- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7347 Articles for C++
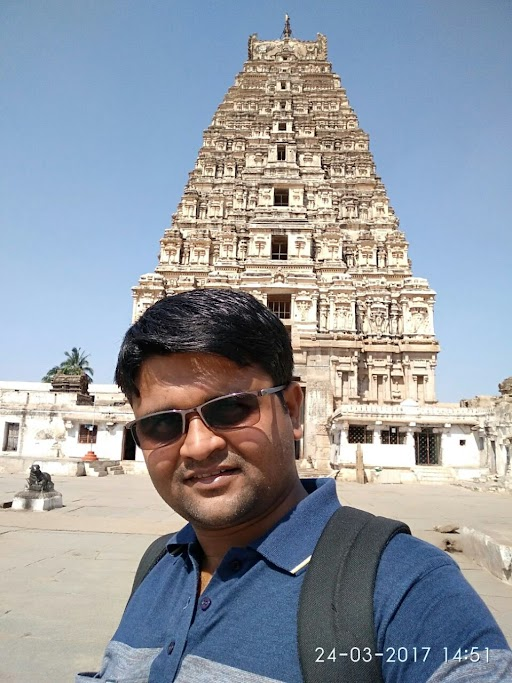
9K+ Views
C++ is a General purpose, middle-level, case sensitive, platform independent programming language that supports object oriented programming concept. C++ was created by Bjarne Stroustrup at Bell Labs in 1979. Since C++ is a platform independent programming language, it can be used on a variety of systems, including Windows, Mac OS, and various UNIX versions.Operators in C++ are used to perform specific operations on values and variables. The following are the list of operators used in C++Arithmetic operatorsAssignment operatorsComparison operatorsRelational OperatorsLogical operatorsBitwise operatorsUnary operatorsTernary/Conditional operators.From the above groups, Assignment operators are used to assign values to variables.Before learning about copy constructors, ... Read More
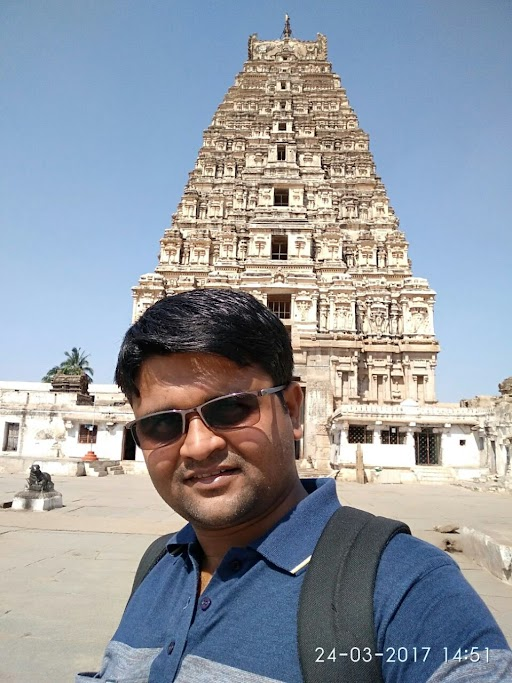
16K+ Views
Syntax defines the rules and regulations that help write any statement in a programming language, while semantics refers to the meaning of the associated line of code in the programming language. Read this article to learn more about syntax and semantics and how they are different from each other. What is Syntax? In a programming language, Syntax defines the rules that govern the structure and arrangement of keywords, symbols, and other elements. Syntax doesn't have any relationship with the meaning of the statement; it is only associated with the grammar and structure of the programming language. A line of ... Read More

939 Views
In this post, we will understand the difference between private and protected access modifiers in C++.Private Access ModifierThey are declared using the ‘private’ keyword, followed by a ‘:’.They can’t be accessed outside the class.The ‘private’ keyword is an access modifier that ensures that the functions and attributes inside the class are accessed only by class members in which they have been declared.Only member functions or friend functions are allowed to access data that is labelled as ‘private’.Example Live Demo#include using namespace std; class base_class{ private: string my_name; int my_age; public: void getName(){ ... Read More

76 Views
In this problem, we are given two values S and N denoting the speed of stream in Km/h and ratio of time with up and down streams. Our task is to Find speed of man from the speed of stream and ratio of time with up and down streams.Let’s take an example to understand the problem, InputS = 5, N = 2Output15Solution ApproachA simple solution to the problem is by using the mathematical formula for the rowing problems. So, let’s see how the formula will work −speed of man = x km/h speed of stream = S km/h speed of ... Read More

641 Views
In this problem, we are given a large number N. Our task is to find the smallest permutation of a given number.Let’s take an example to understand the problem, InputN = 4529016Output1024569Solution ApproachA simple solution to the problem is by storing the long integer value to a string. Then we will sort the string which is our result. But if there are any leading zeros, we will shift them after the first non zero value.Program to illustrate the working of our solution, Example Live Demo#include using namespace std; string smallestNumPer(string s) { int len = s.length(); sort(s.begin(), s.end()); ... Read More

956 Views
In this problem, we are given two values that are the sum (denoting the sum of digits) and digit (denoting the number of digits). Our task is to find the smallest number with a given number of digits and sum of digits.Let’s take an example to understand the problem, Inputsum = 15, dgiti = 2Output69ExplanationAll 2 digits numbers with sum 15 are : 69, 78, 87, 96.Solution ApproachA simple solution to the problem is by considering all the numbers with digit count as digit and find the smallest number whose sum of digit is equal to the sum.An efficient solution ... Read More

654 Views
In this problem, we are given a singly linked list. Our task is to find the smallest and largest elements in single linked list.Let’s take an example to understand the problem, Inputlinked List : 5 -> 2 -> 7 -> 3 ->9 -> 1 -> 4OutputSmallest element = 1 Largest element = 9Solution ApproachA simple solution to the problem is using by traversing the linked list node by node. Prior to this, we will initialise maxElement and minElement to the value of the first element i.e. head -> data. Then we will traverse the linked list element by element. And ... Read More

1K+ Views
In this problem, we are given a square matrix of size nXn. Our task is to Find smallest and largest element from square matrix diagonals. We need to find the smallest and largest elements of the primary and secondary diagonals of the matrox.Let’s take an example to understand the problem, Inputmat[][] = { {3, 4, 7}, {5, 2, 1}, {1, 8, 6} }OutputSmallest element in Primary Diagonal = 2 Largest element in Primary Diagonal = 6 Smallest element in Secondary Diagonal = 1 Largest element in Secondary Diagonal = 7Solution ApproachA simple solution to solve the problem ... Read More

82 Views
In this problem, we are given four values x1, y1, x2, y2 denoting two points (x1, y1) and (x2, y2). Our task is to find single movement in a Matrix. We need to find the direction using which we can move from one point (x1, y1) to (x2, y2). There can be any number of moves by the direction needed to be single and we need to return the direction in the form - “left” , “right”, “up”, “down”. Otherwise return -1, denoting “not possible”.Let’s take an example to understand the problem, Inputx1 = 2, y1 = 1, x2 = ... Read More

140 Views
In this problem, we are given an NxN binary matrix bin[][]. Our task is to find the size of the largest ‘+’ formed by all ones in a binary matrix.Let’s take an example to understand the problem, Input0 1 1 1 1 1 0 1 0Output5Solution ApproachA simple solution to the problem is to find the largest ‘+’ for which we need to find the maximum number of 1’s in one direction for a point in the matrix which needs to be the same in all four directions for a given 1. For this, we will create one matrix for ... Read More