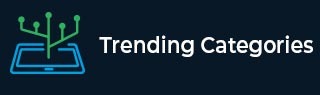
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

2K+ Views
In the world of Python programming, developers often encounter situations where they need to apply a function to every element of a list or an iterable. Traditionally, this involves writing a loop to iterate through the elements and apply the function one by one. However, Python provides a concise and powerful tool called lambda functions, also known as anonymous functions, which allow us to perform iterative operations without the need for explicit loops. In this blog post, we will explore the concept of iterating with Python lambda and discover its usefulness in various scenarios. Understanding Lambda Functions Before diving into ... Read More

975 Views
Inplace editing is a technique that allows us to modify the contents of a file directly, without the need for creating a new file or loading the entire file into memory. It offers a streamlined approach to file manipulation by allowing us to make changes directly to the existing file, making it an efficient and resource-friendly method. To facilitate inplace editing in Python, the fileinput module comes into play. The fileinput module, part of the Python standard library, provides a high-level interface for reading and writing files, streamlining the process of inplace editing. With the fileinput module, we can open ... Read More

1K+ Views
Index-based operations play a vital role in manipulating and accessing specific elements or subsets of data within tensors. PyTorch, a popular open-source deep learning framework, provides powerful mechanisms to perform such operations efficiently. By leveraging index-based operations, developers can extract, modify, and rearrange data along various dimensions of a tensor. Tensor Basics PyTorch tensors are multi-dimensional arrays that can hold numerical data of various types, such as floating-point numbers, integers, or Boolean values. Tensors are the fundamental data structure in PyTorch and serve as the building blocks for constructing and manipulating neural networks. To create a tensor in PyTorch, we ... Read More

10K+ Views
In object-oriented programming, inheritance allows you to create new classes based on existing ones, providing a way to reuse code and organize your program's structure. Python, being an object-oriented language, supports inheritance and allows you to override methods defined in parent classes in child classes. However, there may be situations where you want to leverage the functionality of a parent class method while extending or modifying it in the child class. Method Overriding in Python Before we learn about calling parent class methods, let's briefly discuss method overriding. Method overriding is a feature in object-oriented programming that allows a ... Read More

747 Views
When working with file systems and directories in Python, it's often useful to be able to identify and handle empty directories. Empty directories can accumulate over time, taking up unnecessary space or cluttering the directory structure. Being able to programmatically find and handle these empty directories can help streamline file system operations and improve overall organization. In this tutorial, we will explore different methods to obtain a list of all empty directories using Python. We will cover two approaches: the first using the os.walk() function, and the second utilizing the os.scandir() function. Both methods are efficient and provide different benefits ... Read More

119 Views
Computer vision tasks often require preprocessing and augmentation of image data to improve model performance and generalization. PyTorch, a popular deep learning framework, provides a powerful library for image transformations called torchvision.transforms. This library offers a wide range of predefined transforms for data augmentation and preprocessing. However, in some cases, predefined transforms may not be sufficient, and we need to apply custom transformations to our image data. In this blog post, we will explore the concept of functional transforms in PyTorch and demonstrate how to create and apply custom transforms for computer vision tasks. Understanding Transforms in PyTorch Transforms in ... Read More

182 Views
String manipulation and analysis are fundamental tasks in many programming scenarios. One intriguing problem within this domain is the task of finding the frequency of all substrings within a given string. This article aims to provide a comprehensive guide on efficiently accomplishing this task using the powerful Python programming language. When working with strings, it is often necessary to analyze their contents and extract valuable information. The frequency of substrings is an important metric that can reveal patterns, repetitions, or insights into the structure of the string. By determining how many times each substring appears in a given string, we ... Read More
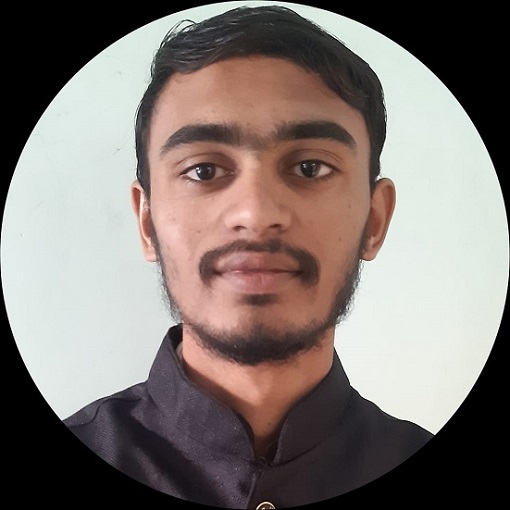
345 Views
In this problem, we need to convert one binary string to another binary string by flipping the characters of the string. We can hold any set bit and flip other bits, and we need to count the total operation to achieve another string by doing it. We can solve the problem based on the total number of ‘01’ and ‘10’ pairs in the given strings. Problem statement − We have given two strings named str1 and str2 of the same length containing ‘0’ and ‘1’ characters, representing the binary string. We need to convert the string str1 to str2 by ... Read More

83 Views
In the world of natural language processing (NLP) and text manipulation, finding all possible space joins in a string can be a valuable task. Whether you're generating permutations, exploring word combinations, or analyzing text data, being able to efficiently discover all the potential ways to join words using spaces is essential. Through this process, we'll generate all possible combinations, enabling us to explore numerous word arrangements and gain valuable insights from our text data. Problem Statement Given a string of words, we want to generate all possible combinations by inserting spaces between the words. string = "hello world". To further ... Read More
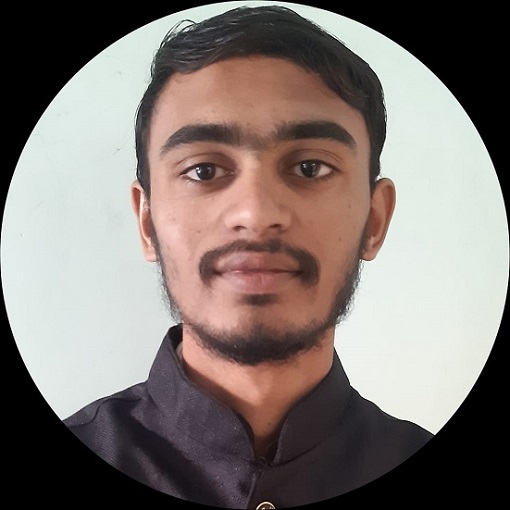
64 Views
In this problem, we need to find the reversible equal substring of maximum length from the start and end of the string. The problem is very similar to finding the palindromic string. We can start traversing the string and traverse the string until characters from the start and end match. Problem statement − We have given string str containing N characters. We need to check whether the string contains the reversible equal substring at the start and end of the string. If we find the substring according to the given condition, print the longest substring. Otherwise, print ‘false’ in the ... Read More