- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10711 Articles for Web Development

95 Views
The JavaScript eval() is used to execute an argument. The code gets execute slower when the eval() method is used. It also has security implementations since it has a different scope of execution.ExampleHere’s how you can implement eval() function − var a = 30; var b = 12; var res1 = eval("a * b") + ""; var res2 = eval("5 + 10") + ""; document.write(res1); document.write(res2);
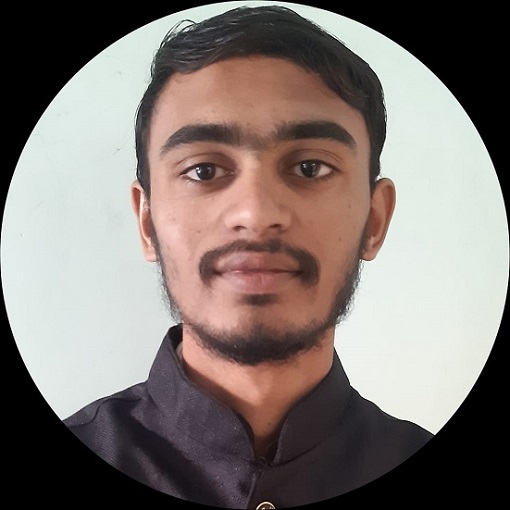
49K+ Views
This tutorial will teach us to call a JavaScript function on page load. In many cases, while programming with HTML and JavaScript, programmers need to call a function, while loading the web page or after the web page load finishes. For example, programmers need to show the welcome message to the user on page load, and the programmer needs to call a JavaScript function after the page load event.There are various ways to call a function on page load or after page load in JavaScript, and we will look at them one by one in this tutorial.Using the onload event ... Read More

7K+ Views
++a returns the value of an after it has been incremented. It is a pre-increment operator since ++ comes before the operand.a++ returns the value of a before incrementing. It is a post-increment operator since ++ comes after the operand.ExampleYou can try to run the following code to learn the difference between i++ and ++i − var a =10; var b =20; //pre-increment operator a = ++a; document.write("++a = "+a); //post-increment operator b = b++; document.write(" b++ = "+b);

134 Views
Using “javascript:void(0)” is definitely better, since its faster. Try to run both the examples in Google Chrome with the developer tools. The “javascript:void(0)” method takes less time than the only #.Here’s the usage of “javascript: void(0)”:If inserting an expression into a web page results in an unwanted effect, then use JavaScript void to remove it. Adding “JavaScript:void(0)”, returns the undefined primitive value.The void operator is used to evaluate the given expression. After that, it returns undefined. It obtains the undefined primitive value, using void(0).The void(0) can be used with hyperlinks to obtain the undefined primitive valueExampleLive Demo ... Read More

123 Views
To split a string on every occurrence of ~, split the array. After splitting, add a line break i.e. for each occurrence of ~.For example, This is demo text 1!~This is demo text 2!~~This is demo text 3!After the split and adding line breaks like the following for ~ occurrence −This is demo text 1! This is demo text 2! This is demo text 3!ExampleLet’s see the complete exampleLive Demo Adding line break var myArray = 'This is demo text 1!~This is demo text 2!~ ... Read More
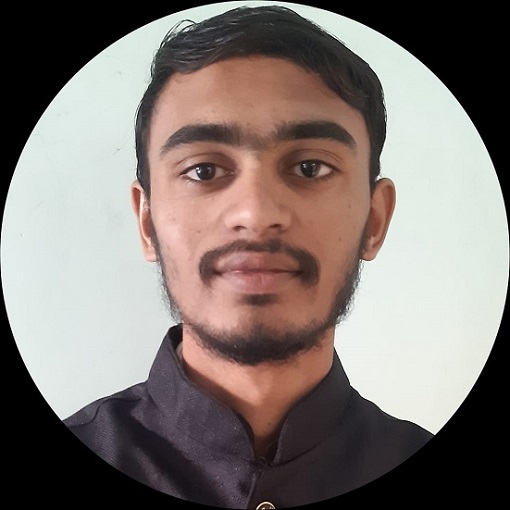
2K+ Views
Calling a function from a string, stored in a variable can be done in two different ways. The first approach makes use of the window object method, and the second method makes use of the eval() function. This tutorial will guide you to learn the way to execute a JavaScript function using its name as a string. Using the window[]() Method Here, to execute the function using the function name as a string, the string should be changed to a pointer using the following syntax. Syntax var functionName = "string"; functionName = window[functionName]( parameters ); Here we have stored ... Read More

262 Views
The JavaScript eval() is used to execute an argument. The code gets execute slower when the eval() method is used. It also has security implementations since it has a different scope of execution. In addition, use it to evaluate a string as a JavaScript expression.The eval() method is not suggested to use in JavaScript since it executes slower and improper usage opens your website to injection attacks.ExampleHere’s how you can implement eval() functionLive Demo var a = 30; var b = 12; var res1 = eval("a * b") + ""; var res2 = eval("5 + 10") + ""; document.write(res1); document.write(res2);

1K+ Views
The (function() { } )() construct is an immediately invoked function expression (IIFE). It is a function, which executes on creation.SyntaxHere’s the syntax −(function() { // code })();As you can see above, the following pair of parentheses converts the code inside the parentheses into an expression −function(){...}In addition, the next pair, i.e. the second pair of parentheses continues the operation. It calls the function, which resulted from the expression above.
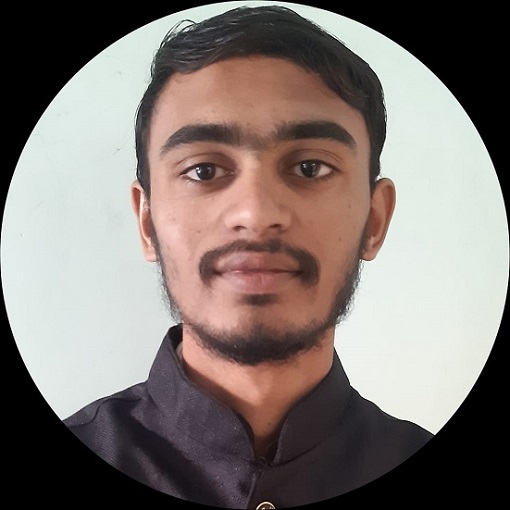
13K+ Views
In this tutorial, we will learn to check whether the JavaScript function is defined or not. If a programmer calls the JavaScript function without defining it, they will see the reference error with the message like “function is not defined.” To overcome the problem, the programmer can check whether the function is defined and call the function. We will look at the various approaches to check if the function is defined or not in JavaScript below. Using the typeof Operator In JavaScript, the typeof operator is useful to check the type of the variable, function, objects, etc. When we use ... Read More

190 Views
The function* declaration is used to define a generator function. It returns a Generator object. Generator Functions allows execution of code in between when a function is exited and resumed later. So, generators can be used to manage flow control in a code.SyntaxHere’s the syntax −function *myFunction() {} // or function* myFunction() {} // or function*myFunction() {}Let’s see how to use generator functionExampleLive Demo function* display() { var num = 1; while (num < 5) ... Read More