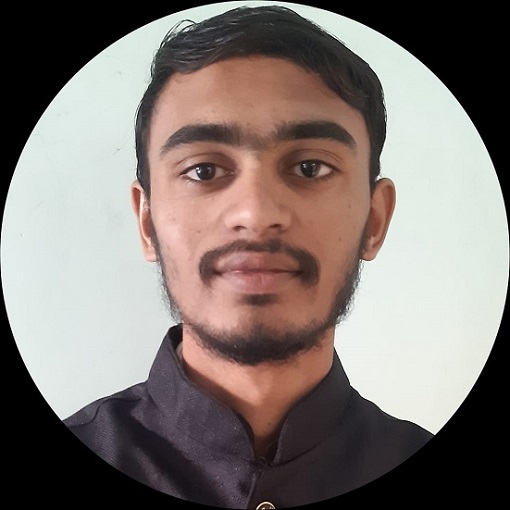
76 Views
In this problem, we need to sort the given binary string in decreasing order by removing only non-adjacent elements. To solve the problem, we require to remove all zeros which are placed before ones in the binary string. If we find two consecutive ones after two consecutive zeros at any position in the string, it means we can’t sort the string in decreasing order. Otherwise, we can sort it out in each case. Problem statement − We have given binary string str with a length equal to N. We need to check whether we can sort the given string in ... Read More

483 Views
Introduction A Successor Graph is a model of a directed graph in which each node stores a list of the nodes that come after it. Successor graphs are better than an adjacency matrix or list because they speed up access to outgoing edges. This makes them perfect for algorithms that need quick access to successor vertices. This choice of design works well for graphs with a lot of points but not many edges Representation of Successor Graphs using Adjacency Matrix Successor graphs store only the direct successors of each vertex, reducing memory usage and speeding up edge insertion and deletion ... Read More

390 Views
Introduction Depth-First Search (DFS) is a graph traversal method that looks at vertices and edges by starting at a certain node and going as far as possible down each branch before going back.It looks at the "depth" of the graph, starting with the node that is the deepest and then going back to look at other lines. Recursion or a stack can be used to make DFS work. It can be used to find paths, find cycles in graphs and vectors, and do exhaustive searches. Understanding the Matrix Structure In data analysis, a matrix is a two-dimensional array. Matrix data ... Read More
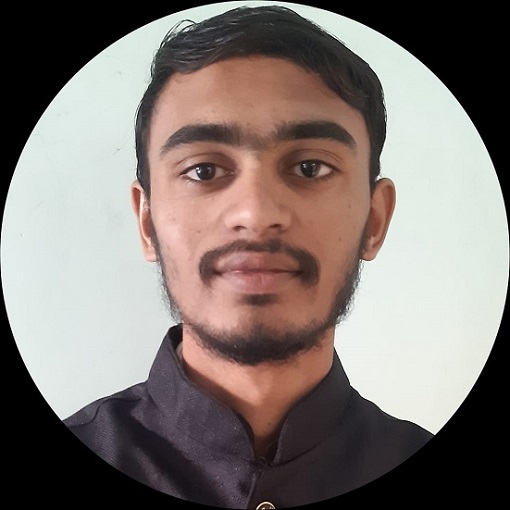
134 Views
In this problem, we need to convert the first binary string to the second binary string by flipping the prefix of the first string. To get the minimum prefix flip, we require to iterate through the end of the string, and if we find mismatched characters in both strings, we need to flip the prefix of the first string. Problem statement − We have given two different binary strings called first and second. The length of both binary strings is equal, which is N. We need to convert the first string into the second string by flipping the prefixes of ... Read More

980 Views
Disjoint set information structure, too known as the Union-Find algorithm, could be an essential concept in computer science that gives an effective way to solve issues related to apportioning and network. It is especially valuable in solving issues including sets of components and determining their connections. In this article, we are going to investigate the language structure, algorithm, and two distinctive approaches to executing the disjoint set information structure in C++. We will also provide fully executable code examples to illustrate these approaches. Syntax Before diving into the algorithm, let's familiarize ourselves with the syntax used in the following code ... Read More

83 Views
A palindromic string is a string that is equal to its reverse string. We are given a string that contains ‘0’, ‘1’, and ‘2’ and an array Q of length N and each index of the given array indicates a range in the form of pairs. We have to find the minimum number of characters that are needed to replace in the given range such that none of the palindromic substrings remains in that range. Sample Example Input1: string s: “01001020002”, int Q = {{0, 4}, {2, 5}, {5, 10}}; Output: 1 1 3 Explanation For the range ... Read More

119 Views
Two binary strings str1 and str2 of the same length are given and we have to maximize a given function value by choosing the substrings from the given strings of equal length. The given function is such that − fun(str1, str2) = (len(substring))/(2^xor(sub1, sub2)). Here, len(substring) is the length of the first substring while xor(sub1, sub2) is the xor of the given substrings as they are binary strings so it is possible. Sample Examples Input1: string str1 = 10110 & string str2 = 11101 Output: 3 Explanation We can choose a lot of different sets of strings ... Read More

341 Views
A string str is given and we can swap only adjacent characters to make the string reverse. We have to find the number of minimum moves required to make the string reverse just by swapping the adjacent characters. We will implement two approaches to find the required solution with the explanation and the implementation of the code. Sample Examples Input1: string str1 = “shkej” Output: 10 Explanation First, we will take the last character to the first position which will take 4 swappings, and then the string will be “jshke”. Then we will move ‘e’ to the second ... Read More

248 Views
Prefixes are the substring from the given string that starts from the zeroth index and can go up to length 1 to the size of the string. Similarly, the suffix is the substring of any length 1 to the size of the string and ends at the last index. We will be given two strings and have to create the first string by using any number of prefixes and suffixes of the second string in any way. If it is not possible to create the given string from the given methods then we will return -1. Sample Examples Input 1: ... Read More