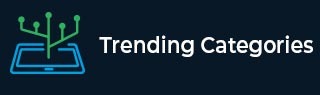
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming
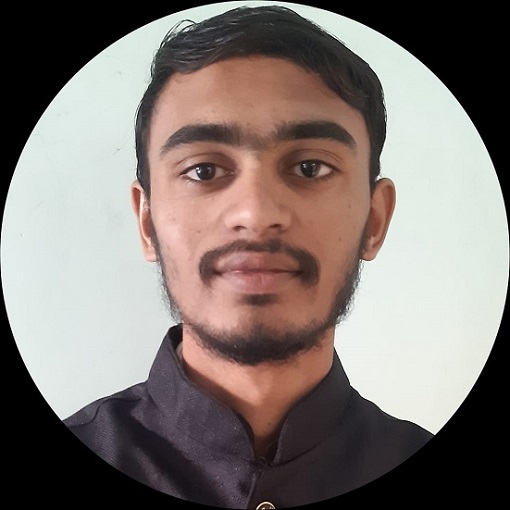
158 Views
In this problem, we need to ensure whether the string String2 contains string String1 as a substring. We will first see the naïve approach to solving the problem, finding all substrings of String1’s length in String2 with String1. Furthermore, we will use built−in methods like match(), includes(), and indexOf() methods to find the String1 substring in the String2. Problem statement − We have given a string String1 and String2 of different sizes. We need to check whether string String1 is present in string String2 as a substring. Sample examples Input string1 = "tutor"; string2 = "pointtutorial"; Output ... Read More

462 Views
Graphical User Interface (GUI) frameworks provide developers with the tools and capabilities to create visually appealing and interactive applications. PyQt5, a Python binding for the Qt framework, offers a robust toolkit for building GUI applications with ease. Among the fundamental components offered by PyQt5 is the CheckBox, a widget that allows users to select or deselect an option. By adding actions to CheckBoxes, we can enhance the functionality and interactivity of our applications. This feature enables us to perform specific tasks or trigger events based on the state of the CheckBox. Whether it is enabling or disabling a feature, updating ... Read More
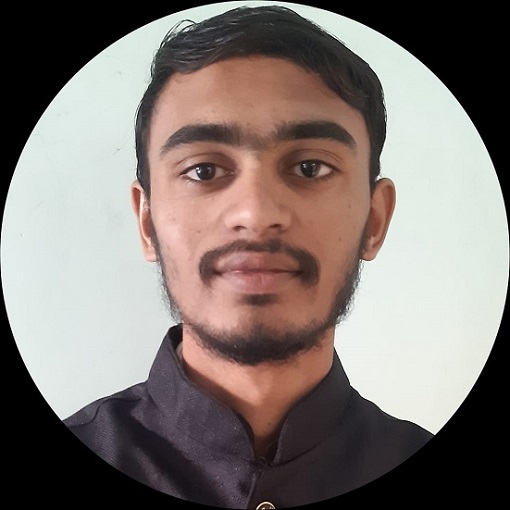
363 Views
We need to remove all adjacent duplicate characters from the string in this problem. We can use a recursive or iterative approach to solve the problem. Here, we first need to remove the adjacent duplicate elements from the left part of the string. After that, we need to recursively remove the adjacent duplicates from the right part of the string. Problem statement − We have given string str of length N containing alphanumeric characters. We need to recursively remove all adjacent duplicate characters so that the resultant string does not contain any adjacent duplicate characters. Sample examples Input str1 ... Read More
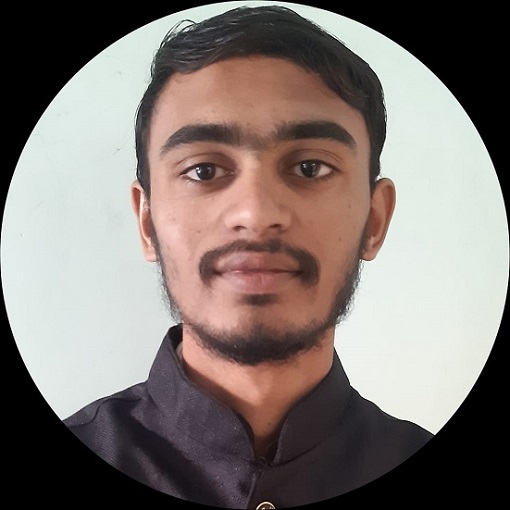
151 Views
In this tutorial, we need to write a Java program to count the total number of minimum required rotations to get the original string. We can solve the problem by getting the rotations substrings of the original string or concatenating the original string to itself. Problem statement − We have given string str of length N. The task is to find the total number of minimum rotations we need to perform to get the original string. Sample examples Input str = "bcdbcd"; Output 3 Explanation − We need to make 3 rotations to get the ... Read More
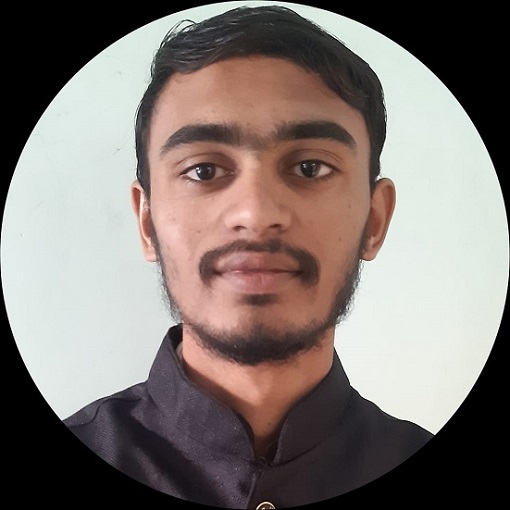
97 Views
In this problem, we have given n strings and need to make all strings equal by rotating them. To solve the problem, we need to pick any string from the array and need make all strings equal by rotating them. Also, we need to count the total number of required operations to rotate all strings. We can pick each string one by one, make all other strings equal, count the total operations for each string, and take a minimum of them. Problem statement − We have given an array containing n strings. All strings are permutations of each other. ... Read More
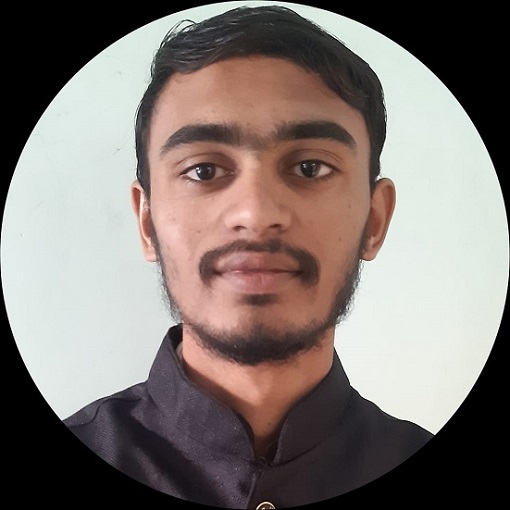
103 Views
In this problem, we need to add spaces before the given indexes in the string. We can use two different approaches to solve the problem. The first approach moves the characters of the given string to add space at a particular index, and the second approach replaces the characters of the pre−initialize string with spaces. Problem statement − We have given string str of length N containing the alphanumeric characters. Also, we have given the spaceList array containing M positive integers representing the string index. We need to app space to the string before each index that exists in the ... Read More
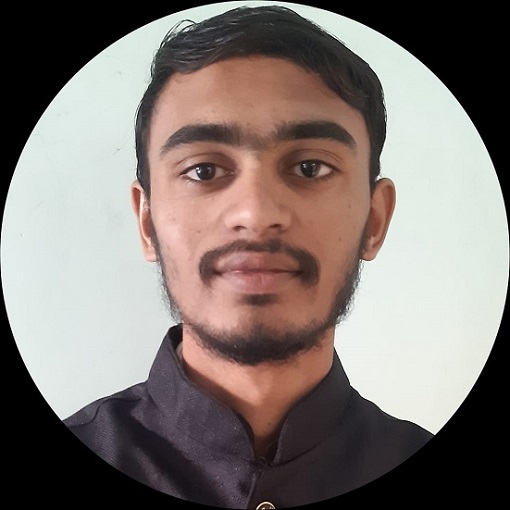
417 Views
We need to find all palindrome strings from the given array in this problem. The string is a palindrome if we can read it the same from the start and end. We can use two approaches to check whether the string is a palindrome. In the first approach, we reverse the string and compare it with the original string, and in the second approach, we keep comparing the string characters from start to last. Problem statement − We have given an array containing the N strings. We need to print all the strings of the array, which are palindrome. If ... Read More
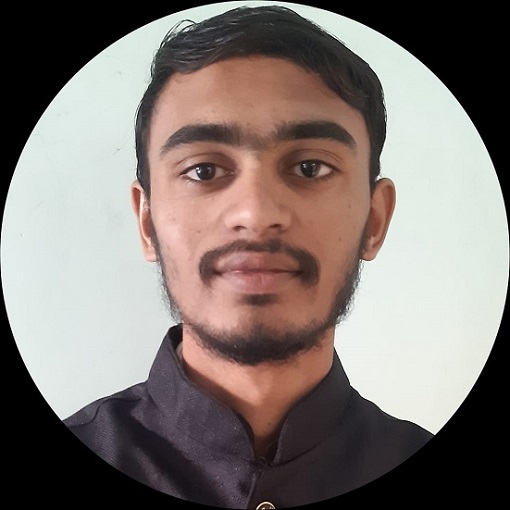
159 Views
In this problem, we need to decode the given string by repeatedly adding the total count number of times. We can have three different approaches to solving the problem, and we can use two stacks or one stack to solve the problem. Also, we can solve the problem without using the two stacks. Problem statement − We have given a string str containing the opening and closing brackets, alphabetical and numeric characters. We need to decode the string recursively. Here are the patterns or rules to decode the string. [chars] − The ‘chars’ should appear count times in ... Read More
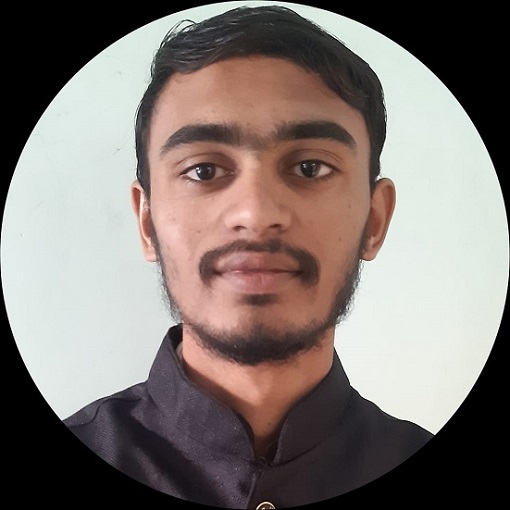
98 Views
In this problem, we need to count the total vowels and constants in the given linked list. We can traverse the linked list and check for each character, whether it is a constant or vowel. Problem statement − We have given a linked list containing the lowercase alphabetical characters. We need to count the total number of vowels and constants in the linked list. Sample examples Input 'a' -> 'b' -> 'e', 'c' -> 'd' -> 'e' -> 'f'’ -> 'o' -> 'i' -> 'a' -> 'a' Output Vowel – 7, constant - 4 Explanation − ... Read More
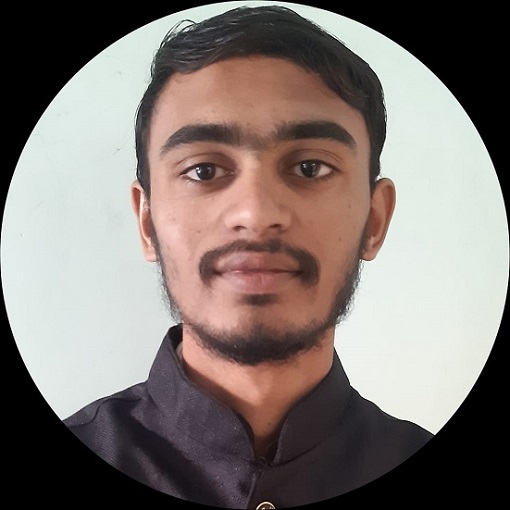
40 Views
In this problem, we need to perform the given operations with each string prefix. In the end, we need to count the frequency of each character. We can follow the greedy approach to solve the problem. We need to take each prefix of length K and update its characters according to the given conditions. We can use the map to count the frequency of characters in the final string. Problem statement − We have given the strings tr containing the N lowercase alphabetical characters. Also, we have given the mapping list, which contains total 26 elements. Each element is mapped ... Read More