- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 1862 Articles for Data Structure
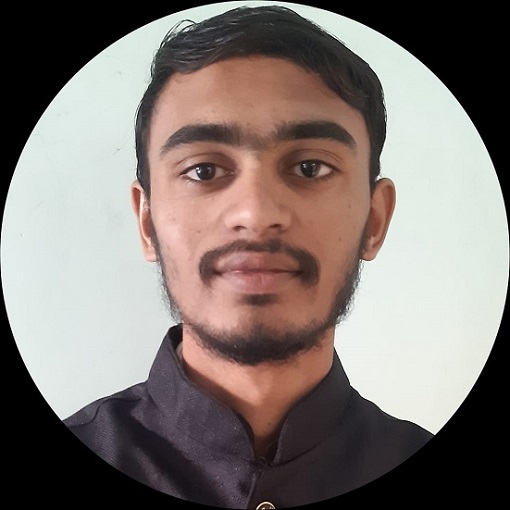
66 Views
In this problem, we require to find the minimum jumps required to make to reach the largest and smallest character in the given string. We can move to the next or previous character in one jump. We can solve the problem by finding the position of the lexicographically largest and smallest character in the given string. After that, we can find the minimum jumps required to the found indexes from the left and right sides. Problem statement – We have a string str of length N containing the alphabetical characters in the uppercase. We need to find the minimum number ... Read More
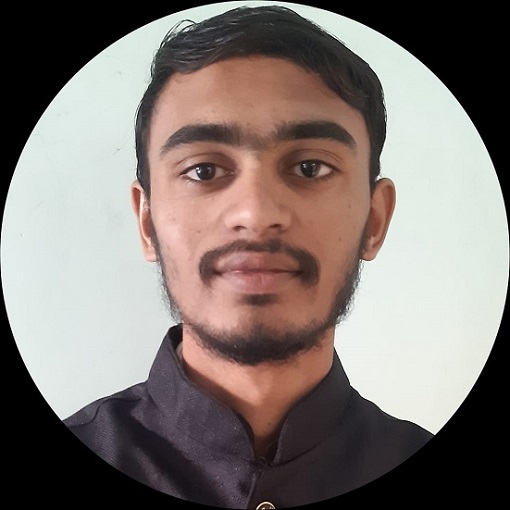
79 Views
In this problem, we need to find the smallest substring so that we can replace its character and make the frequency of each character equal to the N/3 in the given string. We can use the sliding window technique to solve the problem. We can find the minimum window size, which contains all excess characters, that will be the answer to the problem. Problem statement – We have given a string alpha. The size of the alpha is N which is always divisible by 3. The given task is to find the minimum length of the substring so that we ... Read More
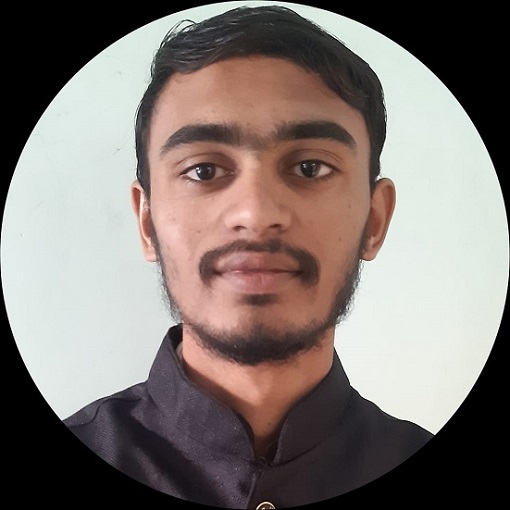
65 Views
In this problem, we require to determine the minimal cost to make the string’s left and right rotation same. Here is the observation which we will use to solve the problem. All characters should be equal for strings with odd lengths to make the left and right rotations the same. The string with an even length should have characters same at the even and odd indexes. Problem statement – We have a string of size N containing the different characters. We need to determine the minimum cost to make the left and right rotations of the given ... Read More
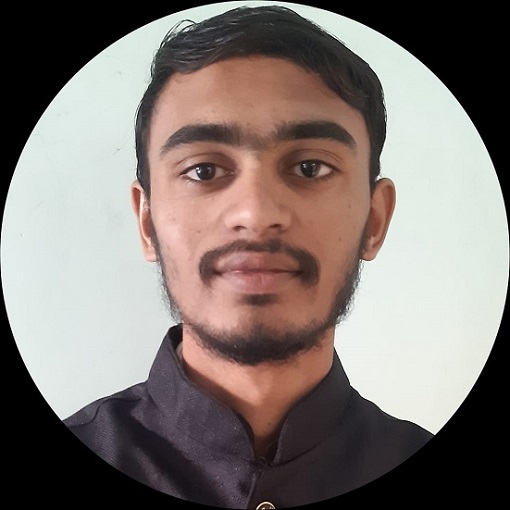
76 Views
In this problem, we need to change the minimum characters in the given string to make its left and right rotations the same. In the string, we can observe that we can only make the left and right rotations of the string same if the string has an odd length and all characters are same, or the string has an even length and characters at even and odd indexes the same. For example, abab – Left and right rotation of the string is baba and baba. aaa – Left and right rotation of the stirng is aaa and aaa. ... Read More
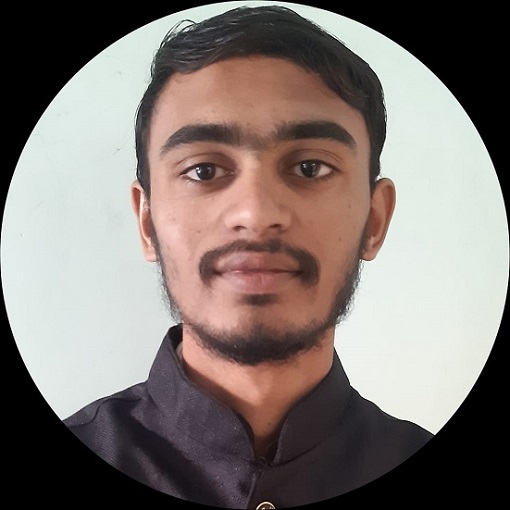
76 Views
In this problem, we will write Java code to find the maximum sum of consecutive zeros at the start and end of any string rotation. First, we will use a naïve approach to solve the problem, which generates all rotations of the binary string and counts the starting and ending consecutive zeros. After that, we will learn an optimized algorithm that counts the maximum consecutive zeros. Problem statement – Here, we have a string of size N containing only 0 and 1 characters. We need to find the maximum sum of consecutive zeros at the start and end of any ... Read More
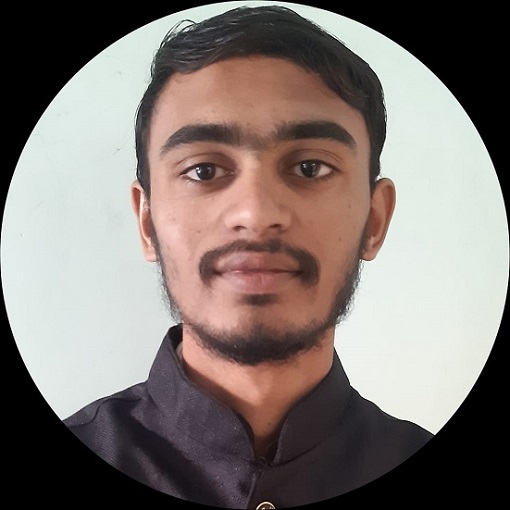
105 Views
In this problem, we will learn to implement the unrolled linked list. The unrolled linked list is a specialized version of the linked list. The normal linked list contains a single element in a single node, but the unrolled linked list contains a group of elements in each node. Also, insertion, deletion, and traversal in the unrolled linked list work the same as the typical linked list. The linear search is faster in the array than in the linked list. So, we can add elements in the array and an array in each node of the linked list. Also, ... Read More
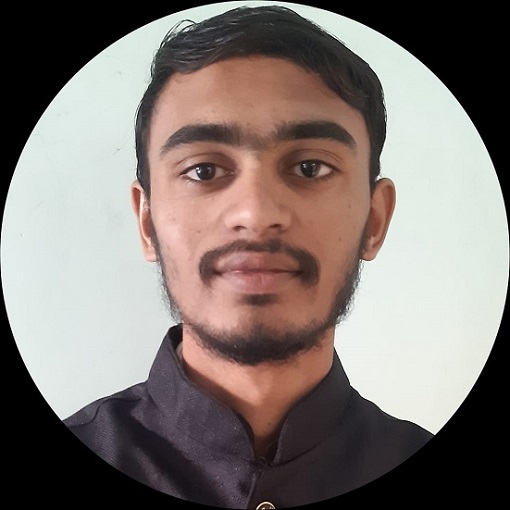
55 Views
In this problem, we need to implement Vizing's Theorem. Vizing's Theorem is used with graphs. Theorem statement - For any undirected graph G, the value of the Chromatic index is equal to the d or d + 1, where d is the maximum degree of the graph. The degree for any vertex is the total number of incoming or outgoing edges. Problem statement - We have given a graph and need to implement Vizing's Theorem to find the Chromatic index of the graph. Note - The chromatic index is a positive integer, requiring a ... Read More
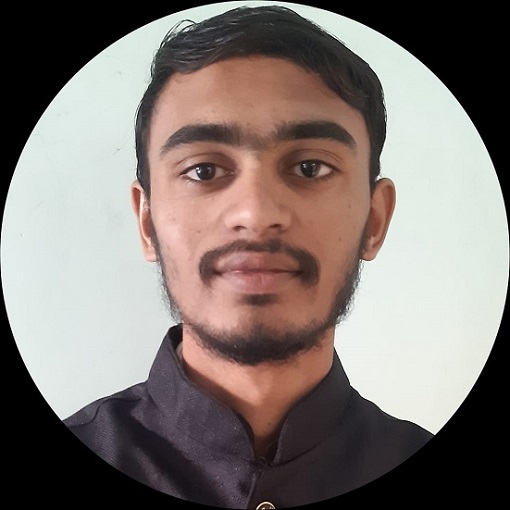
126 Views
The Schonhage-Strassen algorithm is useful when we need to multiply large decimal numbers. As Java supports the 1018 size of integers, and if we need to multiply the digits of more than 1018, we need to use the Schonhage-Strassen algorithm, as it is one of the fastest multiplication algorithms. It uses the basic rules for the multiplication of two numbers. It first performs the linear convolution and then performs the carry to get the final result. Problem statement - We have given mul1 and mul2 large decimal numbers and need to implement the Schonhage-Strassen algorithm to multiply both ... Read More
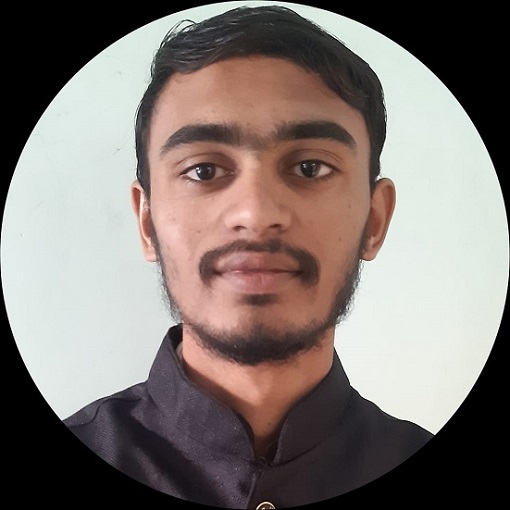
3K+ Views
The RSA name is given by their inventors which is used to encrypt the text with high security. The RSA technique is one of the most used techniques to encrypt text, as it is the asymmetric encryption algorithm. It encrypts the text by utilizing the mathematical properties of the prime numbers. In the RSA algorithm, the sender and receiver have private keys. Also, a common public key exists, which the sender shares with the receiver. The sender encrypts the plain text using their own public and private key, and the receiver decrypts the message using their private and public ... Read More
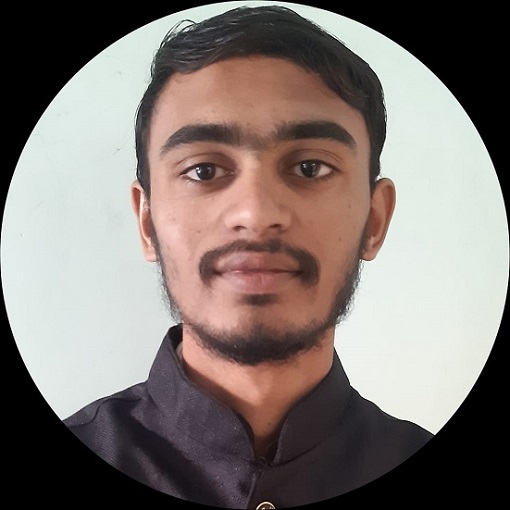
579 Views
In this problem, we need to convert the text to cipher text using the Monoalphabetic Cipher technique. All alphabetical characters are pre-mapped to their cipher character in the Monoalphabetic Cipher algorithm. So, we need to substitute all plain text characters with their mapped characters. Here is the table containing the mapping of characters. a$\mathrm{\rightarrow;}$Q b $\mathrm{\rightarrow;}$ W c $\mathrm{\rightarrow;}$ E d $\mathrm{\rightarrow;}$ R e $\mathrm{\rightarrow;}$ T ... Read More