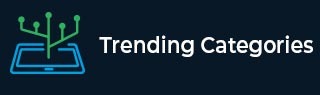
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 210 Articles for Analysis of Algorithms

17K+ Views
Here we will see what are the different applications of DFS and BFS algorithms of a graph?The DFS or Depth First Search is used in different places. Some common uses are −If we perform DFS on unweighted graph, then it will create minimum spanning tree for all pair shortest path treeWe can detect cycles in a graph using DFS. If we get one back-edge during BFS, then there must be one cycle.Using DFS we can find path between two given vertices u and v.We can perform topological sorting is used to scheduling jobs from given dependencies among jobs. Topological sorting ... Read More

42K+ Views
In this section we will see what is a graph data structure, and the traversal algorithms of it.The graph is one non-linear data structure. That is consists of some nodes and their connected edges. The edges may be director or undirected. This graph can be represented as G(V, E). The following graph can be represented as G({A, B, C, D, E}, {(A, B), (B, D), (D, E), (B, C), (C, A)})The graph has two types of traversal algorithms. These are called the Breadth First Search and Depth First Search.Breadth First Search (BFS)The Breadth First Search (BFS) traversal is an algorithm, ... Read More

524 Views
The binary search trees are binary tree which has some properties. These properties are like below −Every Binary Search Tree is a binary treeEvery left child will hold lesser value than rootEvery right child will hold greater value than rootIdeal binary search tree will not hold same value twice.Suppose we have one tree like this −This tree is one binary search tree. It follows all of the mentioned properties. If we traverse elements into inorder traversal mode, we can get 5, 8, 10, 15, 16, 20, 23. Let us see one code to understand how we can implement this in ... Read More

388 Views
In this section we will see the pre-order traversal technique (recursive) for binary search tree.Suppose we have one tree like this −The traversal sequence will be like: 10, 5, 8, 16, 15, 20, 23AlgorithmpreorderTraverse(root): Begin if root is not empty, then print the value of root preorderTraversal(left of root) preorderTraversal(right of root) end if EndExample Live Demo#include using namespace std; class node{ public: int h_left, h_right, bf, value; node *left, *right; }; class tree{ private: node *get_node(int key); ... Read More

349 Views
In this section we will see the post-order traversal technique (recursive) for binary search tree.Suppose we have one tree like this −The traversal sequence will be like: 8, 5, 15, 23, 20, 16, 10AlgorithmpostorderTraverse(root): Begin if root is not empty, then postorderTraversal(left of root) postorderTraversal(right of root) print the value of root end if EndExample Live Demo#include using namespace std; class node{ public: int h_left, h_right, bf, value; node *left, *right; }; class tree{ private: node *get_node(int key); ... Read More

804 Views
In this section we will see different traversal algorithms to traverse keys present in binary search tree. These traversals are Inorder traversal, Preorder traversal, Postorder traversal and the level order traversal.Suppose we have one tree like this −The Inorder traversal sequence will be like − 5 8 10 15 16 20 23The Preorder traversal sequence will be like − 10 5 8 16 15 20 23The Postorder traversal sequence will be like − 8 5 15 23 20 16 10The Level-order traversal sequence will be like − 10, 5, 16, 8, 15, 20, 23AlgorithminorderTraverse(root): Begin if root is not ... Read More

4K+ Views
In this section we will see some important properties of one binary tree data structure. Suppose we have a binary tree like this.Some properties are −The maximum number of nodes at level ‘l’ will be $2^{l-1}$ . Here level is the number of nodes on path from root to the node, including the root itself. We are considering the level of root is 1.Maximum number of nodes present in binary tree of height h is $2^{h}-1$ . Here height is the max number of nodes on root to leaf path. Here we are considering height of a tree with one ... Read More

14K+ Views
Here we will see how to represent a binary tree in computers memory. There are two different methods for representing. These are using array and using linked list.Suppose we have one tree like this −The array representation stores the tree data by scanning elements using level order fashion. So it stores nodes level by level. If some element is missing, it left blank spaces for it. The representation of the above tree is like below −12345678910111213141510516-81520-------23The index 1 is holding the root, it has two children 5 and 16, they are placed at location 2 and 3. Some children are ... Read More

570 Views
Here we will see some basic operations of array data structure. These operations are −TraverseInsertionDeletionSearchUpdateThe traverse is scanning all elements of an array. The insert operation is adding some elements at given position in an array, delete is deleting element from an array and update the respective positions of other elements after deleting. The searching is to find some element that is present in an array, and update is updating the value of element at given position. Let us see one C++ example code to get better idea.Example Live Demo#include #include using namespace std; main(){ vector arr; //insert elements ... Read More

778 Views
Amortize AnalysisThis analysis is used when the occasional operation is very slow, but most of the operations which are executing very frequently are faster. In Data structures we need amortized analysis for Hash Tables, Disjoint Sets etc.In the Hash-table, the most of the time the searching time complexity is O(1), but sometimes it executes O(n) operations. When we want to search or insert an element in a hash table for most of the cases it is constant time taking the task, but when a collision occurs, it needs O(n) times operations for collision resolution.Aggregate MethodThe aggregate method is used to ... Read More