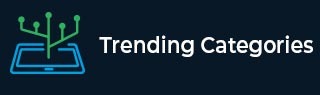
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 871 Articles for Automation Testing

17K+ Views
We can find an element using the attribute id with Selenium webdriver using the locators - id, css, or xpath. To identify the element with css, the expression should be tagname[id='value'] and the method to be used is By.cssSelector.To identify the element with xpath, the expression should be //tagname[@id='value']. Then, we have to use the method By.xpath to locate it. To locate an element with locator id, we have to use the By.id method.Let us look at the html code of an element with id attribute −SyntaxWebElement e = driver. findElement(By.id("session_key")); WebElement m = driver. findElement(By.xpath("//input[@id=' session_key']")); WebElement n = ... Read More

5K+ Views
We can get the tooltip text in Selenium webdriver with help of the method - getAttribute. The attribute title should be passed as a parameter to this method.This technique is only applicable if the element has a title attribute.The tooltip text is the one which gets displayed on hovering the mouse over the element. In the below html code, an element having a tooltip has the attribute title and the value set for title is actually the tooltip text.The below image shows the menu Coding Ground showing the tooltip text as - Coding Ground - Free Online IDE and Terminal.SyntaxWebElement ... Read More

34K+ Views
We can verify whether an element is present or visible in a page with Selenium webdriver. To check the presence of an element, we can use the method – findElements.The method findElements returns a list of matching elements. Then, we have to use the method size to get the number of items in the list. If the size is 0, it means that this element is absent from the page.Syntaxint j = driver.findElements(By.id("txt")).size();To check the visibility of an element in a page, the method isDisplayed() is used. It returns a Boolean value( true is returned if the element is visible, ... Read More

2K+ Views
We can find elements in a web page with the help of JavaScript. We can also validate the elements returned by the JavaScript methods in the browser Console (Pressing F12). JavaScript methods to find elements are −getElementsByTagnameTo obtain a collection of elements with the matching tagname passed as parameter to the method. If there are no matching elements, an empty collection is returned.Syntaxdocument.getElementsByTagName("") To get the first matching element, document.getElementsByTagName("")[0]getElementsByNameTo obtain a collection of elements with the matching value of name attribute passed as parameter to the method. If there are no matching elements, an empty collection is returned.Syntaxdocument.getElementsByName("") To ... Read More

9K+ Views
A hyperlink on a page is identified with the anchor tag. To click a link, we can use the link text locator which matches the text enclosed within the anchor tag.We can also use the partial link text locator which matches the text enclosed within the anchor tag partially. NoSuchElementException is thrown if there is no matching element found by both these locators.SyntaxWebElement t =driver.findElement(By.partialLinkText("Refund")); WebElement m =driver.findElement(By.linkText("Refund Policy"));Let us make an attempt to click a link Refund Policy on the below page −ExampleCode Implementation with linkTextimport org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; public class LnkClick{ ... Read More

2K+ Views
We can get the typed text from a textbox with Selenium webdriver. Firstly, we have to enter text in the text box (after being identified with any locators) with the help of the sendKeys method.Then apply the method getAttribute to obtain the text entered in that field and pass the parameter value to that method. Let us make an attempt to obtain the value entered in the Google search box −SyntaxWebElement m = driver.findElement(By.name("q")); String st = m.getAttribute("value");Exampleimport org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; public class GetTextTyped{ public static void main(String[] args) { ... Read More

3K+ Views
We can get the text found within the span tag with Selenium webdriver.The text of a web element can be captured with the method getText. Let us see an example of an element having the text - © Copyright 2021. All Rights Reserved enclosed within the span tag.SyntaxWebElement l = driver.findElement(By.xpath("//p/span")); String s = l.getText();Exampleimport org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; public class SpanText{ public static void main(String[] args) { System.setProperty("webdriver.gecko.driver", "C:\Users\ghs6kor\Desktop\Java\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); //implicit wait driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); ... Read More

3K+ Views
To specify ENTER button functionality in Selenium webdriver we have to use the method sendKeys. To simulate pressing the ENTER button, we have to add the statement import org.openqa.selenium.Keys to our code.Then pass the parameter – Keys.RETURN or Keys.ENTER to the sendKeys method.Let us make an attempt to press the ENTER button after entering some text in the Google search input box −ExampleCode Implementation with Keys.ENTERimport org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.Keys; public class EnterOperation{ public static void main(String[] args) { System.setProperty("webdriver.gecko.driver", "C:\Users\ghs6kor\Desktop\Java\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); ... Read More

5K+ Views
We can get the text from a list of all web elements with the same class name in Selenium webdriver. We can use any of the locators like the class name with method By.className, xpath with method By.xpath, or css with method By.cssSelector.Let us verify a xpath expression //h2[@class='store-name'] which represents multiple elements that have the same class name as store-name. If we validate this in Console with the expression - $x("//h2[@class='store-name']"), it yields all the matching elements as shown below:Also, since we need to obtain multiple elements, we have to use the findElements method which returns a list. We ... Read More

375 Views
We can use the Selenium webdriver scripts in Firefox (versions>47) with the help of the geckodriver.exe executable file. First, we have to download this file from the following link −https://github.com/mozilla/geckodriver/releasesOnce we navigate to the mentioned URL, we have to click a link based on the operating system (Windows, Linux or Mac) we are presently using. As the download gets over, a zip file is created. We have to extract the zip file and store the geckodriver.exe file in a desired location.Then, we have to configure the path of the geckodriver.exe file using the System.setProperty method along with creating an object ... Read More