- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 1862 Articles for Data Structure
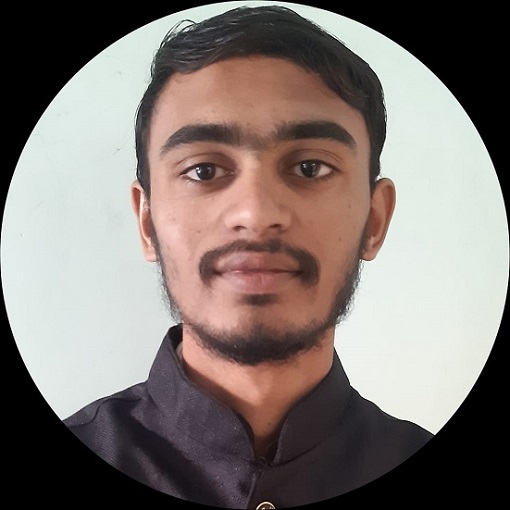
40 Views
In this problem, we need to perform the given operations with each string prefix. In the end, we need to count the frequency of each character. We can follow the greedy approach to solve the problem. We need to take each prefix of length K and update its characters according to the given conditions. We can use the map to count the frequency of characters in the final string. Problem statement − We have given the strings tr containing the N lowercase alphabetical characters. Also, we have given the mapping list, which contains total 26 elements. Each element is mapped ... Read More
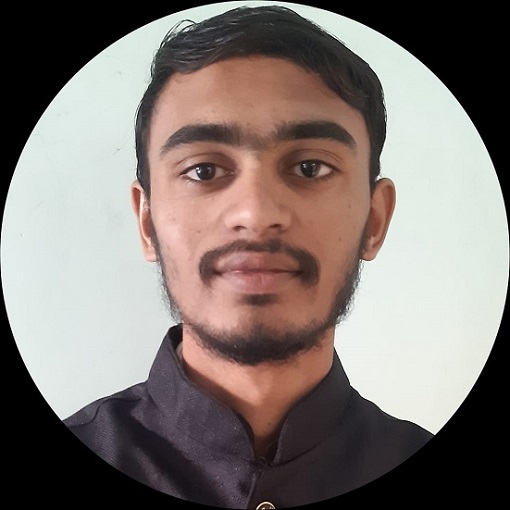
148 Views
In this problem, we will check if the linked list contains the string as a subsequence. We can iterate the list and string together to check whether a string is present as a subsequence in the linked list. Problem statement − We have given a string of size N. Also, we have given a linked list of the dynamic length containing the alphabetical characters. We need to check whether the linked list contains the string as a subsequence. Sample examples Input 'e' -> 'h' -> 'e' -> 'k' -> 'h' -> 'e' -> 'l' ->'o' -> 'l' -> 'o' ... Read More
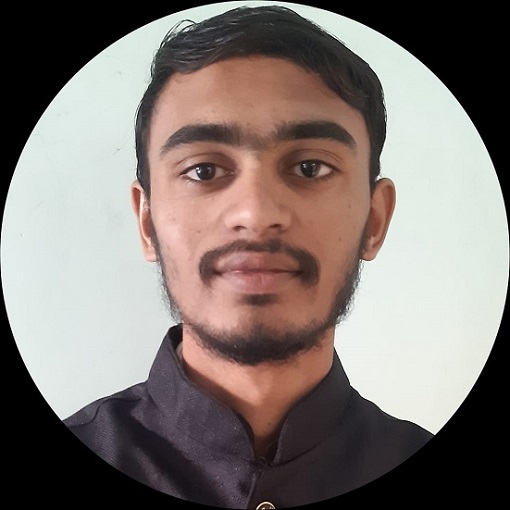
54 Views
In this problem, we need to generate the Lyndon words of the length n using the given characters. The Lyndon words are words such that any of its rotations is strictly larger than itself in the lexicographical order. Here are examples of Lyndon words. 01 − The rotations of ‘01’ is ‘10’, which is always strictly greater than ‘01’. 012 − The rotations of ‘012’ is ‘120’ and ‘210’, which is strictly greater than ‘012’. Problem statement − We have given an array s[] containing numeric characters. Also, we have given n representing the length ... Read More

3K+ Views
In this article, we will discuss different approaches to calculate the number of positive integers which have no repeated digits between a given range Low to high. The first approach is a brute force approach which iterates over all the numbers in the range and check if they contain repeated digits. In our second approach, we calculated the desired count using prefix array while in our last approach we used the concept of memorization in dynamic programming to get the desired result. Problem Statement: We are given two numbers low and high and we have to find the count of ... Read More

261 Views
In this article, we will discuss what is Tomohiko Sakamoto’s algorithm and how this algorithm is used to identify which day of the week does the given date occurs. There are multiple algorithms to know the day of the week but this algorithm is the most powerful one. This algorithm finds the day of the month on which the date occurs in least possible time and least space complexity. Problem statement − We are given a date as per Georgian calendar and our task is to find out which day of the week occurs on the given date using ... Read More

1K+ Views
In this article, we will discuss a few recursive practice problems with their detailed solutions. Let us first understand what recursion is and how it works: Recursion − Recursion is a programming technique in which a function or method calls itself multiple times in order to solve a problem. The function breaks down the problem into smaller sub-problems and solves them until it reaches a base case. The base case is a stopping condition that makes sure that the function stops calling itself and returns a result in finite time. Recursion is a powerful technique for solving complex ... Read More

210 Views
In this article, we will discuss the code solution to swap every alternate bit in a given number and return the resultant number. We will use the concept of bit manipulation in order to solve the problem in constant time without using any loops. Problem statement − We are given a number n, we have to swap the pair of bits that are adjacent to each other. In other words, we have to swap every odd placed bit with its adjacent even placed bit. Constrain: While solving the problem, we have to keep In mind that we cannot use ... Read More

53 Views
In this article, we will study different approaches to calculate the sum of the series- (n^2 - 1^2) + 2(n^2 - 2^2) + …. n(n^2 - n^2). In the first approach, we will calculate the series sum one by one for each i in the range 1 to n and keep adding it to the final sum. In the second approach, we will derive a mathematical formula to calculate the sum of the given series which will result in the reduced time complexity of the program from O(n) to O(1). Problem statement − We are given a number “n “and ... Read More
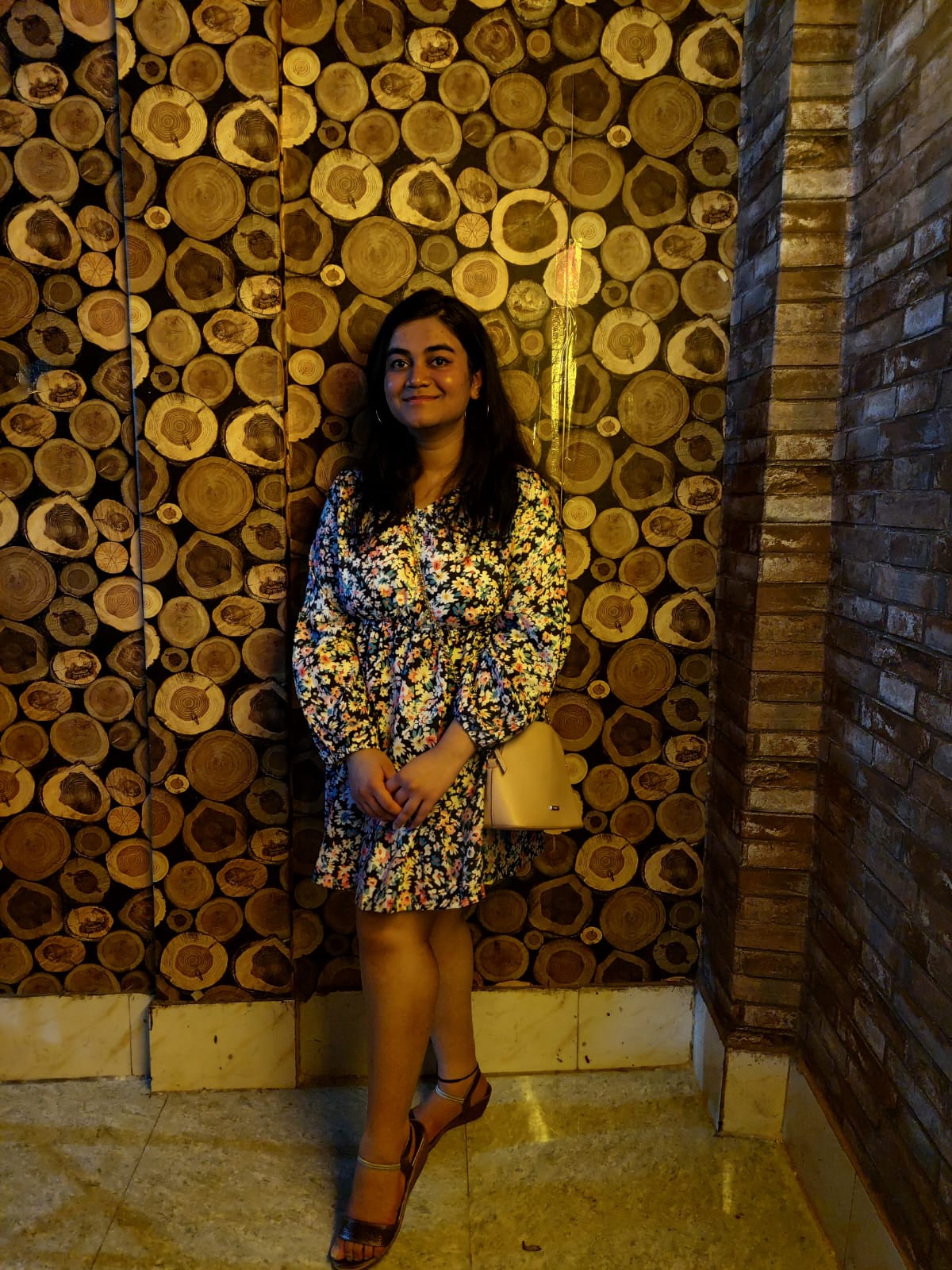
1K+ Views
ASCII Values ASCII (American Standard Code for Information Interchange) is the most common character encoding format for text data in computers and on the internet. In standard ASCII-encoded data, there are unique values for 256 alphabetic, numeric, or special additional characters and control codes. Problem Statement Now, in this problem, we need to find the sorted string as per ASCII values of the characters in increasing order, where the string will be the input given to us by the user. Let us see how we should proceed to solve this problem. Let’s try to understand this problem with the help ... Read More
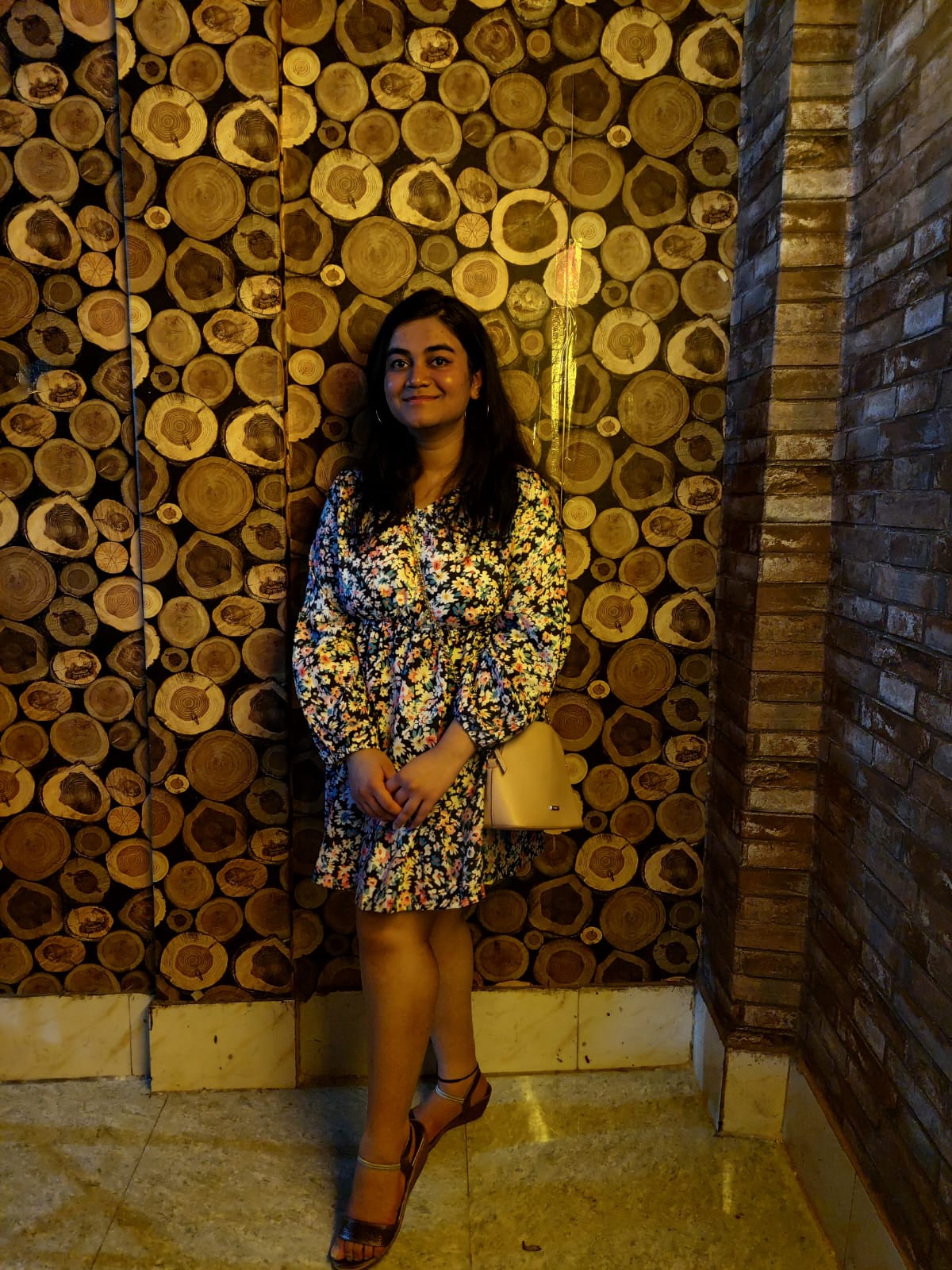
94 Views
Hamming distance between two strings of equal length is the number of all the positions at which a different value exists at the corresponding position of the other string. We can understand this with an example given below − S = “ramanisgoing” T = “dishaisgoing” Here, 5 is the hamming distance between two strings S and T as raman and disha are two words that make a difference in the strings to become equal. Problem Statement However, in this problem, we need to find the hamming distance between two strings that contain binary numbers only. One string would be ... Read More