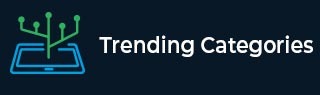
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 871 Articles for Automation Testing

1K+ Views
We can mute all sounds in Chrome with Selenium webdriver. To mute the audio we have to set parameters for the browser. For Chrome, we shall use the ChromeOptions class.We shall create an object of the ChromeOptions class. Then utilize that object to invoke the addArguments method. Then pass −mute−audio as a parameter to that method. Finally, send this information to the driver object.SyntaxChromeOptions op = new ChromeOptions(); op.addArguments("−−mute−audio"); WebDriver d = new ChromeDriver(op);For Firefox, we shall use the FirefoxOptions class and create an object for that class. Then utilize that object to invoke the addPreference method and pass media.volume_scale ... Read More

11K+ Views
We can make Selenium wait for 10 seconds. This can be done by using the Thread.sleep method. Here, the wait time (10 seconds) is passed as a parameter to the method.We can also use the synchronization concept in Selenium for waiting. There are two kinds of wait − implicit and explicit. Both these are of dynamic nature, however the implicit wait is applied to every step of automation, the explicit wait is applicable only to a particular element.ExampleCode Implementation with sleep method.import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class WaitThrd{ public static void main(String[] args) throws ... Read More

205 Views
We can maximize browser window with Selenium webdriver in Python. It is a good practise to maximize the browser while running the tests to reduce the chance of failure.Once a browser is maximized, the majority of elements become visible to the screen and the probability of interaction with the driver increases. Also, with a maximized browser, users have a better view of the elements on the page.Some of ways of maximizing browser −With the maximize_window methodSyntaxdriver.maximize_window()With the fullscreen_window methodSyntaxdriver.fullscreen_window()ExampleCode Implementation with maximize_window()from selenium import webdriver #set chromedriver.exe path driver = webdriver.Chrome (executable_path="C:\chromedriver.exe") # maximize browser driver.maximize_window() driver.get("https://www.tutorialspoint.com/index.htm") driver.close()ExampleCode Implementation with ... Read More

4K+ Views
We can take a screenshot of a full page with Selenium webdriver in Python with chromedriver. First of all, we shall obtain the original window size with the get_window_size method.Then with the help of JavaScript Executor we shall fetch the complete height and width of the page which is opened on the browser. Then set the window size to that dimension with the set_window_size method.Next, capture the screenshot of the entire content within the body tag in the html with the screenshot method. This method accepts the path of the screenshot that will be captured as a parameter.Examplefrom selenium import ... Read More

263 Views
We can start the Selenium browser(like Firefox) in minimized mode. This will be achieved by taking the help of the Dimension class. We shall create an object of this class.While creating the object , we shall pass the dimensions of the browser size as parameters to the Dimension class. Finally pass the object as parameter to the setSize method.SyntaxDimension s = new Dimension(100, 200); driver.manage().window().setSize(s);Exampleimport org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.Dimension; public class FirefoxBrwSize{ public static void main(String[] args) { System.setProperty("webdriver.gecko.driver", "C:\Users\ghs6kor\Desktop\Java\geckodriver.exe"); WebDriver driver = new ... Read More

2K+ Views
We can PID of browsers launched by Selenium webdriver. First of all, we have to create an object of the webdriver. Next, for example, to launch the browser in the Firefox browser, we have to take the help of webdriver.Firefox() class.The location of the geckodriver.exe file is passed as a parameter to that class. This is done by setting the path to executable_path property. Then, the browser shall be launched with the get method.Finally, to obtain the PID of the browser, we shall use the driver.service.process.id method.Syntaxs = driver.service.process.pidExamplefrom selenium import webdriver #path of geckodriver.exe driver = webdriver.Firefox(executable_path="C:\geckodriver.exe") #launch browser ... Read More

1K+ Views
We can start chromedriver in headless mode. Headless execution is getting popular now−a−days since the resource consumption is less and execution is done at a faster speed.Post version 59, Chrome supports headless execution. ChromeOptions class is utilized to modify the default characteristics of the browser. The addArguments method of the ChromeOptions class is used for headless execution and headless is passed as a parameter to that method.SyntaxChromeOptions opt = new ChromeOptions(); opt.addArguments("headless"); WebDriver drv = new ChromeDriver(opt);Exampleimport org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class HeadlessChrome{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", ... Read More

2K+ Views
We can parse a website using Selenium and Beautiful Soup in Python. Web Scraping is a concept used to extract content from the web pages, used extensively in Data Science and metrics preparation. In Python, it is achieved with the BeautifulSoup package.To have BeautifulSoup along with Selenium, we should run the command −pip install bs4 seleniumLet us scrap the below links appearing on the page −Then investigate the html structure of the above elements −Examplefrom selenium import webdriver from bs4 import BeautifulSoup #path of chromedriver.exe driver = webdriver.Chrome (executable_path="C:\chromedriver.exe") #launch browser driver.get ("https://www.tutorialspoint.com/about/about_careers.htm") #content whole page in html format s ... Read More

4K+ Views
We can get console.log output from Chrome with Selenium Python API bindings. We will perform this with the DesiredCapabilities class. We shall enable the logging from the browser with DesiredCapabilities.Chrome setting.We have to pass this browser capability to the driver object by passing it as a parameter to the Chrome class. To enable logging we shall set the property goog:loggingPrefs of the browser to 'browser':'ALL'.SyntaxSyntax:dc = DesiredCapabilities.CHROME dc['goog:loggingPrefs'] = { 'browser':'ALL' } driver = webdriver.Chrome(desired_capabilities=dc)Examplefrom selenium import webdriver from selenium.webdriver.common.desired_capabilities import DesiredCapabilities #set browser log dc = DesiredCapabilities.CHROME dc['goog:loggingPrefs'] = { 'browser':'ALL' } driver = webdriver.Chrome(executable_path="C:\chromedriver.exe", desired_capabilities=dc) #launch browser driver.get ... Read More

230 Views
We can run Selenium webdriver with Python bindings in Chrome. This can be done by downloading the chromedriver.exe file. Visit the link: https://chromedriver.chromium.org/downloads. There shall be links available for download for various chromedriver versions.Select the version which is compatible with the Chrome browser in the local system. Click on it. As the following page is navigated, select the zip file available for download for the operating system which is compatible with our local operating system.Once the zip file is downloaded, extract it and save the chromodriver.exe file at a location. To launch the Chrome, we have to add the statement from ... Read More