- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10710 Articles for Web Development

98 Views
ProblemWe are required to write a JavaScript function that takes in a string, str, which contains a combination of alphabets, special characters and numbers.Our function should return a new string based on the input string that contains only the numbers present in the string str, maintaining their relative order.For example, if the input to the function is −const str = 'revd1fdfdfs2v34fd5gfgfd6gffg7ds';Then the output should be −const output = '1234567';ExampleFollowing is the code − Live Democonst str = 'revd1fdfdfs2v34fd5gfgfd6gffg7ds'; const pickNumbers = (str = '') => { let res = ''; for(let i = 0; i < str.length; i++){ ... Read More

102 Views
ProblemWe are required to write a JavaScript function that takes in a lowercase English alphabets string, str, as the first and the only argumentOur function should create and return a new string based on the input string which contains characters sorted according to the reverse English alphabets.For example, if the input to the function is −const str = 'abcdef';Then the output should be −const output = 'fedcba';ExampleFollowing is the code − Live Democonst str = 'abcdef'; const reverseSorting = (str = '') => { const strArr = str.split(''); const mapString = 'abcdefghijkmnopqrstuvwxyz'; const sorter = (a, b) => ... Read More

75 Views
ProblemWe are required to write a JavaScript function that takes in two arrays of numbers, arr1 and arr2, as the first and the second arguments.Our function should merge the elements of both these arrays into a new array and if upon merging or before merging there exists any duplicates, we should delete the excess duplicates so that only one copy of each element is present in the merged array.The order here is not so important but the frequency of elements (which should be 1 for each element) is important.For example, if the input to the function is −onst arr1 = ... Read More

357 Views
ProblemWe are required to write a JavaScript function that takes in a number, num, as the first and the only argument.Our function should return true if the sum of the digits of the number num is a palindrome number, false otherwise.For example, if the input to the function is −const num = 781296;Then the output should be −const output = true;Output ExplanationBecause the digit sum of 781296 is 33 which is a palindrome number.ExampleFollowing is the code − Live Democonst num = 781296; const findSum = (num, sum = 0) => { if(num){ return findSum(Math.floor(num / 10), sum + (num ... Read More

133 Views
ProblemWe are required to write a JavaScript function that takes in an array of numbers, arr, as the first argument and a number, num, (num { const res = []; let sum = 0, right = 0, left = 0; for(; right < num; right++){ sum += arr[right]; }; res.push(sum); while(right < arr.length){ sum -= arr[left]; sum += arr[right]; right++; left++; res.push(sum); }; return res; }; console.log(accumulateArray(arr, num));OutputFollowing is the console output−[3, 5, 7, 9, 11]
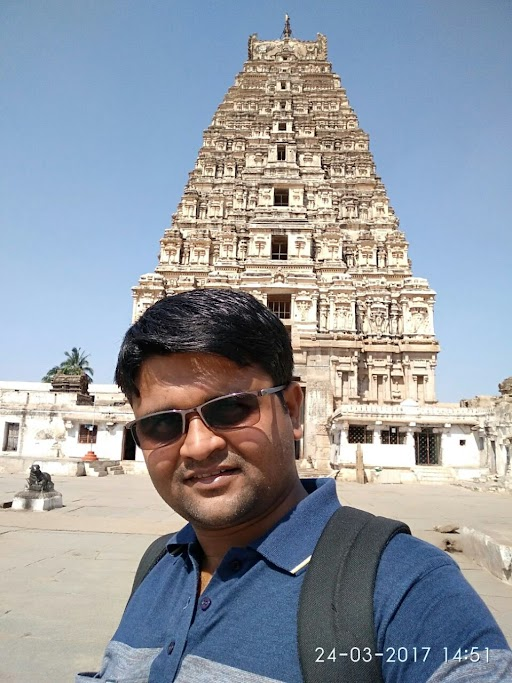
1K+ Views
There are two methods namely blogging and vlogging for sharing your personal experiences or observations with a community. These two methods involve sharing information through internet. The basic difference between a blog and a vlog is in their medium of information sharing, i.e., a blog is a medium in which the information is shared using text, images, etc. in the form a story. On the other hand, vlogging is a form of video-blogging. There is a tremendous increase in popularity of vlog in recent years. The popular platforms for vlogging are YouTube, Instagram, Snapchat, etc. Read through this article to ... Read More

83 Views
ProblemWe are required to write a JavaScript function that takes in two arrays of literals, arr1 and arr2, as the first and the second argument.Our function should find out the common element in arr1 and arr2 with the least list index sum. If there is a choice tie between answers, we should output all of them with no order requirement.For example, if the input to the function is− Live Democonst arr1 = ['a', 'b', 'c', 'd']; const arr2 = ['d', 'a', 'c'];Then the output should be −const output = ['a'];Output ExplanationSince 'd' and 'a' are common in both the arrays the ... Read More

146 Views
ProblemWe are required to write a JavaScript function that takes in a string number as the only argument.Our function should return the input number with the second half of digits changed to 0.In cases where the number has an odd number of digits, the middle digit onwards should be changed to 0.For example −938473 → 938000ExampleFollowing is the code − Live Democonst num = '938473'; const convertHalf = (num = '') => { let i = num.toString(); let j = Math.floor(i.length / 2); if (j * 2 === i.length) { return parseInt(i.slice(0, j) + '0'.repeat(j)); ... Read More

111 Views
ProblemWe are required to write a JavaScript function that takes in a number n. Our function is required to return the maximum value by rearranging its digits.ExampleFollowing is the code −const num = 124; const rotateToMax = n => { n = n .toString() .split('') .map(el => +el); n.sort((a, b) => return b - a; }); return n .join(''); }; console.log(rotateToMax(num));Output421

274 Views
ProblemWe are required to write a JavaScript function that takes in an array of integers. Our function should sum the differences between consecutive pairs in the array in descending order.For example − If the array is −[6, 2, 15]Then the output should be −(15 - 6) + (6 - 2) = 13ExampleFollowing is the code − Live Democonst arr = [6, 2, 15]; const sumDifference = (arr = []) => { const descArr = arr.sort((a, b) => b - a); if (descArr.length