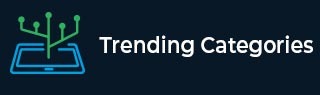
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 871 Articles for Automation Testing

3K+ Views
We can select an option from the dropdown list with Selenium webdriver. The Select class is used to handle static dropdown. A dropdown is identified with the tag in an html code.Let us consider the below html code for tag.We have to import org.openqa.selenium.support.ui.Select to work with methods under Select class in our code. Let us see some of the Select methods−selectByVisibleText(arg) – An option is selected if the text visible on the dropdown is the same as the parameter arg passed as an argument to the method.Syntaxsel = Select (driver.findElement(By.id ("option")));sel.selectByVisibleText ("Selenium");selectByValue(arg) – An option is selected ... Read More

492 Views
We can simulate HTML5 drag and drop with Selenium webdriver. This is a feature that gets implemented if an element is dragged from its position and dropped on another element in another position.Actions class in Selenium is used for taking care of this functionality. The drag_and_drop(source, target) is the available method under Actions class for carrying out this task. We have to import from selenium.webdriver import ActionChains to our code to use this method of Actions class.Let us take the two elements and try to drag the first element on to the second element.Examplefrom selenium.webdriver import ActionChains from selenium import ... Read More

4K+ Views
We can find an element by attributes with Selenium webdriver. There are multiple ways to do this. We can use the locators like css and xpath that use attributes and its value to identify an element.For css selector, theexpression to be used is tagname[attribute='value']. There are two types of xpath – absolute and relative. The xpath expression to be used is //tagname[@attribute='value'], the tagname in the expression is optional. If omitted, the xpath expression should be //*[@attribute='value'].Let us consider an element with input tagname. It will be identified with xpath locator with id attribute (//input[@id='txtSearchText']).Examplefrom selenium import webdriver driver = ... Read More

13K+ Views
We can click on Google search with Selenium webdriver. First of all we need to identify the search edit box with help of any of the locators like id, class, name, xpath or css.Then we will input some text with the sendKeys() method. Next we have to identify the search button with help of any of the locators like id, class, name, xpath or css and finally apply click() method on it or directly apply submit() method. We will wait for the search results to appear with presenceOfElementLocatedexpected condition.We need to import org.openqa.selenium.support.ui.ExpectedConditions and import org.openqa.selenium.support.ui.WebDriverWait to incorporate expected conditions ... Read More

2K+ Views
We can erase the contents from text boxes with Selenium webdriver. This is done with the clear() method. This method clears the edit box and also makes the field enabled.First of all we need to identify the element with help of any of the locators like id, class, name, xpath or css and then apply sendKeys() method to type some text inside it. Next we shall apply the clear() method on it. To check if the edit box got cleared, we shall use getAttribute() method and pass value parameter as an argument to the method. We shall get blank value ... Read More

7K+ Views
We can get text from a webelement with Selenium webdriver. The getText() methods obtains the innerText of an element. It fetches the text of an element which is visible along with its sub elements. It ignores the trailing and leading spaces.First of all we need to identify the element with help of any of the locators like id, class, name, xpath or css and then apply getText() method on it to get the text content of the element.Let us get the text of the element About Careers at Tutorials on the page−Exampleimport org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import ... Read More

5K+ Views
We can check if an element contains a specific class attribute value. The getAttribute() method is used to get the value of the class attribute. We need to pass class as a parameter to the getAttribute() method.First of all we need to identify the element with help of any of the locators like id, class, name, xpath or css. Then obtain the class value of the attribute. Finally, we need to check if the class attribute contains a specific value.Let us take an element with the below html code having class attribute. The tp-logo is the value of the class ... Read More

487 Views
We can get a parent HTML tag with Selenium webdriver. First of all we need to identify the child element with help of any of the locators like id, class, name, xpath or css. Then we have to identify the parent with the findElement(By.xpath()) method.We can identify the parent from the child, by localizing it with the child and then passing (parent::*) as a parameter to the findElement(By.xpath()). Next to get the tagname of the parent, we have to use the getTagName() method.Syntaxchild.findElement(By.xpath("parent::*"));Let us identify tagname of parent of child element li in below html code−The tagname of the parent ... Read More

1K+ Views
We can work with isDisplayed() method in Selenium webdriver. This method checks if a webelement is visible on the page. If it is visible, then the method returns a true value, else it returns false.First of all, we have to identify the element with any of the locators like id, class, name, xpath or css and then apply isDisplayed() method on it.Syntaxboolean s= driver.findElement(By.id("txt-bx")).isDisplayed();Let us check if the element About Careers at Tutorials Point is displayed on the page. Since it is available it shall return a true value.Exampleimport org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public ... Read More

843 Views
We can get the current URL of a page with Selenium webdriver. The method current_url is available which obtains the present page URL and then we can print the result in the console.Syntaxs = driver.current_urlLet us find the URL of the page presently navigated and we shall get https://www.tutorialspoint.com/index.htm as the output. Examplefrom selenium import webdriver driver = webdriver.Chrome(executable_path="C:\chromedriver.exe") driver.implicitly_wait(0.5) driver.get("https://www.tutorialspoint.com/index.htm") #identify current URL with current_url l= driver.current_url print(Current URL is: " + l) driver.close() Output Read More