- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 1862 Articles for Data Structure
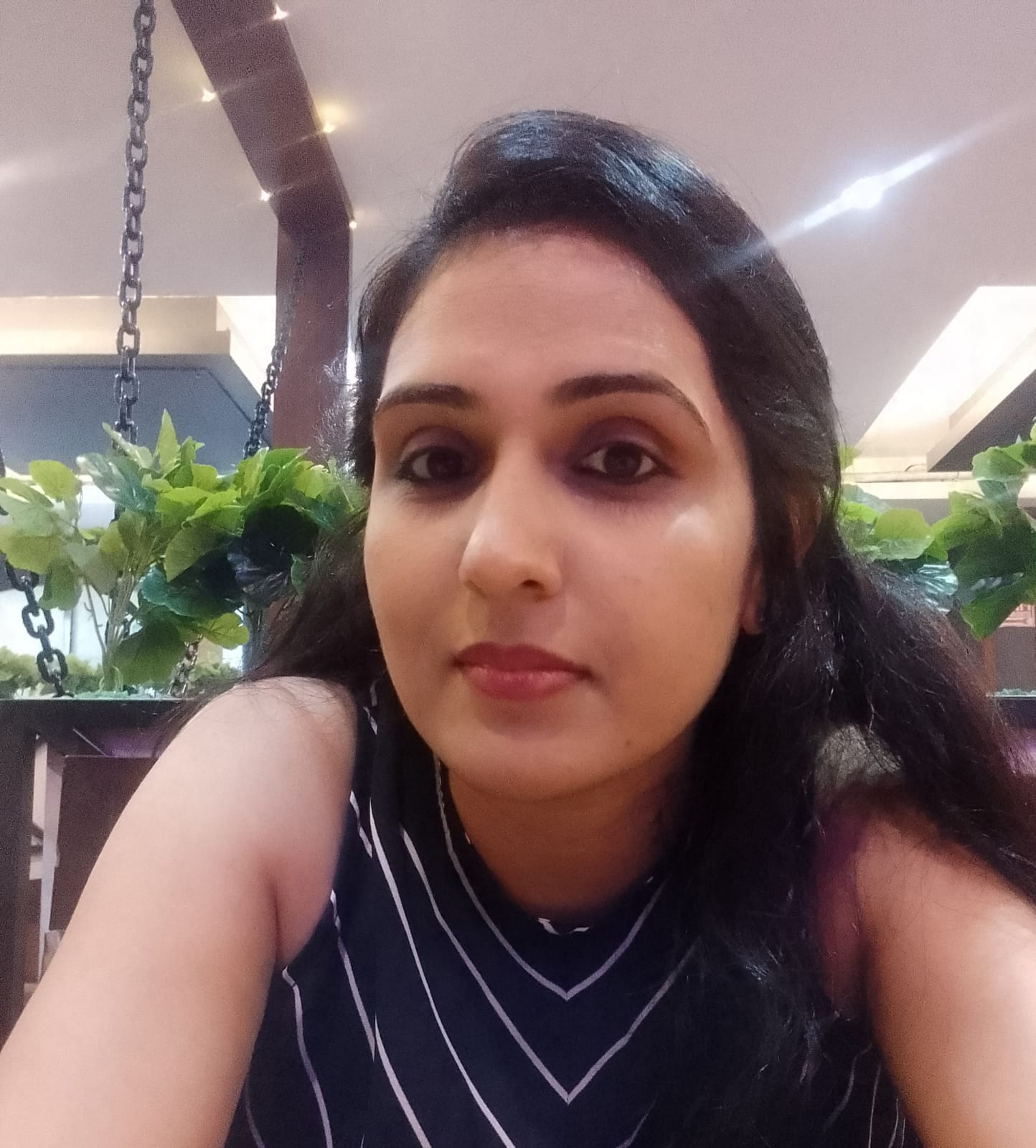
150 Views
Introduction In this tutorial, we discuss the problem of range queries to find the element having Maximum Digit Sum in C++. To solve the problem, take an array of elements and some queries. The queries denote the indices of the array and using those queries find the array element with the maximum digit sum. Maximum digit sum is the highest sum of two digit numbers (addition of ones and tens place digits) or one digit number. For example: 12, its sum is 3 (1 + 2). In this tutorial, by using the queries find such a number that has a ... Read More
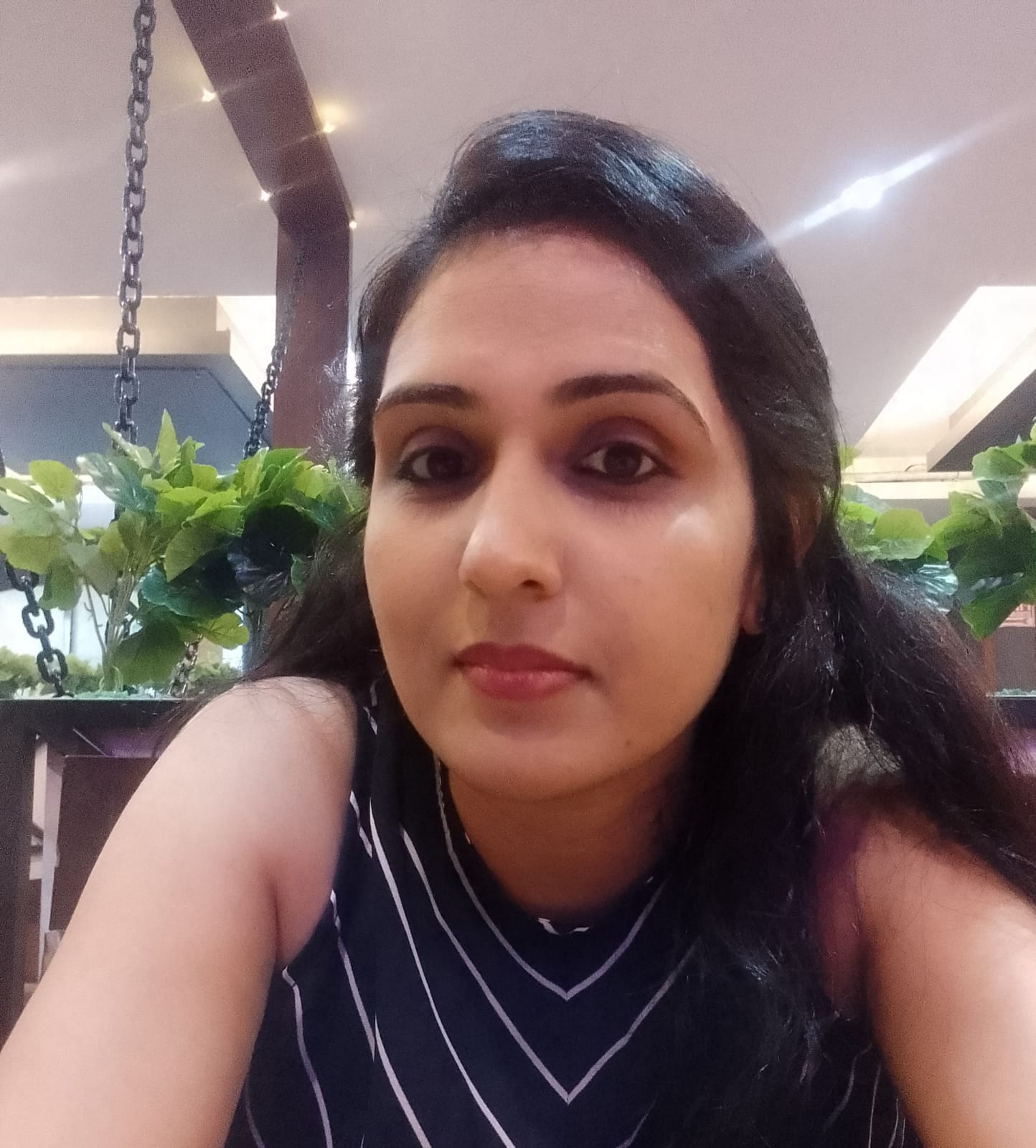
55 Views
Introduction In this tutorial, we implement an approach in C++ to resolve queries for the count of even digit sum elements in the given range. We use a segment tree. To solve this task, we consider an array of elements with queries defining the range of the subarray. In that subarray count the even digit sum elements. Predefine the element array and the queries to resolve the problem using the Segment tree. What is a segment tree? A segment tree is a binary data structure that stores array interval or segment information. It efficiently solves range or segment query problems. ... Read More
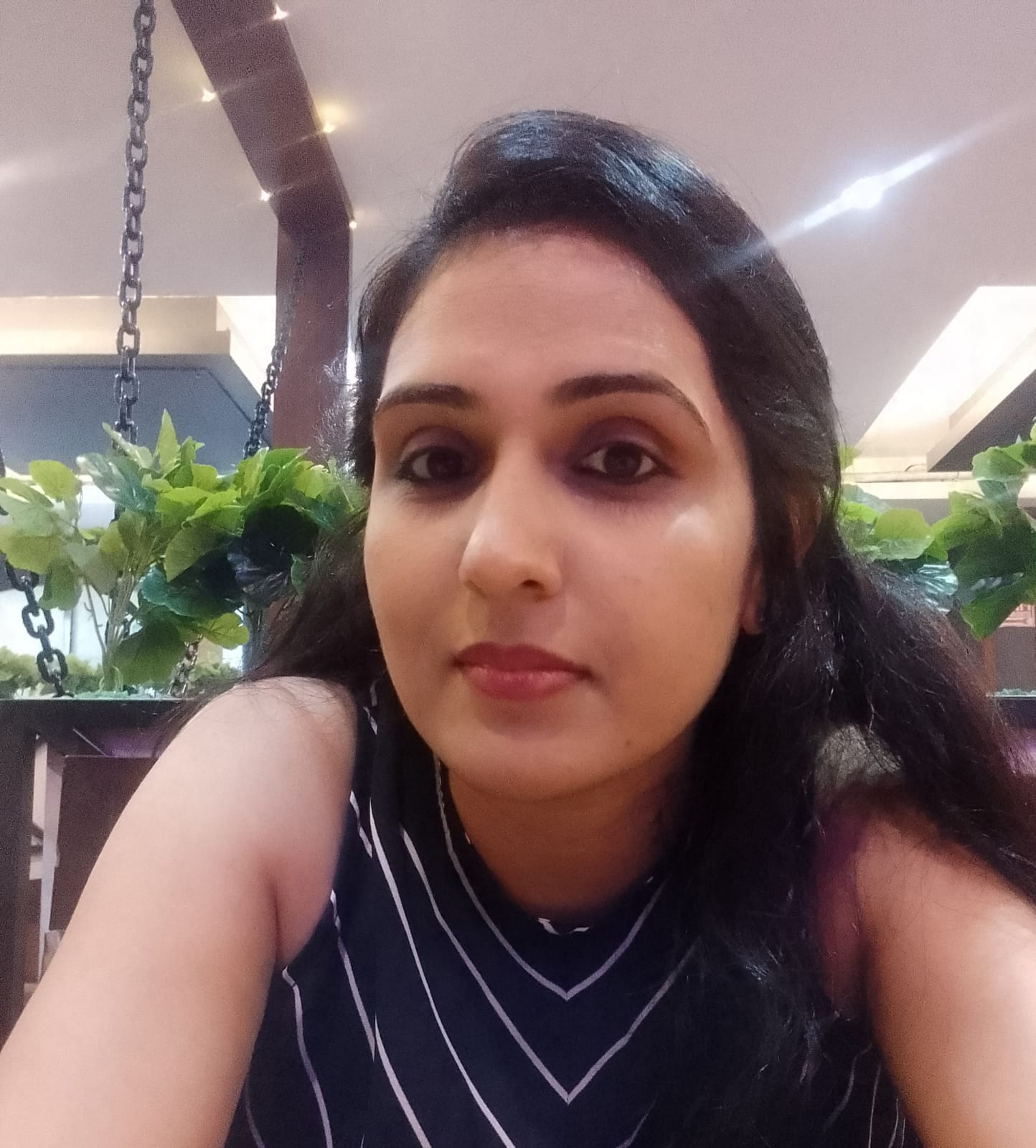
136 Views
Introduction This tutorial implements an approach to printing Strings In Reverse Dictionary Order Using Trie. Trie is a data structure with a tree representation. It is in ordered form and provides an efficient approach for string retrieval. It has nodes and edges like a tree data structure. To solve the task, initialize an array and arrange strings in reverse dictionary order using the trie. Each alphabet is used as a node in the tree. Duplicate array elements are printed only once. Demonstration 1 arr[] = {“hello", "world", "open", "ai", "c++", "programming"”} Output world programming open hello c++ ai ... Read More
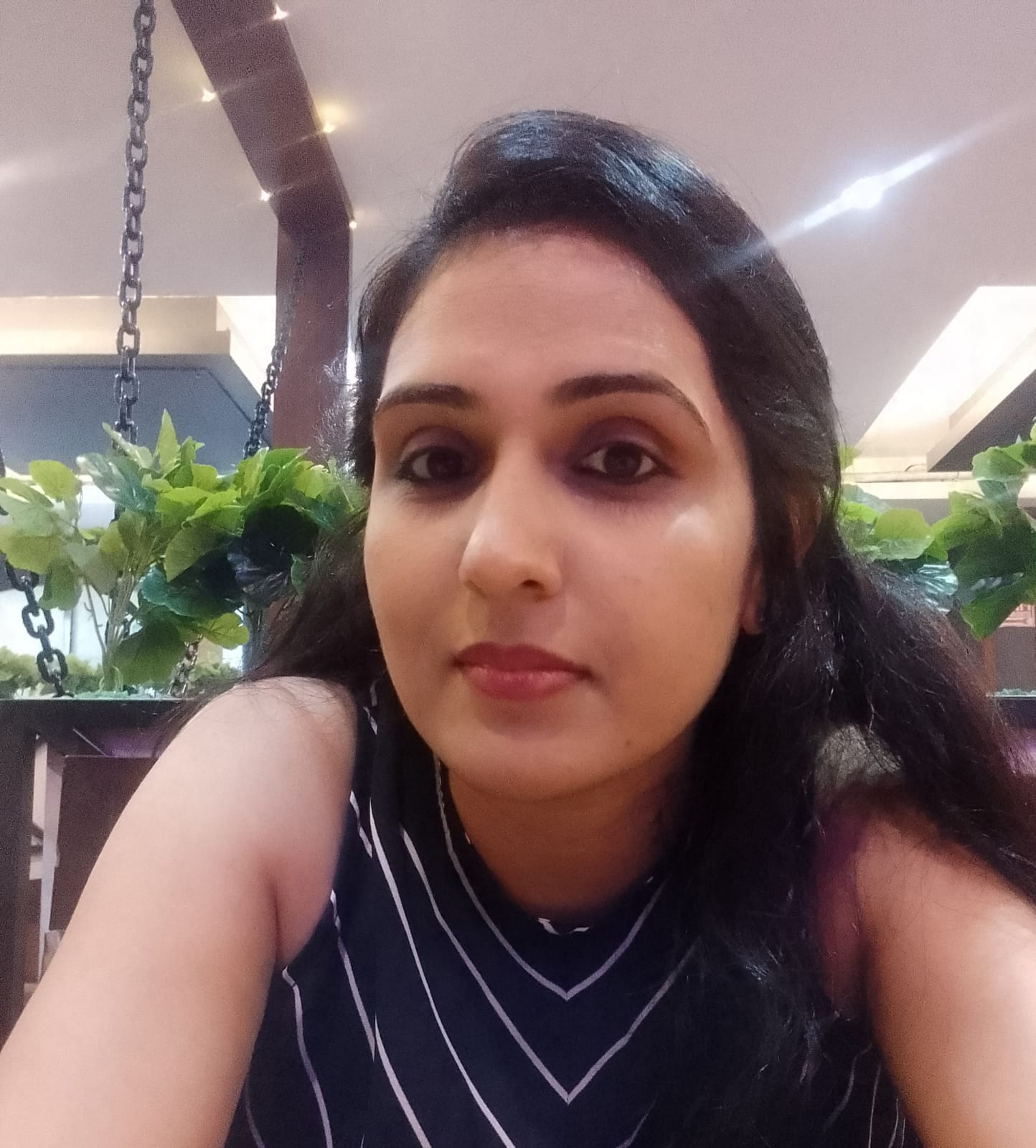
61 Views
Introduction In this tutorial, we implement examples using C++ to find the number of times an input string occurs in an array of the range [l, r]. Only lowercase alphabets are used in the string array to resolve this task. Different strings are stored in the string array and traversed to check if a particular string exists. This is for a given range of L and R. L and R are the starting and ending index values of an array to search a string for that range in the input string array. Find string lying between L and R of ... Read More
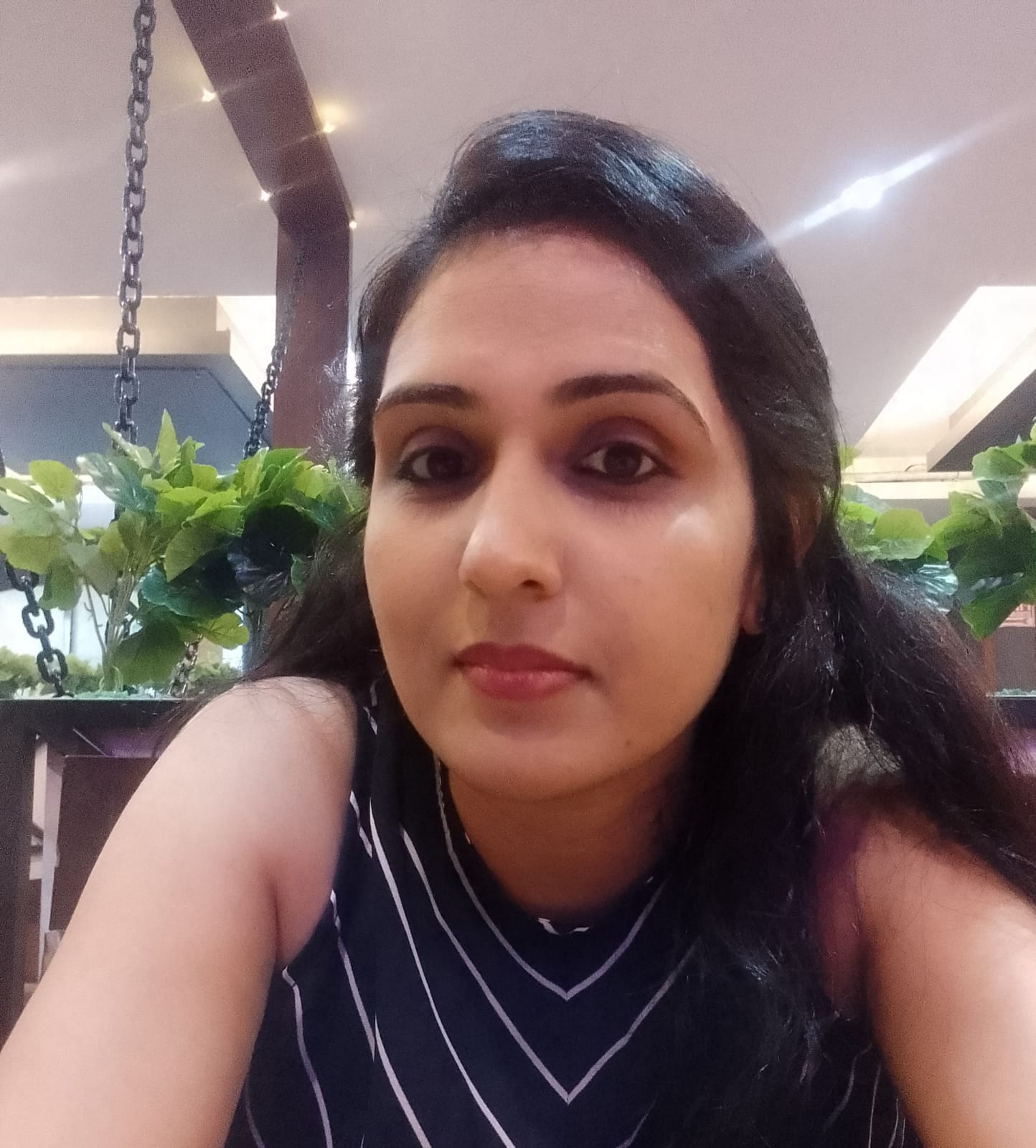
104 Views
Introduction In this tutorial, we implement 2 examples using C++ to find the minimum number of substrings in a given string. Those substrings are all powers of 5 that means, the substrings are the factors of the number 5. To implement the example, take an input binary string and generate the minimum possible substrings that are factors of 5. If you want to know whether a substring is a power of 5 or not, check its decimal values. The binary string is a combination of 1 and 0 and we cannot find a particular binary string that is ... Read More
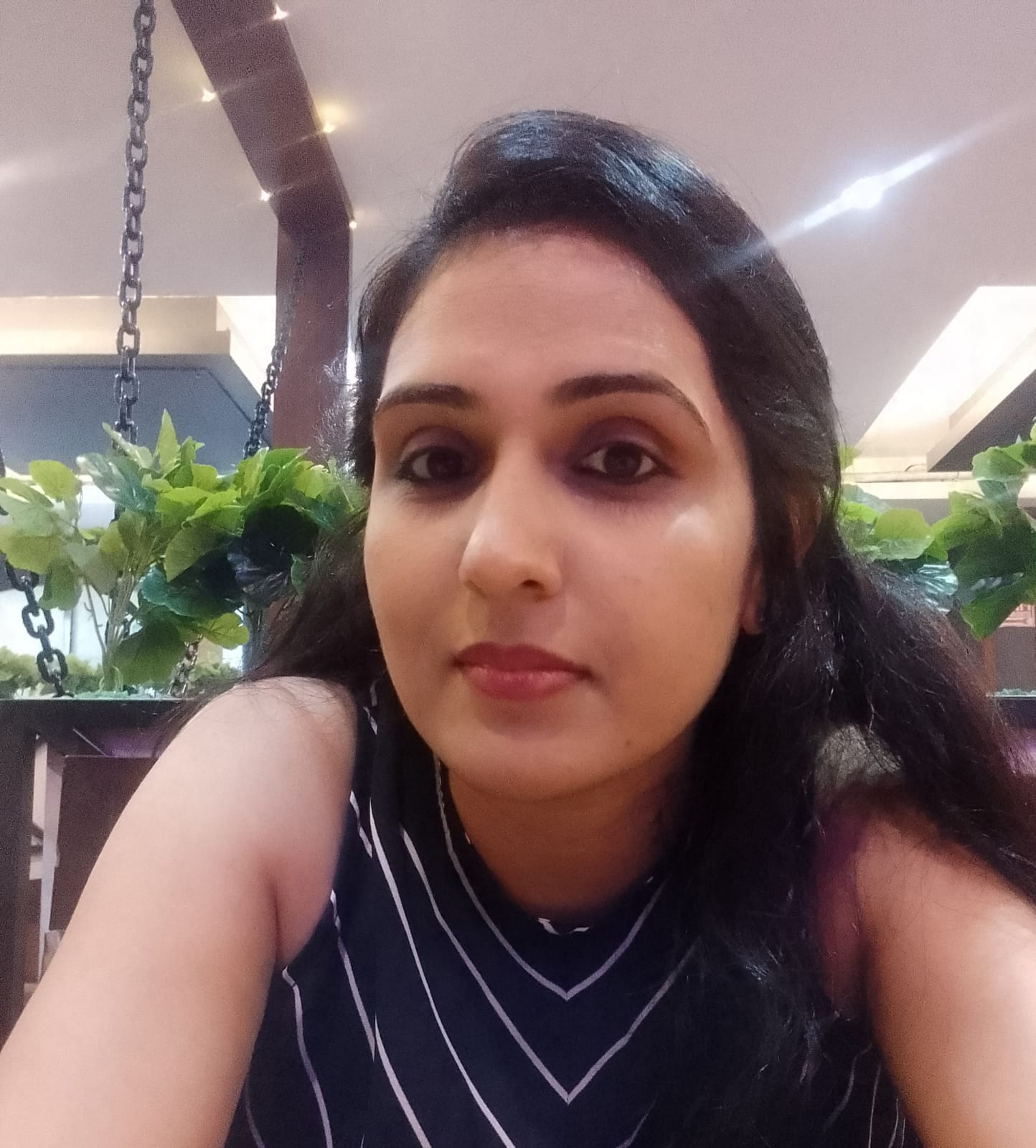
207 Views
Introduction In this tutorial, we use C++ programming concepts to implement examples to find the minimum cost to modify a string. String modification includes operations to change one string into another string. String operations include insertion, deletion, and substitution. We predefined the cost of each operation. You can choose the cost values of your choice. Generate output by calculating the total operation cost for string modification. The insertion function is used to insert missing characters, deletion is used to remove unwanted characters, and the substitution operation is used to replace a character with another character. For implementing the above ... Read More
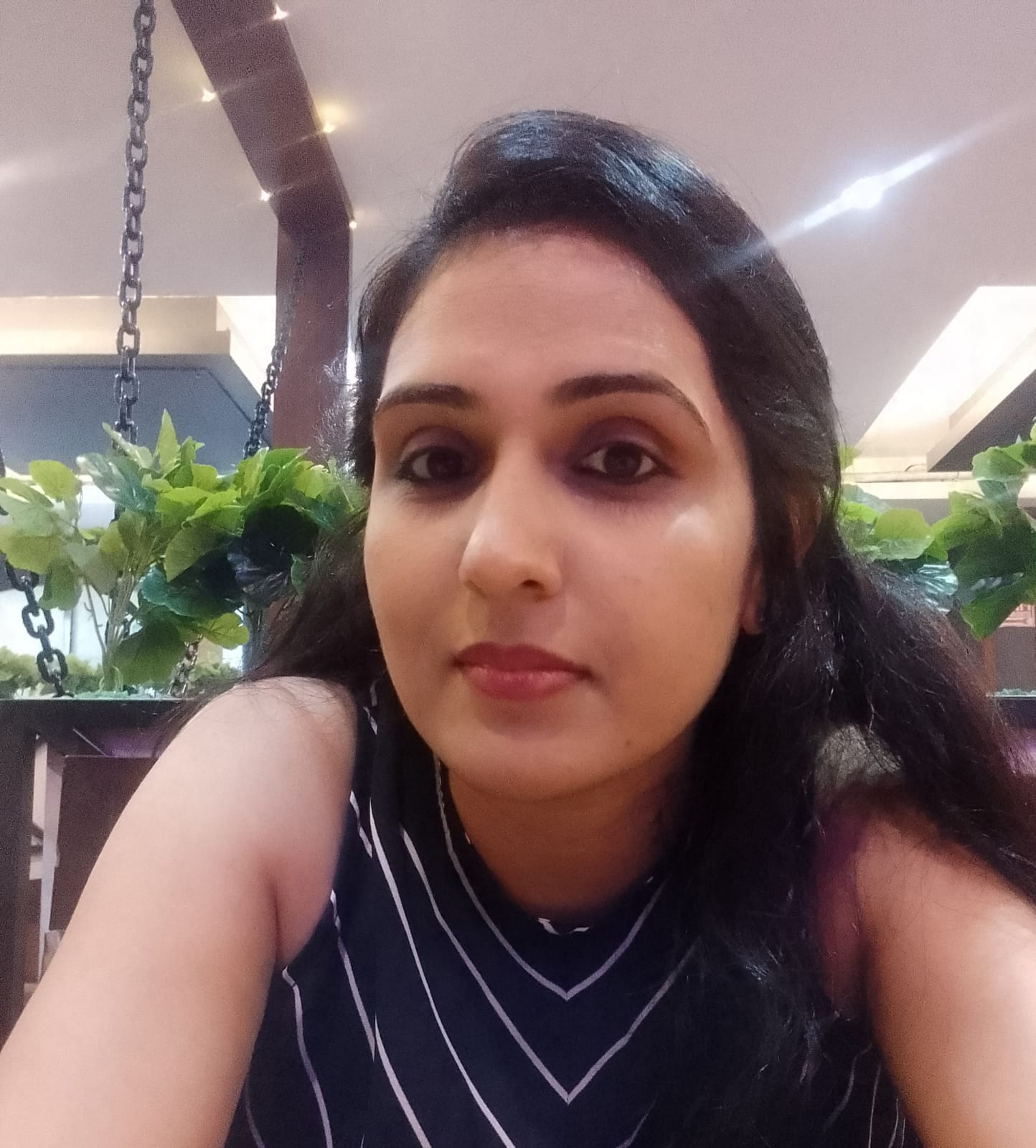
91 Views
Introduction A palindrome is one that reads the same forward and backward. An example of a palindrome string is Mam. In this tutorial, we use C++ programming to find the maximum length palindrome of a string by predefining the range of characters. Our task is to find the largest length of a palindrome string using the input string. We define the range of characters to generate that string. Depending on the situation, L and R can hold any value. Demonstration 1 String = “amem” Range = {1, 4} Output 3 In the above demonstration, the ... Read More
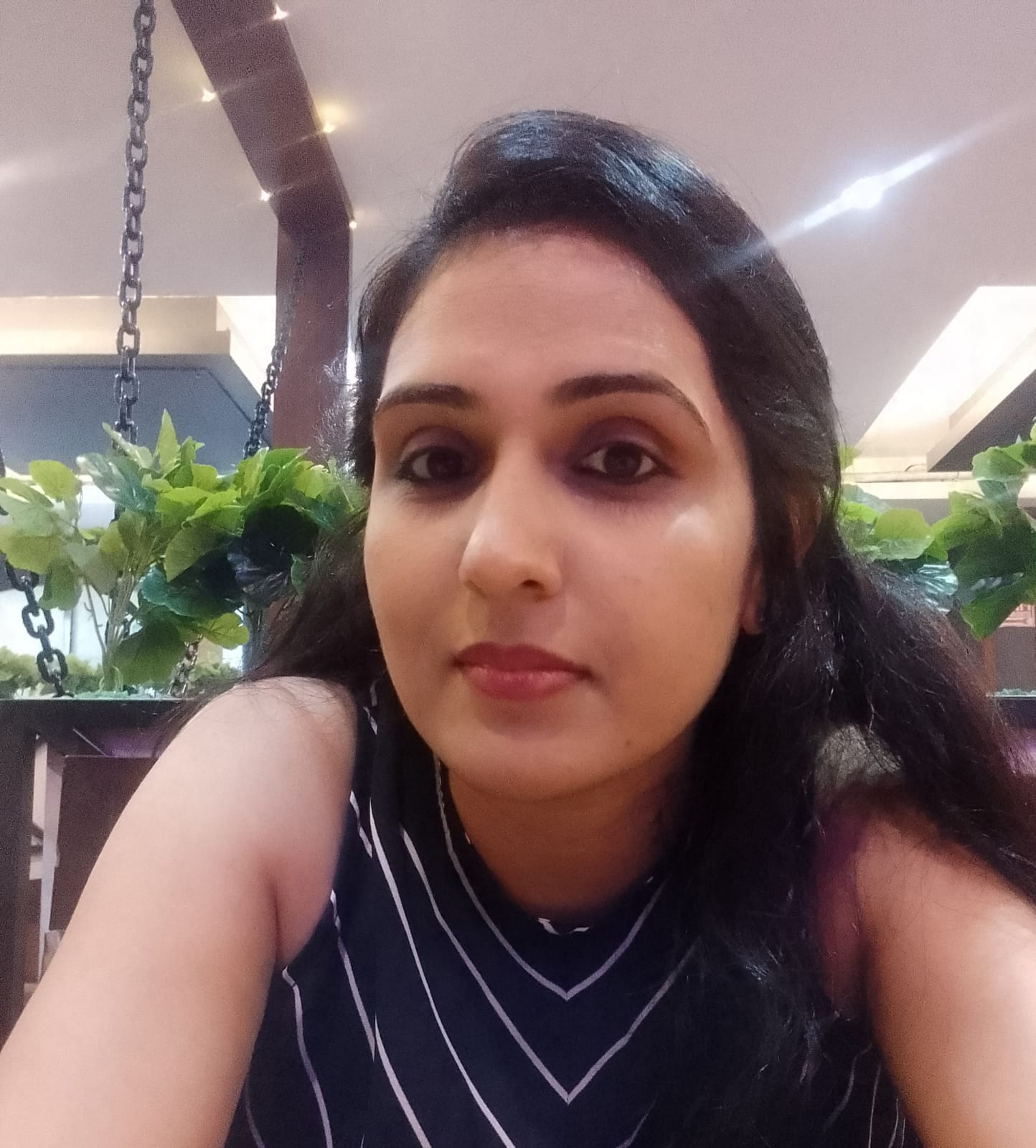
68 Views
Introduction In this tutorial, we implement an approach to find the longest substring of only 4 using the first N characters of the infinite string. Infinite string using 4 looks like this: “44444444……” and for this string we define the length of characters to consider for solving the task. To solve the problem of this tutorial, consider an input numerical string, We solve this problem using two conditions and those conditions are as follows: Consider an input string with random digits and generate the longest substring of 4’s from the string. We consider an infinite string of combinations of ... Read More
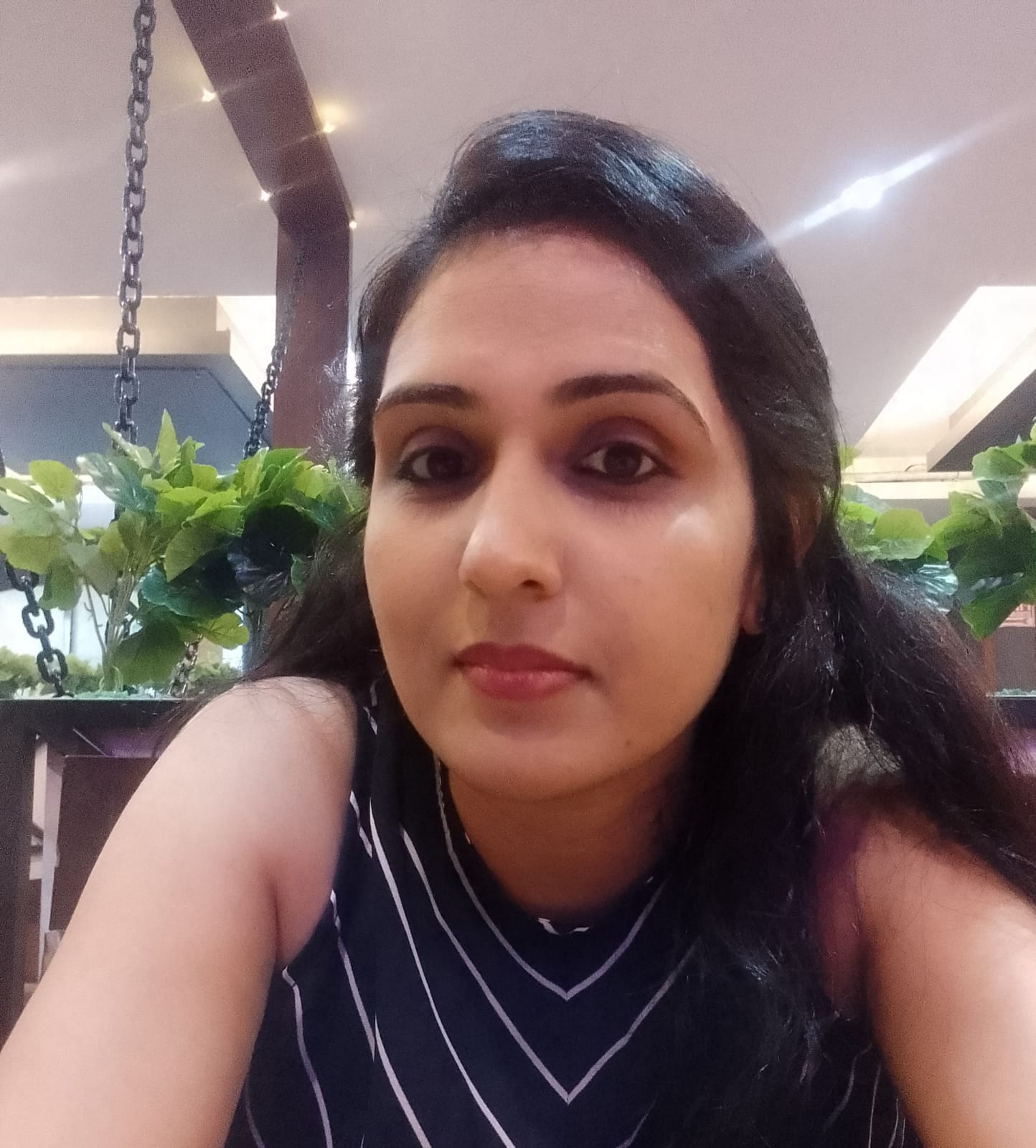
72 Views
Introduction In this tutorial, we discuss the problem of finding the largest component size in a graph generated by connecting non-co-prime nodes through C++. Graphs are formed by nodes connected by edges. The components of the graph are a subset of values that form nodes. There is an array a[] which forms graph G. The components of the graph are a subset of values that form nodes. The non-coprime numbers are the numbers that have a HCF (Highest Common Factor) other than 1, that means they have some other common factors. We solve the problem statement in this tutorial using ... Read More
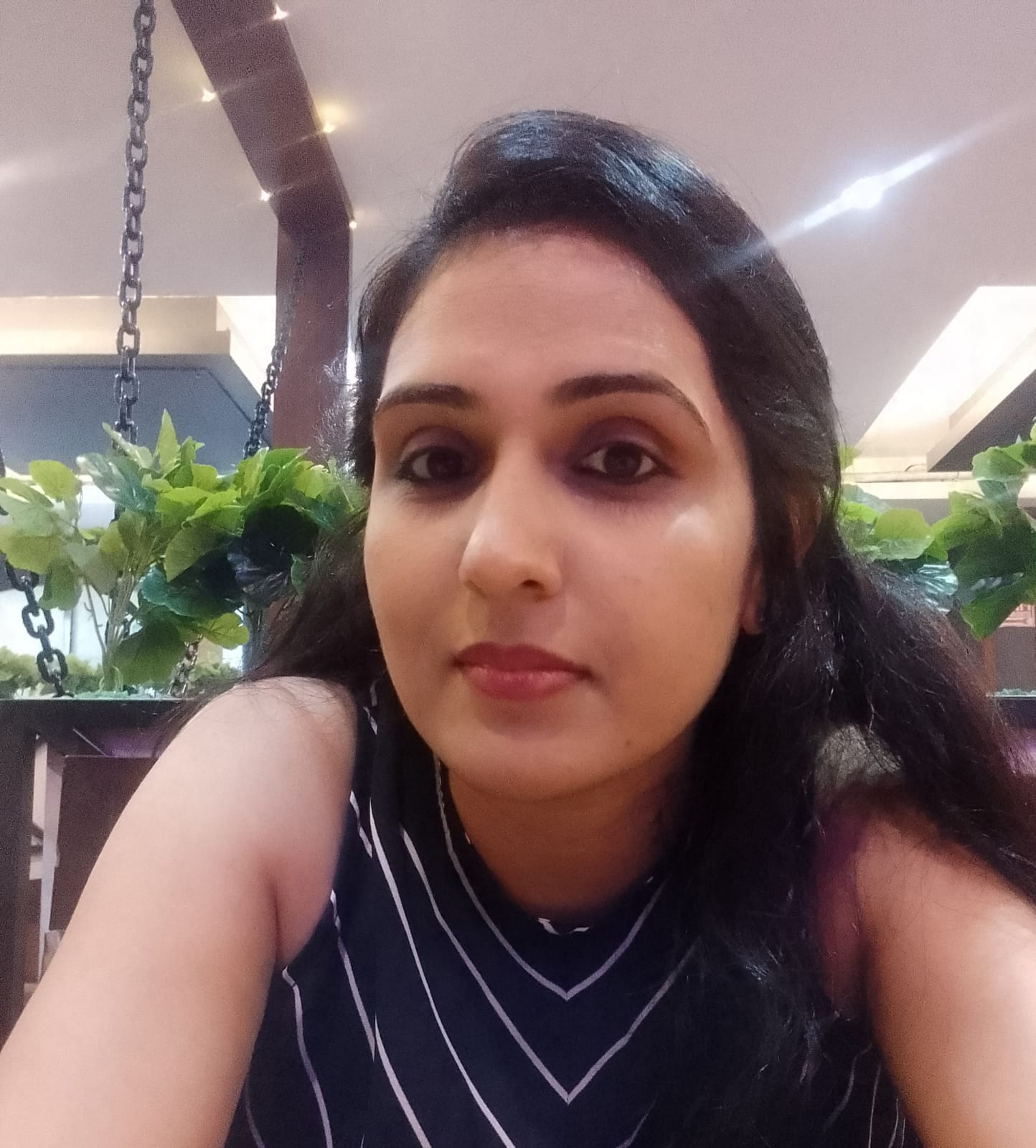
534 Views
Introduction In this tutorial, we will discuss probabilistic data structures in detail. This tutorial will cover the meaning of a Probabilistic Data Structure, its types, and its benefits. When dealing with large data sets or Big Data, basic data structures that use hashtables or HashSets would not be effective enough. As the data size increases, memory requirements increase with limited time for solving a query which restricts the functionality of deterministic basic data structures. Probabilistic data structures are approximate data structures that are collections of data structures. They are called so because they do not provide exact values. They ... Read More